poj 1486 Sorting Slides
Sorting Slides
Time Limit: 1000MS | Memory Limit: 10000K | |
http://poj.org/problem?id=1486 |
Description
Professor Clumsey is going to give an important talk this afternoon. Unfortunately, he is not a very tidy person and has put all his transparencies on one big heap. Before giving the talk, he has to sort the slides. Being a kind of minimalist, he wants to do this with the minimum amount of work possible.
The situation is like this. The slides all have numbers written on them according to their order in the talk. Since the slides lie on each other and are transparent, one cannot see on which slide each number is written.
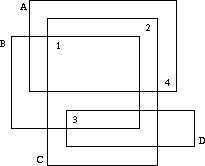
Well, one cannot see on which slide a number is written, but one may deduce which numbers are written on which slides. If we label the slides which characters A, B, C, ... as in the figure above, it is obvious that D has number 3, B has number 1, C number 2 and A number 4.
Your task, should you choose to accept it, is to write a program that automates this process.
The situation is like this. The slides all have numbers written on them according to their order in the talk. Since the slides lie on each other and are transparent, one cannot see on which slide each number is written.
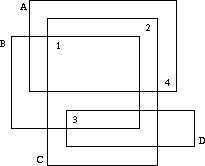
Well, one cannot see on which slide a number is written, but one may deduce which numbers are written on which slides. If we label the slides which characters A, B, C, ... as in the figure above, it is obvious that D has number 3, B has number 1, C number 2 and A number 4.
Your task, should you choose to accept it, is to write a program that automates this process.
Input
The input consists of several heap descriptions. Each heap descriptions starts with a line containing a single integer n, the number of slides in the heap. The following n lines contain four integers xmin, xmax, ymin and ymax, each, the bounding coordinates of the slides. The slides will be labeled as A, B, C, ... in the order of the input.
This is followed by n lines containing two integers each, the x- and y-coordinates of the n numbers printed on the slides. The first coordinate pair will be for number 1, the next pair for 2, etc. No number will lie on a slide boundary.
The input is terminated by a heap description starting with n = 0, which should not be processed.
This is followed by n lines containing two integers each, the x- and y-coordinates of the n numbers printed on the slides. The first coordinate pair will be for number 1, the next pair for 2, etc. No number will lie on a slide boundary.
The input is terminated by a heap description starting with n = 0, which should not be processed.
Output
For each heap description in the input first output its number. Then print a series of all the slides whose numbers can be uniquely determined from the input. Order the pairs by their letter identifier.
If no matchings can be determined from the input, just print the word none on a line by itself.
Output a blank line after each test case.
If no matchings can be determined from the input, just print the word none on a line by itself.
Output a blank line after each test case.
Sample Input
4 6 22 10 20 4 18 6 16 8 20 2 18 10 24 4 8 9 15 19 17 11 7 21 11 2 0 2 0 2 0 2 0 2 1 1 1 1 0
Sample Output
Heap 1 (A,4) (B,1) (C,2) (D,3) Heap 2 none
题解:这题就是烦人的幻灯片的改编版,原来是要求全部匹配,这次是要求输出最大的匹配(我因为这个WA了一下午,我自己都佩服自己);
中间有不确定的不用管;
1.拓扑:于是拓扑排序时就不能只看幻灯片或者数字的度数,要两者结合才能找最大匹配,因为幻灯片一对多或者数字一对多都是存在的;

#include<cstdio> #include<queue> #include<cstring> #include<algorithm> using namespace std; #define ll long long const int M = 70; int xl[M], xr[M], deg[M], yl[M], yr[M], xp[M], mp[M][M], cp[M]; bool vis[M]; int main(){ int idc = 0, n; while(scanf("%d", &n) && n){ memset(deg, 0, sizeof(deg)); memset(mp, 0, sizeof(mp)); memset(cp, 0, sizeof(cp)); memset(vis, 0, sizeof(vis)); int ans = 0; for(int i = 1; i <= n; i++)scanf("%d%d%d%d", &xl[i], &xr[i], &yl[i], &yr[i]); for(int i = 1; i <= n; i++){ int x, y; scanf("%d%d", &x, &y); for(int j = 1; j <= n; j++) if(x <= xr[j] && x >= xl[j] && y <= yr[j] && y >= yl[j]){ mp[i][j + n] = mp[j + n][i] = 1; deg[i]++;deg[j + n]++; } } queue <int> q; for(int i = 1; i <= 2*n; i++) if(deg[i] == 1){ q.push(i); } while(!q.empty()){ int u = q.front(); q.pop(); if(vis[u])continue; vis[u] = 1; for(int i = 1; i <= 2 * n; i++){ if(mp[u][i]){ cp[u] = i, cp[i] = u; vis[i] = 1; deg[i]--; deg[u]--; for(int j = 1; j <= 2*n; j++) if(mp[i][j]) { deg[j]--, mp[i][j] = mp[j][i] = 0; if(deg[j] == 1)q.push(j); } break; } } } for(int i = 1; i <= n; i++) if(cp[i])ans = -1; printf("Heap %d\n", ++idc); if(ans == 0)puts("none"); else { for(int i = 1 + n; i <= 2*n; i++) if(cp[i]){ printf("(%c,%d) ", i - n - 1 + 'A', cp[i]); } puts(""); } puts(""); } }
2.二分图匹配,因为幻灯片和数字是一一对应关系,所以可以往这方面联想;
原图显然是找一个完美匹配,必须这个匹配必须唯一;
我们考虑这个匹配中的边,如果删除他,匹配数减少,说明他是一条必须边,这个匹配是可以唯一确定的;
我们就枚举每条边看是否为必须边

#include<cstdio> #include<queue> #include<cstring> #include<algorithm> using namespace std; #define ll long long const int M = 70; int xl[M], xr[M], yl[M], tot, t[M], yr[M], mp[M][M], lik[M]; bool vis[M]; int cc, n; bool dfs(int u){ for(int i = 1 + n; i <= 2 * n; i++){ if(mp[u][i] && mp[u][i] != cc && !vis[i]){ vis[i] = 1; if(!lik[i] || dfs(lik[i])){ lik[i] = u; lik[u] = i; return 1; } } } return 0; } int hungry(){ memset(lik, 0, sizeof(lik)); int ans = 0; for(int i = 1; i <= n; i++){ memset(vis, 0, sizeof(vis)); if(dfs(i))ans++; } return ans; } int main(){ int idc = 0; while(scanf("%d", &n) && n){ memset(t, 0, sizeof(t)); memset(mp, 0, sizeof(mp)); cc = 0; tot = 0; int ans = 0; for(int i = 1; i <= n; i++)scanf("%d%d%d%d", &xl[i], &xr[i], &yl[i], &yr[i]); for(int i = 1; i <= n; i++){ int x, y; scanf("%d%d", &x, &y); for(int j = 1; j <= n; j++) if(x <= xr[j] && x >= xl[j] && y <= yr[j] && y >= yl[j])mp[j][i + n] = ++tot; } printf("Heap %d\n", ++idc); int Max = hungry(), fg = 0; for(int i = 1; i <= n; i++)t[i] = lik[i]; for(int i = 1; i <= n; i++){ if(t[i]){ cc = mp[i][t[i]]; int tmp = hungry(); if(tmp < n) printf("(%c,%d) ", i - 1 + 'A', t[i] - n), fg = 1; } } if(!fg)printf("none"); puts("");puts(""); } }