上机练习:
1.使用for循环计算1-100的和,除了以3结尾的那些数
2.package text;
3.
4.public class test 1{
5.
6. /**
7. * @param args
8. */
9. public static void main(String[] args) {
10. // TODO Auto-generated method stub
11. int sum=0;
12. for(int i=1;i<=100;i++){
13. if(i!=3&&i%10!=3){
14. sum+=i;
15. }
16. }
17. System.out.println("sum="+sum);
18. }
19.
20.}
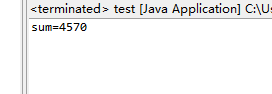
2.使用二重循环输出以下图形
*
***
*****
*******
*****
****
***
**
*
package text;
public class test2 {
/**
* @param args
*/
public static void main(String[] args) {
// TODO Auto-generated method stub
for(int i=1;i<=5;i++){
for(int j=1;j<=6-i;j++){
System.out.print("*");
}
System.out.println();
}
}
}
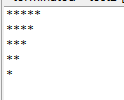
3.循环输入学生姓名,直到输入‘Q’程序结束。
package text;
import java.util.Scanner;
public class test3 {
/**
* @param args
*/
public static void main(String[] args) {
// TODO Auto-generated method stub
Scanner input=new Scanner(System.in);
System.out.println("输入学生姓名");
int i=1;
while(i<=100){
String name=input.next();
if(name.equals("Q")){
System.out.println("程序结束");
break;
}
}
}
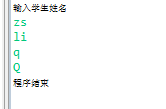
4.输入5个学生成绩,求和,当输入不合法的时候,提示输入错误重新输入。
package text;
import java.util.Scanner;
public class test4 {
/**
* @param args
*/
public static void main(String[] args) {
// TODO Auto-generated method stub
Scanner input=new Scanner(System.in);
System.out.println("输入学生成绩");
int i=1;
int sum=0;
while(i<=5){
int grate=input.nextInt();
if(grate<0||grate>100){
System.out.println("输入格式错误");
break;
}
sum+=grate;
i++;
}
System.out.println("sum="+sum);
}
}
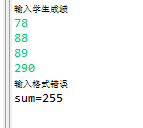
计算 1+1/(1+2) + 1/(1+2+3) +1/(1+2+3+4)+…………+1/(1+2+....+10)
package text;
public class text5{
/**
* @param args
*/
public static void main(String[] args) {
// TODO Auto-generated method stub
double sum = 0;
double a = 0;
for (int i = 1; i <= 10; i++) {
a += i;
sum += 1 / a;
}
System.out.println("sum=" + sum);
}
}
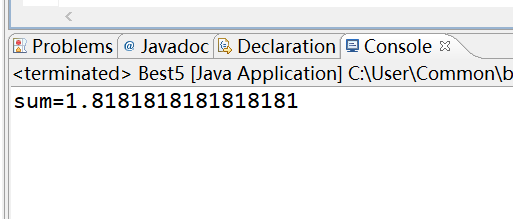
作业
1.产生一个1-99的随机数,猜数字,若大了就提示“大了点”,若小了就提示“小了点”直到猜对为止。
(判断猜的次数,如果1-3次,提示太棒了 如果5-8次 一般般 如果8次以上 太笨了)
import java.util.Random;
import java.util.Scanner;
public class text {
/**
* @param args
*/
public static void main(String[] args) {
// TODO Auto-generated method stub
int c;
Random r = new Random();
int a = r.nextInt(100);
System.out.println("请猜数字");
for (c = 1; c < c + 1; c++) {
Scanner input = new Scanner(System.in);
int b = input.nextInt();
if (b > a)
System.out.println("大了点");
else if (b < a)
System.out.println("小了点");
else {
System.out.println("猜对啦");
if (c < 3 && c > 1)
System.out.println("太棒啦");
else if (c > 8)
System.out.println("太笨啦");
else
System.out.println("一般般啦");
break;
}
}}
}
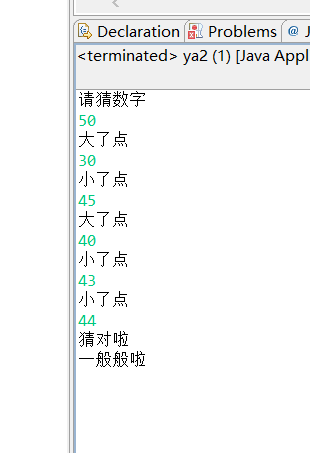
2.输入一个数,判断是不是质数(只能被1和自身整除的数是质数)
package text;
import java.util.Scanner;
public class test2 {
/**
* @param args
*/
public static void main(String[] args) {
// TODO Auto-generated method stub
Scanner input = new Scanner(System.in);
System.out.println("输入一个数");
int num = input.nextInt();
boolean isPrime = true;
if (num == 1) {
isPrime = false;
}
for (int i = 2; i <= num - 1; i++) {
if (num % i == 0) {
isPrime = false;
break;
}
}
if (isPrime) {
System.out.println(num + "是质数");
} else {
System.out.println(num + "不是质数");
}
}
}
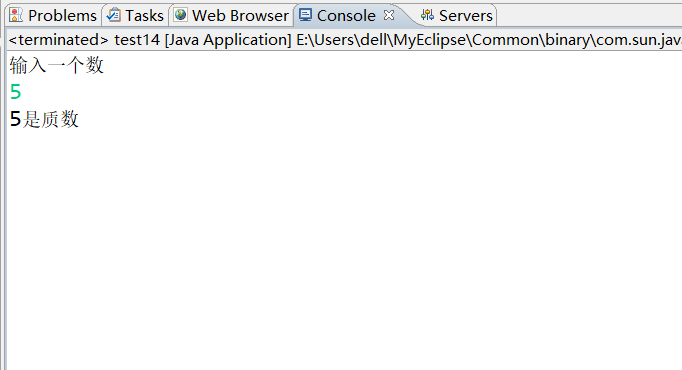