C#数组学习
1.多维数组
using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace ControlProject { class Program { static void Main(string[] args) { //新建一个3*4的数组 string[,] person = new string[3, 4]; person[0, 0] = "姓名"; person[0, 1] = "性别"; person[0, 2] = "职业"; person[0, 3] = "年龄"; person[1, 0] = "神秘"; person[1, 1] = "帅哥"; person[1, 2] = "农民工"; person[1, 3] = "26岁"; person[2, 0] = "小白"; person[2, 1] = "男"; person[2, 2] = "大宅男"; person[2, 3] = "27岁"; StringBuilder sb = new StringBuilder();//用一个可变字符串对象来接收循环中的值 //取出数组二维数组的长度 int x = person.GetLength(0); int y = person.GetLength(1); for (int i = 0; i < x; i++) { for (int j = 0; j < y; j++) { sb.Append(person[i, j] + " | "); } sb.Append("\n----------------------------------------------\n"); } Console.WriteLine(sb.ToString()); } } }
2.锯齿数组
在锯齿数组中,每一行都可以有不同的大小。
锯齿数组的效率比多维数组要高很多。
using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace ControlProject { class Program { static void Main(string[] args) { int[][] myint = new int[3][]; myint[0] = new int[3] { 1, 2, 3 }; myint[1] = new int[2] { 1, 2 }; myint[2] = new int[3] { 1, 2, 3 }; foreach (int[] i in myint) { foreach (int x in i) { Console.Write(x + ","); } Console.WriteLine(); } } } }
3.Array类
数组类型是从抽象基类型Array派生的引用类型,
由于此类型实现了IEnumerable和IEnumerable<(Of<(T>)>), 因此可以对C#中的所有数组使用foreach迭代
4.ArrayList
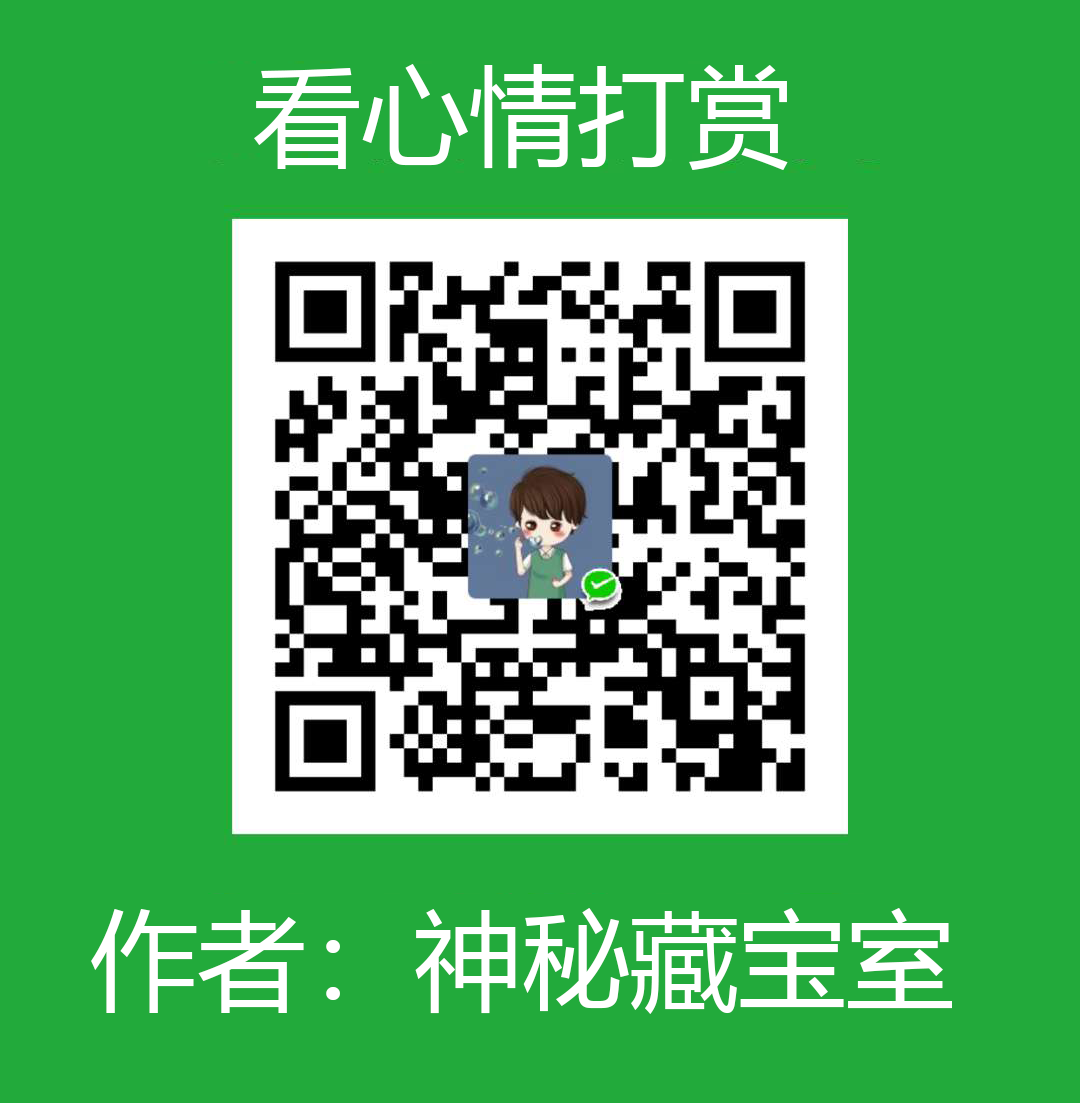
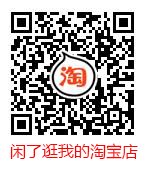
文章对您有帮助,开心可以打赏我,金额随意,欢迎来赏!
需要电子方面开发板/传感器/模块等硬件可以到我的淘宝店逛逛