【luogu P4180 严格次小生成树[BJWC2010]】 模板
题目链接:https://www.luogu.org/problemnew/show/P4180
这个题卡树剖。记得开O2。
这个题inf要到1e18。
定理:次小生成树和最小生成树差距只有在一条边上
非严格次小生成树:枚举每一条不在最小生成树上的边,加入到最小生成树中构成一个环。删去这个环上的最大值。(此最大值有可能与加入生成树中的边相等,故为非严格次小生成树。)重复此操作取min,得到次小生成树。(基于kruskal实现。)
严格次小生成树:与非严格次小生成树类似,不同在于为了避免删去环上的最大值等于加入生成树中的边,需要记录次最大值。恶心点所在。
于是维护最大值和次小值又成了一道数据结构题。
树剖:剖MST,查询加进来的边的两端点编号 = =
#include <cstdio>
#include <cstring>
#include <iostream>
#include <algorithm>
#define ll long long
#define lson l, mid, rt<<1
#define rson mid+1, r, rt<<1|1
using namespace std;
const int maxn = 300010;
const ll inf = 1e18;
inline ll read()
{
char ch = getchar(); ll u = 0, f = 1;
while (!isdigit(ch)) {if (ch == '-')f = -1; ch = getchar();}
while (isdigit(ch)) {u = u * 10 + ch - 48; ch = getchar();}return u * f;
}
ll n, m, fa[maxn], deep[maxn], size[maxn], son[maxn], top[maxn], seg[maxn], rev[maxn], W[maxn], ans = inf, num;
ll father[maxn], mstans, mstcnt;
bool vis[maxn];
struct EDG{
ll u, v, w;
}G[maxn];//mst
struct edge{
ll to, next, len;
}e[maxn<<2];
ll head[maxn], cnt;
void add(ll u, ll v, ll w)
{
e[++cnt].len = w; e[cnt].next = head[u]; e[cnt].to = v; head[u] = cnt;
e[++cnt].len = w; e[cnt].next = head[v]; e[cnt].to = u; head[v] = cnt;
}
//kruskal
bool cmp(EDG a, EDG b)
{
return a.w < b.w;
}
ll find(ll x)
{
return father[x] == x ? x : father[x] = find(father[x]);
}
void init()
{
for(ll i = 1; i <= n; i++) father[i] = i;
sort(G+1, G+1+m, cmp);
}
void kruskal()
{
init();
for(ll i = 1; i <= m; i++)
{
if(mstcnt == n-1) break;
ll x = find(G[i].u), y = find(G[i].v);
if(x != y)
{
mstans += G[i].w;
mstcnt++;
vis[i] = 1;
add(G[i].u, G[i].v, G[i].w);
father[x] = y;
}
}
}
//Segment_Tree
bool maxcmp(ll a, ll b)
{
return a > b;
}
ll get_sec(ll a, ll b, ll c, ll d)
{
ll z[5] = {a, b, c, d};
sort(z, z+4, maxcmp);
for(ll i = 1; i <= 3; i++)
{
if(z[i] != z[0]) return z[i];
}
}
struct Segment_Tree{
ll fir, sec;
}tree[maxn<<2];
void PushUPfir(ll rt)
{
tree[rt].fir = max(tree[rt<<1].fir, tree[rt<<1|1].fir);
}
void PushUPsec(ll rt)
{
tree[rt].sec = get_sec(tree[rt<<1].fir, tree[rt<<1|1].fir, tree[rt<<1].sec, tree[rt<<1|1].sec);
}
void build(ll l, ll r, ll rt)
{
if(l == r)
{
tree[rt].fir = rev[l];
return;
}
ll mid = (l + r) >> 1;
build(lson);
build(rson);
PushUPfir(rt);
PushUPsec(rt);
}
Segment_Tree query(ll left, ll right, ll l, ll r, ll rt)
{
Segment_Tree max1 = {-inf,-inf}, max2 = {-inf,-inf};
if(left <= l && r <= right)
{
return (Segment_Tree){tree[rt].fir, tree[rt].sec};
}
ll mid = (l + r) >> 1;
if(left <= mid) max1 = query(left, right, lson);
if(right > mid) max2 = query(left, right, rson);
return (Segment_Tree) {max(max1.fir, max2.fir), get_sec(max1.fir, max1.sec, max2.fir, max2.sec)};
}
//Tree_cut
void dfs1(ll u, ll f, ll d)
{
ll maxson = -1;
size[u] = 1;
deep[u] = d;
fa[u] = f;
for(ll i = head[u]; i != -1; i = e[i].next)
{
ll v = e[i].to;
if(f != v)
{
W[v] = W[u] + e[i].len;
dfs1(v, u, d+1);
size[u] += size[v];
if(maxson < size[v]) maxson = size[v], son[u] = v;
}
}
}
void dfs2(ll u, ll t)
{
seg[u] = ++num;
rev[num] = W[u] - W[fa[u]];//前缀和边权上点权
//rev[num] = node[u];
top[u] = t;
if(!son[u]) return;
dfs2(son[u], t);
for(ll i = head[u]; i != -1; i = e[i].next)
{
ll v = e[i].to;
if(v == fa[u] || v == son[u]) continue;
dfs2(v, v);
}
}
ll LCA(ll x, ll y, ll d)//当前边的权值
{
ll res = -inf;
while(top[x] != top[y])
{
if(deep[top[x]] < deep[top[y]]) swap(x, y);
Segment_Tree temp1 = query(seg[top[x]], seg[x], 1, n, 1);
x = fa[top[x]];
res = max(res, (temp1.fir == d) ? temp1.sec : temp1.fir);
}
if(deep[x] < deep[y]) swap(x, y);
Segment_Tree temp2 = query(seg[y] + 1, seg[x], 1, n, 1);
return res = max(res, (temp2.fir == d) ? temp2.sec : temp2.fir);
}
int main()
{
memset(head, -1, sizeof(head));
n = read(); m = read(); //scanf("%lld%lld",&n,&m);
for(ll i = 1; i <= m; i++) {G[i].u = read(); G[i].v = read(); G[i].w = read();}//scanf("%lld%lld%lld",&G[i].u,&G[i].v,&G[i].w);
kruskal();
dfs1(1, 0, 1); dfs2(1, 1);
build(1, n, 1);
for(ll i = 1; i <= m; i++)
{
if(vis[i]) continue;
ll temp = mstans + G[i].w - LCA(G[i].u, G[i].v, G[i].w);
if(ans > temp && temp != mstans + G[i].w && temp > mstans) ans = temp;
}
printf("%lld",ans);
return 0;
}
隐约雷鸣,阴霾天空,但盼风雨来,能留你在此。
隐约雷鸣,阴霾天空,即使天无雨,我亦留此地。
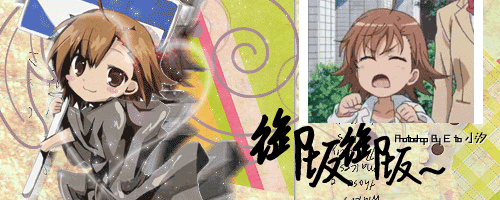