LeetCode-13. Roman to Integer | 罗马数字转整数
题目
题解
先维护一个map
,用于建立罗马数字和整数的关系,接着挨个破译即可。
//Go
func romanToInt(s string) int {
var charToIntMap = make(map[byte]int, 8)
charToIntMap['I'] = 1
charToIntMap['V'] = 5
charToIntMap['X'] = 10
charToIntMap['L'] = 50
charToIntMap['C'] = 100
charToIntMap['D'] = 500
charToIntMap['M'] = 1000
sum := 0
for i:= 0; i < len(s); i++ {
switch s[i] {
case 'I':
if i + 1 < len(s) && (s[i+1] == 'V' || s[i+1] == 'X') {
sum -= charToIntMap[s[i]]
} else {
sum += charToIntMap[s[i]]
}
break
case 'X':
if i + 1 < len(s) && (s[i+1] == 'L' || s[i+1] == 'C') {
sum -= charToIntMap[s[i]]
} else {
sum += charToIntMap[s[i]]
}
break
case 'C':
if i + 1 < len(s) && (s[i+1] == 'D' || s[i+1] == 'M') {
sum -= charToIntMap[s[i]]
} else {
sum += charToIntMap[s[i]]
}
break
default:
sum += charToIntMap[s[i]]
}
}
return sum
}
执行结果:
leetcode-cn执行:
执行用时:16 ms, 在所有 Go 提交中击败了22.55%的用户
内存消耗:3.1 MB, 在所有 Go 提交中击败了78.12%的用户
leetcode执行:
Runtime: 8 ms, faster than 64.36% of Go online submissions for Roman to Integer.
Memory Usage: 3.1 MB, less than 100.00% of Go online submissions for Roman to Integer.
版权声明:本文版权归作者和博客园共有,欢迎转载,但未经作者同意必须保留此段声明。
特此声明:所有评论和私信都会在第一时间回复。也欢迎园子里和园子外的大大们指正错误,共同进步。或者直接私信我 (^∀^)
声援博主:如果您觉得文章对您有帮助,可以点击文章右下角【推荐】一下。您的鼓励是作者坚持原创和持续写作的最大动力!
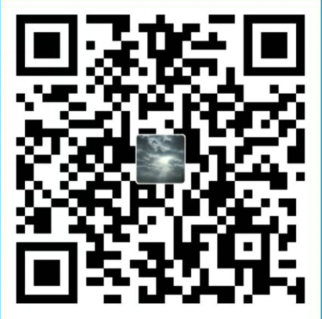
您的资助是我最大的动力!
金额随意,欢迎来赏!
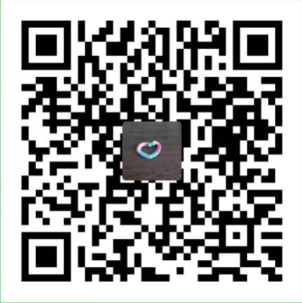
如果,您希望更容易地发现我的新博客,不妨点击一下绿色通道的
本博客的所有打赏均将用于博主女朋友的化妆品购买以及养肥计划O(∩_∩)O。我是【~不会飞的章鱼~】!