hdu 1198 Farm Irrigation(15ms)
Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 65536/32768 K (Java/Others)
Total Submission(s): 6608 Accepted Submission(s): 2848
Problem Description
Benny has a spacious farm land to irrigate. The farm land is a rectangle, and is divided into a lot of samll squares. Water pipes are placed in these squares. Different square has a different type of pipe. There are 11 types of pipes, which is marked from A to K, as Figure 1 shows.
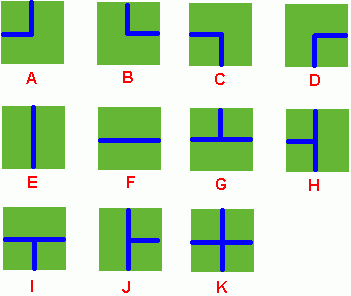
Figure 1
Benny has a map of his farm, which is an array of marks denoting the distribution of water pipes over the whole farm. For example, if he has a map
ADC
FJK
IHE
then the water pipes are distributed like
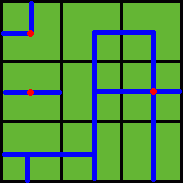
Figure 2
Several wellsprings are found in the center of some squares, so water can flow along the pipes from one square to another. If water flow crosses one square, the whole farm land in this square is irrigated and will have a good harvest in autumn.
Now Benny wants to know at least how many wellsprings should be found to have the whole farm land irrigated. Can you help him?
Note: In the above example, at least 3 wellsprings are needed, as those red points in Figure 2 show.
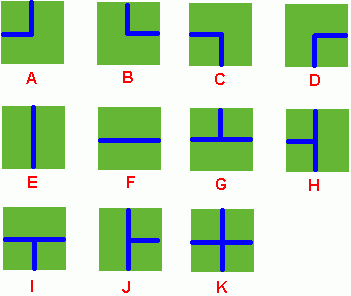
Benny has a map of his farm, which is an array of marks denoting the distribution of water pipes over the whole farm. For example, if he has a map
ADC
FJK
IHE
then the water pipes are distributed like
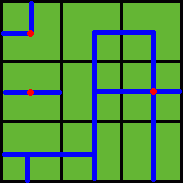
Several wellsprings are found in the center of some squares, so water can flow along the pipes from one square to another. If water flow crosses one square, the whole farm land in this square is irrigated and will have a good harvest in autumn.
Now Benny wants to know at least how many wellsprings should be found to have the whole farm land irrigated. Can you help him?
Note: In the above example, at least 3 wellsprings are needed, as those red points in Figure 2 show.
Input
There are several test cases! In each test case, the first line contains 2 integers M and N, then M lines follow. In each of these lines, there are N characters, in the range of 'A' to 'K', denoting the type of water pipe over the corresponding square. A negative M or N denotes the end of input, else you can assume 1 <= M, N <= 50.
Output
For each test case, output in one line the least number of wellsprings needed.
Sample Input
2 2
DK
HF
3 3
ADC
FJK
IHE
-1 -1
Sample Output
2
3
题目大意:农民有块矩形的地,是由若干块正方形的田地组成,每块地有每一块的水管路,总共有11种管道铺法,联通的管道不需要重复浇水,问最少要在几个点浇水
我的思路是用图来做一次遍历之后用并查集来储存关系

1 #include<iostream> 2 #include<cstdio> 3 #include<cstring> 4 #include<cmath> 5 #include<cstdlib> 6 #include<algorithm> 7 using namespace std; 8 #define maxm 55 9 10 bool p[11][4]; 11 int father[maxm*maxm],Map[maxm][maxm]; 12 13 int find(int x)//因为数据最大为2500不会爆栈就用递归来路径压缩 14 { 15 if(father[x] == x) return x; 16 return x = find(father[x]); 17 } 18 19 void updata(int a,int b) 20 { 21 int x = find(a); 22 int y = find(b); 23 father[y] = x; 24 } 25 26 int main() 27 { 28 int m,n; 29 char s; 30 31 //初始化一哪里通了就在那里标记1 32 memset(p,0,sizeof(p)); 33 p[0][0] = p[0][1] = 1; 34 p[1][1] = p[1][2] = 1; 35 p[2][0] = p[2][3] = 1; 36 p[3][2] = p[3][3] = 1; 37 p[4][1] = p[4][3] = 1; 38 p[5][0] = p[5][2] = 1; 39 p[6][0] = p[6][1] = p[6][2] = 1; 40 p[7][0] = p[7][1] = p[7][3] = 1; 41 p[8][0] = p[8][2] = p[8][3] = 1; 42 p[9][1] = p[9][2] = p[9][3] = 1; 43 p[10][0] = p[10][1] = p[10][2] = p[10][3] = 1; 44 /*不理解的可以用这个代码来看下 45 for(int i = 0; i < 11; i++) 46 { 47 printf("%c\n",i+'A'); 48 cout<<' '<<p[i][1]<<endl; 49 cout<<p[i][0]<<' '<<p[i][2]<<endl; 50 cout<<' '<<p[i][3]<<endl; 51 cout<<endl; 52 } 53 */ 54 55 while(cin>>m>>n,n != -1) 56 { 57 for(int i = 0; i < m ; i++) 58 for(int j = 0; j < n; j++) 59 { 60 cin>>s; 61 Map[i][j] = s - 'A'; 62 } 63 int maxn = m*n; 64 for(int i = 0; i < maxn; i++) 65 father[i] = i; 66 int x = m-1,y = n-1; 67 //遍历 68 for(int i = 0; i < x ; i++) 69 { 70 for(int j = 0; j < y; j++) 71 { 72 if(p[Map[i][j]][3] == 1 && p[Map[i+1][j]][1] == 1) 73 updata(i*n+j,i*n+n+j); 74 if(p[Map[i][j]][2] == 1 && p[Map[i][j+1]][0] == 1) 75 updata(i*n+j,i*n+j+1); 76 } 77 if(p[Map[i][y]][3] == 1 && p[Map[i+1][y]][1] == 1) 78 updata(i*n+y,i*n+n+y); 79 } 80 for(int j = 0; j < y; j++) 81 { 82 if(p[Map[x][j]][2] == 1 && p[Map[x][j+1]][0] == 1) 83 updata(x*n+j,x*n+j+1); 84 } 85 //有种代码更简便的代码不过时间为45ms 86 /*代码 87 for(int i = 0; i < m ; i++) 88 for(int j = 0; j < n; j++) 89 { 90 if(i != m-1 && p[Map[i][j]][3] == 1 && p[Map[i+1][j]][1] == 1) 91 updata(i*n+j,i*n+n+j); 92 if(j != n-1 && p[Map[i][j]][2] == 1 && p[Map[i][j+1]][0] == 1) 93 updata(i*n+j,i*n+j+1); 94 } 95 */ 96 int ans = 0; 97 for(int i = 0; i < maxn ; i++) 98 if(father[i] == i) ans++; 99 cout<<ans<<endl; 100 } 101 return 0; 102 } 103 104 /* 105 4 4 106 ACDA 107 BECF 108 IKAC 109 FCIJ 110 111 2 2 112 DK 113 HF 114 115 3 3 116 ADC 117 FJK 118 IHE 119 120 -1 -1 121 */