JQuery
大纲
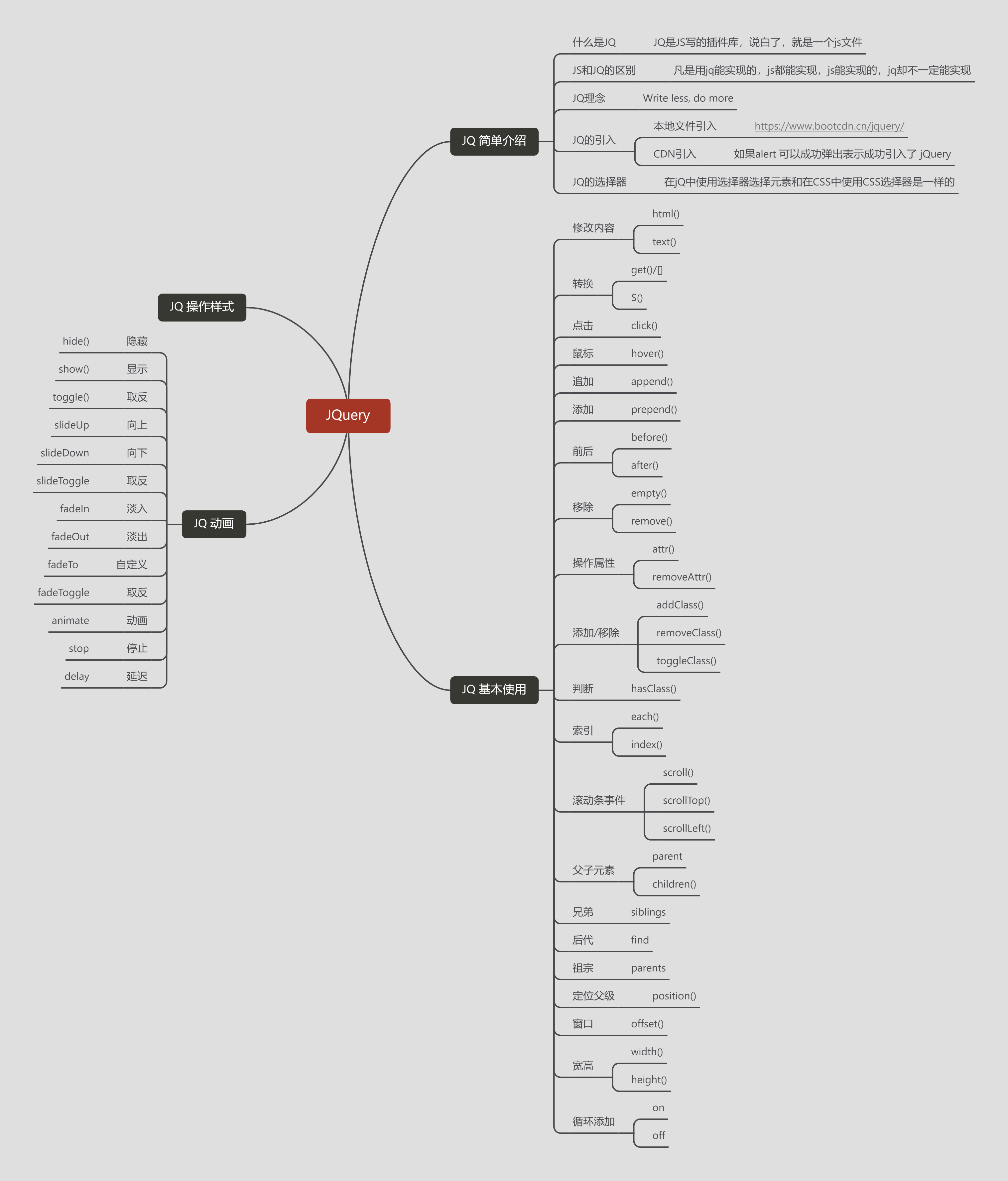
JQ的引入
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title> JQuery的引入 </title>
</head>
<body>
<div id="box">我是女王</div>
<!--方法一: CDN引入JQuery-->
<!-- <script src="https://cdn.bootcdn.net/ajax/libs/jquery/3.6.0/jquery.js"></script>-->
<!--方法二:本地文件引入JQuery-->
<script src="JQuery.js"></script>
<!--如果网页内容改变,则表示成功引入了 jQuery-->
<script>
var $box = $("#box").html("hello world");
</script>
</body>
</html>
JS和JQ的用法
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title> JS和JQ的用法 </title>
</head>
<body>
<div id="box">我是女王</div>
<ul>
<li>1</li>
<li>2</li>
<li>3</li>
<li>4</li>
</ul>
<!-- 引入JQ文件-->
<script src="JQuery.js"></script>
<script>
// js获取对象
var box = document.getElementById("box");
box.innerText = "hello world"
// jq获取对象
// 方法一
$(box).html("how are you!")
