面试题3:二维数组中的查找
二维数组的查找
题型1
做法1
class Solution(object):
def searchMatrix(self, matrix, target):
"""
:type matrix: List[List[int]]
:type target: int
:rtype: bool
"""
row = len(matrix)
if row == 0:
return False
col = len(matrix[0])
if col == 0:
return False
h_row = 0
h_col = col-1
if target < matrix[0][0]:
return False
elif target > matrix[row-1][col-1]:
return False
while h_row < row and h_col >= 0:
if matrix[h_row][h_col] > target:
h_col -= 1
elif matrix[h_row][h_col] < target:
h_row += 1
else:
return True
return False
做法2
class Solution(object):
def searchMatrix(self, matrix, target):
"""
:type matrix: List[List[int]]
:type target: int
:rtype: bool
"""
if not matrix or not len(matrix[0]):
return False
top = 0
bottom = len(matrix) - 1
row = -1
while top <= bottom:
mid = top + ((bottom - top) >> 1)
if matrix[mid][0] <= target <= matrix[mid][-1]:
row = mid
break
elif target < matrix[mid][0]:
bottom = mid - 1
else:
top = mid + 1
if row == -1:
return False
left = 0
right = len(matrix[row]) - 1
while left <= right:
mid = left + ((right - left) >> 1)
if matrix[row][mid] == target:
return True
elif matrix[row][mid] < target:
left = mid + 1
else:
right = right - 1
return False
做法3
class Solution(object):
def searchMatrix(self, matrix, target):
"""
:type matrix: List[List[int]]
:type target: int
:rtype: bool
"""
m = len(matrix)
if m == 0:
return False
n = len(matrix[0])
if n == 0:
return False
left = 0
right = m * n - 1
while left <= right:
mid = left + (right - left) / 2
i = mid / n
j = mid % n
if target < matrix[i][j]:
right = mid - 1
elif target > matrix[i][j]:
left = mid + 1
else:
return True
return False
做法4
class Solution(object):
def searchMatrix(self, matrix, target):
"""
:type matrix: List[List[int]]
:type target: int
:rtype: bool
"""
for i in matrix:
if target in i:
return True
return False
class Solution(object):
def searchMatrix(self, matrix, target):
if not matrix or not matrix[0]: return False
for row in matrix:
if target==row[-1]: return True
elif target<row[-1]:
return target in row
return False
题型2
class Solution(object):
def searchMatrix(self, matrix, target):
row = len(matrix)
if not row:
return False
col = len(matrix[0])
if not col:
return False
i = 0
j = col - 1
while i < row and j >= 0:
if matrix[i][j] == target:
return True
elif matrix[i][j] < target:
i += 1
else:
j -= 1
return False
题外篇
print(0.1+0.2 == 0.3) # False
import decimal
print(decimal.Decimal('0.1') + decimal.Decimal('0.2') == decimal.Decimal('0.3')) # True
import math
print(math.isclose(0.1+0.2, 0.3)) # True
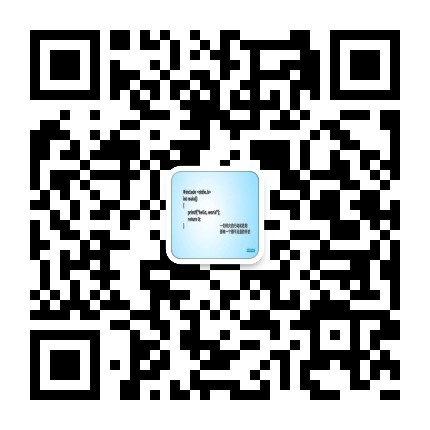
关注公众号:数据结构与算法那些事儿,每天一篇数据结构与算法