[LeetCode]567. Permutation in String
567. Permutation in String
class Solution(object):
def checkInclusion(self, s1, s2):
"""
:type s1: str
:type s2: str
:rtype: bool
"""
def backtracking(index, strs):
if index >= len(strs):
res.append(''.join(strs[:]))
return
for i in range(index, len(strs)):
if i > index and strs[i] == strs[i - 1]:
continue
strs[i], strs[index] = strs[index], strs[i]
backtracking(index+1, strs)
strs[index], strs[i] = strs[i], strs[index]
if not s1 and not s2:
return True
elif not s1 or not s2:
return False
res = []
strs = list(s1)
backtracking(0, strs)
for n in res:
if s2.find(n) != -1:
return True
return False
import collections
class Solution(object):
def checkInclusion(self, s1, s2):
"""
:type s1: str
:type s2: str
:rtype: bool
"""
counter, window = collections.Counter(s1), collections.Counter('')
for i, n in enumerate(s2):
window[n] += 1
if i >= len(s1):
window[s2[i-len(s1)]] -= 1
if window[s2[i-len(s1)]] <= 0:
del window[s2[i-len(s1)]]
if window == counter:
return True
return False
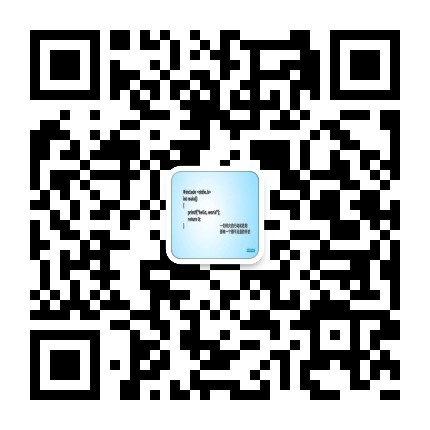
关注公众号:数据结构与算法那些事儿,每天一篇数据结构与算法