hdu 1016 Prime Ring Problem (DFS)
Prime Ring Problem
Time Limit: 4000/2000 MS (Java/Others) Memory Limit: 65536/32768 K (Java/Others)
Total Submission(s): 21703 Accepted Submission(s): 9682
Problem Description
A ring is compose of n circles as shown in diagram. Put natural number 1, 2, ..., n into each circle separately, and the sum of numbers in two adjacent circles should be a prime.
Note: the number of first circle should always be 1.
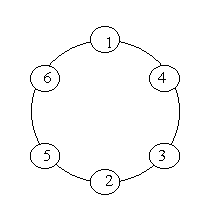
Note: the number of first circle should always be 1.
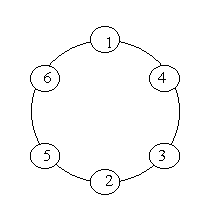
Input
n (0 < n < 20).
Output
The output format is shown as sample below. Each row represents a series of circle numbers in the ring beginning from 1 clockwisely and anticlockwisely. The order of numbers must satisfy the above requirements. Print solutions in lexicographical order.
You are to write a program that completes above process.
Print a blank line after each case.
You are to write a program that completes above process.
Print a blank line after each case.
Sample Input
6
8
Sample Output
Case 1:
1 4 3 2 5 6
1 6 5 2 3 4
Case 2:
1 2 3 8 5 6 7 4
1 2 5 8 3 4 7 6
1 4 7 6 5 8 3 2
1 6 7 4 3 8 5 2
Source
Recommend
1 //250MS 232K 1064 B G++ 2 /* 3 4 题意: 5 给出一个数n,求数1到n组成的环,环相邻的两个数和为素数。输出符合条件的环。 6 7 DFS: 8 这道题我开始看了几次都没动手,怕功力不够写不出来。后来最多了再回来做, 9 发现也算是一道基础题而已。 10 因为n比较小,我这里先预处理符合条件的一对数的情况,保存在g中。然后直接深搜, 11 再用pre数组保存没个数的前驱,最后将符合条件的pirnt出来。 12 13 */ 14 #include<stdio.h> 15 #include<string.h> 16 int g[25][25]; //预处理 17 int vis[25]; 18 int pre[25]; //前一个数 19 int n; 20 int judge(int x) 21 { 22 for(int i=2;i*i<=x;i++) 23 if(x%i==0) return 0; 24 return 1; 25 } 26 void init() 27 { 28 memset(g,0,sizeof(g)); 29 for(int i=1;i<25;i++) 30 for(int j=i+1;j<25;j++) 31 if(judge(i+j)) g[i][j]=g[j][i]=1; 32 } 33 void print(int x) 34 { 35 int ans[25],i=1; 36 ans[0]=x; 37 while(pre[x]!=-1){ 38 ans[i++]=pre[x]; 39 x=pre[x]; 40 } 41 for(int i=n-1;i>0;i--) printf("%d ",ans[i]); 42 printf("%d\n",ans[0]); 43 } 44 void dfs(int x,int cnt) 45 { 46 if(cnt==n && g[x][1]) print(x); //第一个和最后一个的和也是素数 47 for(int i=2;i<=n;i++){ 48 if(!vis[i] && g[x][i]){ 49 pre[i]=x; 50 vis[i]=1; 51 dfs(i,cnt+1); 52 vis[i]=0; 53 } 54 } 55 } 56 int main(void) 57 { 58 int k=1; 59 init(); 60 while(scanf("%d",&n)!=EOF) 61 { 62 printf("Case %d:\n",k++); 63 memset(vis,0,sizeof(vis)); 64 pre[1]=-1; 65 vis[1]=1; 66 dfs(1,1); 67 printf("\n"); 68 } 69 return 0; 70 }