poj 2451:Uyuw's Concert
Time Limit: 6000MS | Memory Limit: 65536K | |
Total Submissions: 8669 | Accepted: 3269 |
Description
Prince Remmarguts solved the CHESS puzzle successfully. As an award, Uyuw planned to hold a concert in a huge piazza named after its great designer Ihsnayish.
The piazza in UDF - United Delta of Freedom’s downtown was a square of [0, 10000] * [0, 10000]. Some basket chairs had been standing there for years, but in a terrible mess. Look at the following graph.
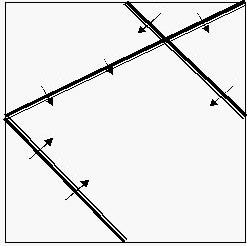
In this case we have three chairs, and the audiences face the direction as what arrows have pointed out. The chairs were old-aged and too heavy to be moved. Princess Remmarguts told the piazza's current owner Mr. UW, to build a large stage inside it. The stage must be as large as possible, but he should also make sure the audience in every position of every chair would be able to see the stage without turning aside (that means the stage is in the forward direction of their own).
To make it simple, the stage could be set highly enough to make sure even thousands of chairs were in front of you, as long as you were facing the stage, you would be able to see the singer / pianist – Uyuw.
Being a mad idolater, can you tell them the maximal size of the stage?
The piazza in UDF - United Delta of Freedom’s downtown was a square of [0, 10000] * [0, 10000]. Some basket chairs had been standing there for years, but in a terrible mess. Look at the following graph.
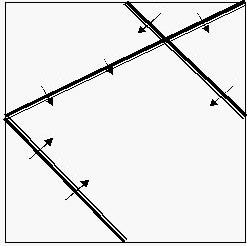
In this case we have three chairs, and the audiences face the direction as what arrows have pointed out. The chairs were old-aged and too heavy to be moved. Princess Remmarguts told the piazza's current owner Mr. UW, to build a large stage inside it. The stage must be as large as possible, but he should also make sure the audience in every position of every chair would be able to see the stage without turning aside (that means the stage is in the forward direction of their own).
To make it simple, the stage could be set highly enough to make sure even thousands of chairs were in front of you, as long as you were facing the stage, you would be able to see the singer / pianist – Uyuw.
Being a mad idolater, can you tell them the maximal size of the stage?
Input
In the first line, there's a single non-negative integer N (N <= 20000), denoting the number of basket chairs. Each of the following lines contains four floating numbers x1, y1, x2, y2, which means there’s a basket chair on the line segment of (x1, y1) – (x2, y2), and facing to its LEFT (That a point (x, y) is at the LEFT side of this segment means that (x – x1) * (y – y2) – (x – x2) * (y – y1) >= 0).
Output
Output a single floating number, rounded to 1 digit after the decimal point. This is the maximal area of the stage.
Sample Input
3
10000 10000 0 5000
10000 5000 5000 10000
0 5000 5000 0
Sample Output
54166666.7
Hint
Sample input is the same as the graph above, while the correct solution for it is as below:
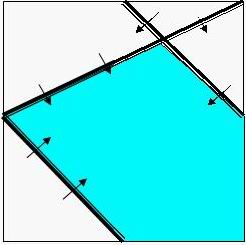
I suggest that you use Extended in pascal and long double in C / C++ to avoid precision error. But the standard program only uses double.
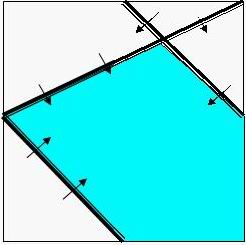
I suggest that you use Extended in pascal and long double in C / C++ to avoid precision error. But the standard program only uses double.
