[Leetcode] 543. Diameter of Binary Tree_Easy Tag: DFS
2021-07-29 05:51 Johnson_强生仔仔 阅读(7) 评论(0) 编辑 收藏 举报Given the
root
of a binary tree, return the length of the diameter of the tree.The diameter of a binary tree is the length of the longest path between any two nodes in a tree. This path may or may not pass through the root
.
The length of a path between two nodes is represented by the number of edges between them.
Example 1:
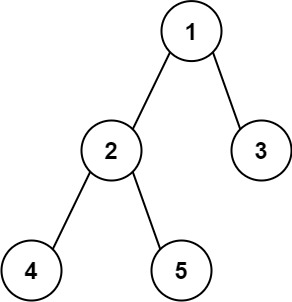
Input: root = [1,2,3,4,5] Output: 3 Explanation: 3 is the length of the path [4,2,1,3] or [5,2,1,3].
Example 2:
Input: root = [1,2] Output: 1
Constraints:
- The number of nodes in the tree is in the range
[1, 104]
. -100 <= Node.val <= 100
Ideas: 最长一条边的node的个数 - 1, 建立一个helper function, get the max number of nodes with the root, 再用self.an = max(self.ans, left + right + 1 - 1) 去得到边
Code
# Definition for a binary tree node. # class TreeNode: # def __init__(self, val=0, left=None, right=None): # self.val = val # self.left = left # self.right = right class Solution: def diameterOfBinaryTree(self, root: TreeNode) -> int: self.ans = 0 self.helperWithRoot(root) return self.ans # get the number of nodes def helperWithRoot(self, root): if not root: return 0 left = self.helperWithRoot(root.left) right = self.helperWithRoot(root.right) self.ans = max(self.ans, left + right ) return 1 + max(left, right)
get all the nodes, and then self.ans records the most nodes of one path, finally we decrease one for the ans.
# Definition for a binary tree node. # class TreeNode: # def __init__(self, val=0, left=None, right=None): # self.val = val # self.left = left # self.right = right class Solution: def __init__(self): self.ans = 0 def diameterOfBinaryTree(self, root: TreeNode) -> int: self.depth(root) return self.ans - 1 def depth(self, root): if not root: return 0 left = self.depth(root.left) right = self.depth(root.right) local = 1 + max(left, right) self.ans = max(self.ans, 1 + left + right) return local