// 方法二
$("#box").html("I love you")
// jq获取多个
// 方法一:通过索引获取
var $li = $("ul li");
$li[1].innerText = "白雪";
// 方法二:通过get获取
$li.get(2).innerText = "皇后";
// 方法三:通过eq获取 方法返回带有被选元素的指定索引的元素
$li.eq(3).text("皇子");
// 把所有li标签都获取并修改
$li.text("beauti")
</script>
</body>
</html>
each方法
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title> each方法 </title>
</head>
<body>
<ul>
<li>1</li>
<li>2</li>
<li>3</li>
<li>4</li>
</ul>
<!-- 引入JQ文件-->
<script src="JQuery.js"></script>
<script>
// each 方法只适用于有下标,内容的,如果要去执行事件的话,就不太合适了
// each方法获取标签 相当于一个 for 循环 遍历对象
$("ul li").each(function (i) {
// this 表示当前的对象 $("ul li")
$(this).html("我是第"+i+"个")
})
// each方法 遍历数组 each(obj,callback) obj 数组 callback 要执行的函数
var $obj = [52,65,89];
$(function () {
$.each($obj,function (index,value) {
alert(index+":"+value);
})
})
</script>
</body>
</html>
操作方法
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title> 操作方法 </title>
</head>
<body>
<div id="box">
<p class="box">1</p>
<p>2</p>
<p class="wrap">3</p>
</div>
<ul>
<li>1</li>
<li>2</li>
<li>3</li>
<li>4</li>
</ul>
<!-- 引入JQ文件-->
<script src="JQuery.js"></script>
<script>
// attr 操作属性
var $box = $("#box");
alert($box.attr("id")); // 获取id属性的值 // box
$box.attr("class","add"); // 添加属性 // 新增了属性 class="add"
// removeAttr 移除属性
$box.removeAttr("class"); //移除了属性 class="add"
// addClass 添加类属性
$("p").eq(0).addClass("xiongkong"); // class="box xiongkong"
// removeClass 删除类属性
$("p").eq(0).removeClass("box"); // class="xiongkong"
// toggleClass 有就删除,没有就添加
$("p").toggleClass("mcdog"); // 所有p标签都添加了属性 class="mcdog"
// parent 找父类
console.log($("li").parent()); // ul
// children 找子类
console.log($("ul").children()); // li, li, li, li
// siblings 兄弟标签
console.log($("ul").siblings()); // div#box, script, script
// find 找后代,但是要指定标签名,否则无法找到
console.log($("ul").find("li")); // li, li, li, li
// hasClass 查看是否有属性
console.log($("p").eq(0).hasClass("box")); // false
</script>
</body>
</html>
操作样式
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title> 操作样式 </title>
<style>
*{
margin: 0;
padding: 0;
}
#box{
/**/
width: 100px;
height: 100px;
background-color: red;
/*外边距 上下50px 左右0*/
margin: 50px;
/*边框*/
border: 10px solid skyblue;
/*内边距*/
padding: 40px;
/*相对定位 父代 父相子绝*/
position: relative;
}
#wrap {
width: 50px;
height: 50px;
background-color: springgreen;
/*绝对定位 子代*/
position: absolute;
/*上边距*/
top: 100px;
/*左边距*/
left:100px;
}
</style>
</head>
<body>
<div id="box">
<div id="wrap"></div>
</div>
<!-- 引入JQ文件-->
<script src="JQuery.js"></script>
<script>
// 打印宽度
// innerWidth 打印的是内容+内边距
console.log($("#box").width()); // 100
console.log($("#box").innerWidth()); // 100+40+40=180
// outerWidth 打印的是内容+内边距+边框
console.log($("#box").outerWidth()); // 100+40+40+10+10=200