1 #include<iostream> 2 #include<cstdio> 3 #include<cstdlib> 4 #include<cstring> 5 #include<cmath> 6 #include<algorithm> 7 #include<queue> 8 #include<vector> 9 using namespace std; 10 const double eps=1e-9; 11 struct P{ 12 double x,y; 13 }p[20005]; 14 struct L{ 15 P t1,t2; double angle; 16 }A[20005],q[20005]; 17 18 double ANS; 19 int N,top,bot,tot; 20 inline P operator-(P a,P b){ 21 P t; t.x=a.x-b.x; t.y=a.y-b.y; 22 return t; 23 } 24 inline double operator*(P a,P b){ 25 return a.x*b.y-b.x*a.y; 26 } 27 inline bool operator<(L a,L b){ 28 if(a.angle==b.angle) return (b.t2-a.t1)*(b.t1-a.t1)>0;//越靠右,排名越靠前,会被覆盖掉 29 return a.angle<b.angle; 30 } 31 inline P inter(L a,L b){ 32 double k1,k2;//k1 k2 是两条线段四点连线所形成的两个三角形面积 33 k1=(b.t1-a.t1)*(b.t2-a.t1); 34 k2=(b.t2-a.t2)*(b.t1-a.t2); 35 P ans; 36 ans.x=((a.t1.x)*(k2)+(a.t2.x)*(k1))/(k1+k2); 37 ans.y=((a.t1.y)*(k2)+(a.t2.y)*(k1))/(k1+k2); 38 return ans; 39 } 40 inline bool jud(L a,L b,L t){//判断a和b的交点是不是在t内 ,在外部返回true 41 P tmp=inter(a,b); 42 return (t.t1-tmp)*(t.t2-tmp)<0; 43 } 44 inline void hpi(){ 45 sort(A+1,A+N+1);//极角排序 46 for(int i=1;i<=N;i++){//去重 47 if(A[i].angle!=A[i-1].angle) tot++; 48 A[tot]=A[i]; 49 } 50 N=tot; q[0]=A[1]; q[1]=A[2]; 51 top=1; bot=0; 52 for(int i=3;i<=N;i++){ 53 while(bot<top&&jud(q[top],q[top-1],A[i])) top--; 54 while(bot<top&&jud(q[bot],q[bot+1],A[i])) bot++; 55 q[++top]=A[i]; 56 } 57 while(bot<top&&jud(q[top],q[top-1],q[bot])) top--; 58 while(bot<top&&jud(q[bot],q[bot+1],q[top])) bot++; 59 q[top+1]=q[bot]; 60 tot=0; 61 for(int i=bot;i<=top;i++){ 62 p[++tot]=inter(q[i],q[i+1]); 63 } 64 } 65 inline void getans(){ 66 if(tot<3) return ; 67 p[++tot]=p[1]; 68 for(int i=1;i<=tot;i++){ 69 ANS+=p[i]*p[i+1]; 70 } 71 ANS=fabs(ANS)/2; 72 } 73 int main(){ 74 scanf("%d",&N); 75 for(int i=1;i<=N;i++){ 76 scanf("%lf%lf%lf%lf",&A[i].t1.x,&A[i].t1.y,&A[i].t2.x,&A[i].t2.y); 77 } 78 //加入边界的四条线段 79 A[++N].t1.x=0; A[N].t1.y=0; A[N].t2.x=10000; A[N].t2.y=0;//(0,0) (10000,0) 80 A[++N].t1.x=0; A[N].t1.y=10000; A[N].t2.x=0; A[N].t2.y=0;//(0,10000) (0,0) 81 A[++N].t1.x=10000; A[N].t1.y=10000; A[N].t2.x=0; A[N].t2.y=10000;//(10000,10000) (0,10000) 82 A[++N].t1.x=10000; A[N].t1.y=0; A[N].t2.x=10000; A[N].t2.y=10000;//(10000,0) (10000,10000) 83 84 for(int i=1;i<=N;i++){//计算每条线段的弧度 85 A[i].angle=atan2((A[i].t2.y-A[i].t1.y),(A[i].t2.x-A[i].t1.x)); 86 } 87 hpi(); 88 getans(); 89 printf("%.1lf",ANS); 90 return 0; 91 }