[LeetCode]79. Word Search
79. Word Search
回溯
class Solution(object):
def exist(self, board, word):
"""
:type board: List[List[str]]
:type word: str
:rtype: bool
"""
def backtracking(i, j, index, cur, visited):
if index == len(word)-1:
if (i, j) not in visited and cur + board[i][j] == word:
return True
return False
if (i, j) not in visited and i < len(board)-1 and board[i+1][j] == word[index+1] and backtracking(i + 1, j, index + 1, cur + board[i][j], visited|{(i, j)}):
return True
if (i, j) not in visited and i > 0 and board[i-1][j] == word[index+1] and backtracking(i - 1, j, index + 1, cur + board[i][j], visited|{(i, j)}):
return True
if (i, j) not in visited and j < len(board[0])-1 and board[i][j+1] == word[index+1] and backtracking(i, j + 1, index + 1, cur + board[i][j], visited|{(i, j)}):
return True
if (i, j) not in visited and j > 0 and board[i][j-1] == word[index+1] and backtracking(i, j - 1, index + 1, cur + board[i][j], visited|{(i, j)}):
return True
return False
if not board or not word:
return False
for i in range(len(board)):
for j in range(len(board[0])):
if board[i][j] == word[0] and backtracking(i, j, 0, '', set()):
return True
return False
迭代版本
import collections
class Solution(object):
def exist(self, board, word):
"""
:type board: List[List[str]]
:type word: str
:rtype: bool
"""
if not board or not word:
return False
mapping = collections.defaultdict(list)
for i in range(len(board)):
for j in range(len((board[0]))):
mapping[board[i][j]].append((i, j))
# 去除条件
for key, value in collections.Counter(word).items():
if len(mapping[key]) < value:
return False
path = []
visited = set()
stack = [(None, (i, j), 0) for i, j in mapping[word[0]]]
while stack:
pre, (i, j), idx = stack.pop()
if idx == len(word)-1:
return True
# 回溯
while path and path[-1] != pre:
visited -= {path.pop()}
path += (i, j),
visited |= {(i, j)}
stack.extend([((i, j), (x, y), idx+1) for x, y in [(i+1, j), (i-1, j), (i, j+1), (i, j-1)] if (x, y) in mapping[word[idx+1]] and (x, y) not in visited])
return False
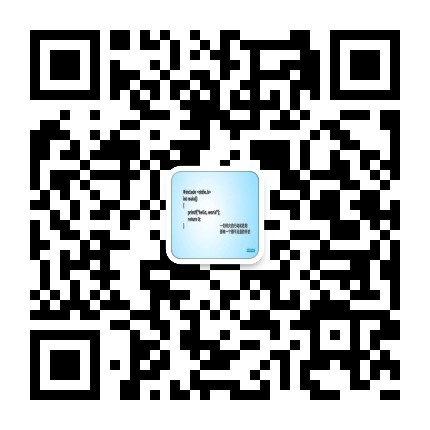
关注公众号:数据结构与算法那些事儿,每天一篇数据结构与算法