// 修改标签样式
$("#box").css({
"width":"200px",
"height":"200px",
"background":"pink"
})
// 案例
// 点击页面任意位置,都会触发点击事件
$(document).click(function () {
$("#box").css({
"width":"200px",
"height":"200px",
"background":"pink"
})
})
// position 到父级padding的距离
console.log($("#wrap").position().left); // 100
// offset 到网页边距的距离
console.log($("#box").offset().left); // 50
</script>
</body>
</html>
事件
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title> 事件 </title>
<style>
*{
margin: 0;
padding: 0;
}
li{
width: 50px;
height: 50px;
background-color: pink;
margin: 10px;
/*去除无序列表前的点*/
list-style: none;
/*居中*/
text-align: center;
line-height: 50px;
/*字体颜色*/
color: white;
/*圆角*/
border-radius: 5px;
}
ul{
/*边框*/
border: 5px solid skyblue;
/*圆角*/
border-radius: 5px;
margin: 10px;
}
</style>
</head>
<body>
<ul id="box">
<li>1</li>
<li>2</li>
<li>3</li>
<li>4</li>
</ul>
<!-- 引入JQ文件-->
<script src="JQuery.js"></script>
<script>
var $box = $("#box");
// 事件
// on() 循环添加事件
$box.on({
// 鼠标点击事件
"click":function () {
console.log("click");
},
// 鼠标双击事件
"dblclick":function () {
console.log("dblclick")
},
// 鼠标划入事件
"mouseenter":function () {
console.log("mouseenter");
},
// 鼠标划出事件
"mouseleave":function () {
console.log("mouseleave");
}
})
// 鼠标事件 划入划出
$box.hover(function () {
console.log("我进来了")
},function () {
console.log("我出去了")
})
</script>
</body>
</html>
动画
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title> 动画 </title>
<style>
#box{
width: 200px;
height: 200px;
background-color: pink;
}
</style>
</head>
<body>
<div id="box"></div>
<script src="JQuery.js"></script>
<script>
$(document).click(function () {
// toggle 取反 效果:往左上角消失或重现
// $("#box").toggle(3000);
// fadeTo 透明度 透明度是0-1
// $("#box").fadeTo(3000,0.1);
// fadeToggle 透明度取反 效果:消失或重现
// $("#box").fadeToggle(3000);
// slideToggle 可做进度条 效果:向下拉伸
// $("#box").slideToggle(3000);
// echarts 图形网站
// tootstrap 美观前端网站
})
// 案例
var off = true;
$(document).click(function () {
if (off){
$("#box").fadeTo(3000,0.1);
}else {
$("#box").fadeTo(3000,1);
}
// 非 变成false
off = !off;
})
</script>
</body>
</html>
案例
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title> 动画案例 </title>
<style>
.box{
width: 200px;
height: 200px;
background-color: skyblue;
}
</style>
</head>
<body>
<input type="button" value="动画" id="btn">
<div class="box"></div>
<script src="JQuery.js"></script>
<script>
$(function () {
var $btn = $("#btn");
var $div = $(".box");
// animate 可以嵌套多个动画
$btn.click(function () {
$div.animate({
// 宽度
"width":"1000"
},1500,function () {
$div.animate({
// 上边距
"margin-top":"300"
},1500,function () {
$div.animate({
// 宽度跟上边距
"width":"200",
"margin-top":0
},1500)
})
})
})
})
</script>
</body>
</html>