[HDU] 3177.Crixalis's Equipment (贪心)
Problem Description
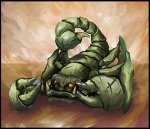
题目大意:
有V的空间以及N个物品,对于放入每个物品有Ai(放入物品后剩余空间的减少大小),Bi(放入该物品之前需要的最小剩下空间大小,如果大于当前剩余空间大小则无法放入)
Input
T组数据,每组数据有N+1行,每组数据第一行输入V,N,之后的N行每行描述一个物品的Ai,Bi
0<T<= 10, 0<V<10000, 0<N<1000, 0 <Ai< V, Ai <= Bi < 1000.
Output
For each case output "Yes" if Crixalis can move all his equipment into the new hole or else output "No".
Sample Input
2
20 3
10 20
3 10
1 7
10 2
1 10
2 11
Sample Output
Yes
No
这是一道贪心题目,贪心的策略以及证明也是看了网上dalao的思路,下面就是数学证明:
对于x(a1,b1) y(a2,b2)
先x后y所需的最大空间为 max(b1,a1+b2)
先y后x所需的最大空间则为 max(b2,a2+b1)
显然对于放入两件物品,优先考虑的就是所需空间最小的
贪心策略则为
min(max(b1,a1+b2),max(b2,a2+b1)) ①
那么就可以化简为b1,b2加上对方的ai后更小的一方
b1+a2<b2+a1 ②
则为b1-a1<b2-a2 ③
p.s.:(也许有些难以理解,但上面的①式是可以在比较函数中直接写成max(b1,a1+b2)<max(b2,a2+b1)的,一样可以AC)
p.p.s.:在下面的我的代码中,由于我采用的是降序排列,所以下面的代码则是改成max(b1,a1+b2)>max(b2,a2+b1),具体的各位dalao 看到代码应该会注意到的qwq

1 #include <iostream> 2 #include <cstdio> 3 #include <cstring> 4 #include <string> 5 #include <algorithm> 6 using namespace std; 7 8 struct pr 9 { 10 int a,b; 11 bool operator <(const pr &x) 12 { 13 if(b-a==x.b-x.a) 14 return b<x.b; 15 return b-a<x.b-x.a; 16 } 17 }p[1010]; 18 19 int main() 20 { 21 int T,n,v; 22 cin>>T; 23 while(T--) 24 { 25 scanf("%d%d",&v,&n); 26 for(int i=0;i<n;++i) 27 scanf("%d%d",&p[i].a,&p[i].b); 28 sort(p,p+n); 29 bool suc=true; 30 for(int i=n-1;i>=0;--i) 31 { 32 if(v>=p[i].b) 33 v-=p[i].a; 34 else 35 { 36 suc=false; 37 break; 38 } 39 } 40 if(suc) printf("Yes\n"); 41 else printf("No\n"); 42 } 43 return 0; 44 }
贪心虽然是比较基础的算法,但是其中很多情况的贪心策略总是不同的,平时更需要注重积累和思考qwq
继续摸鱼去了orz