[LeetCode]236. Lowest Common Ancestor of a Binary Tree
236. Lowest Common Ancestor of a Binary Tree
# Definition for a binary tree node.
# class TreeNode(object):
# def __init__(self, x):
# self.val = x
# self.left = None
# self.right = None
class Solution(object):
def lowestCommonAncestor(self, root, p, q):
"""
:type root: TreeNode
:type p: TreeNode
:type q: TreeNode
:rtype: TreeNode
"""
def lca(node):
if not node:
return None
# 找到p或者q
if node == p or node == q:
return node
left = lca(node.left)
right = lca(node.right)
# 如果同时存在,表明当前结点则为lca,其余均为处在同个方向上。
if left and right:
return node
elif left:
return left
elif right:
return right
else:
return None
return lca(root)
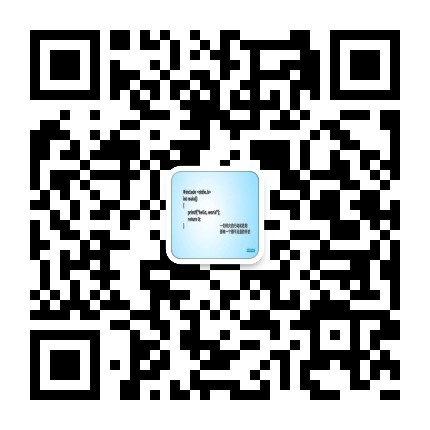
关注公众号:数据结构与算法那些事儿,每天一篇数据结构与算法