陈凯迪的实验3
一、实验结论
1
代码:

1 #include <stdio.h> 2 #include <stdlib.h> 3 #include <time.h> 4 #include <windows.h> 5 #define N 80 6 7 void printText(int line, int col,char text[]); 8 void printSpace(int n); 9 void printBlankLines(int n); 10 11 int main(){ 12 int line ,col,i; 13 char text[N] = "hi, May~"; 14 15 srand(time(0)); 16 17 for(i=1;i<=10;i++){ 18 line = rand()%25; 19 col=rand()%80; 20 printText(line,col,text); 21 Sleep(1000); 22 } 23 return 0; 24 } 25 26 void printSpace(int n){ 27 int i; 28 for(i=1;i<=n;i++) 29 printf(" "); 30 } 31 32 void printBlankLines(int n){ 33 int i; 34 for(i=1;i<=n;i++) 35 printf("\n"); 36 } 37 38 void printText(int line,int col,char text[]){ 39 printBlankLines(line-1); 40 printSpace(col-1); 41 printf("%s",text); 42 }
图片:

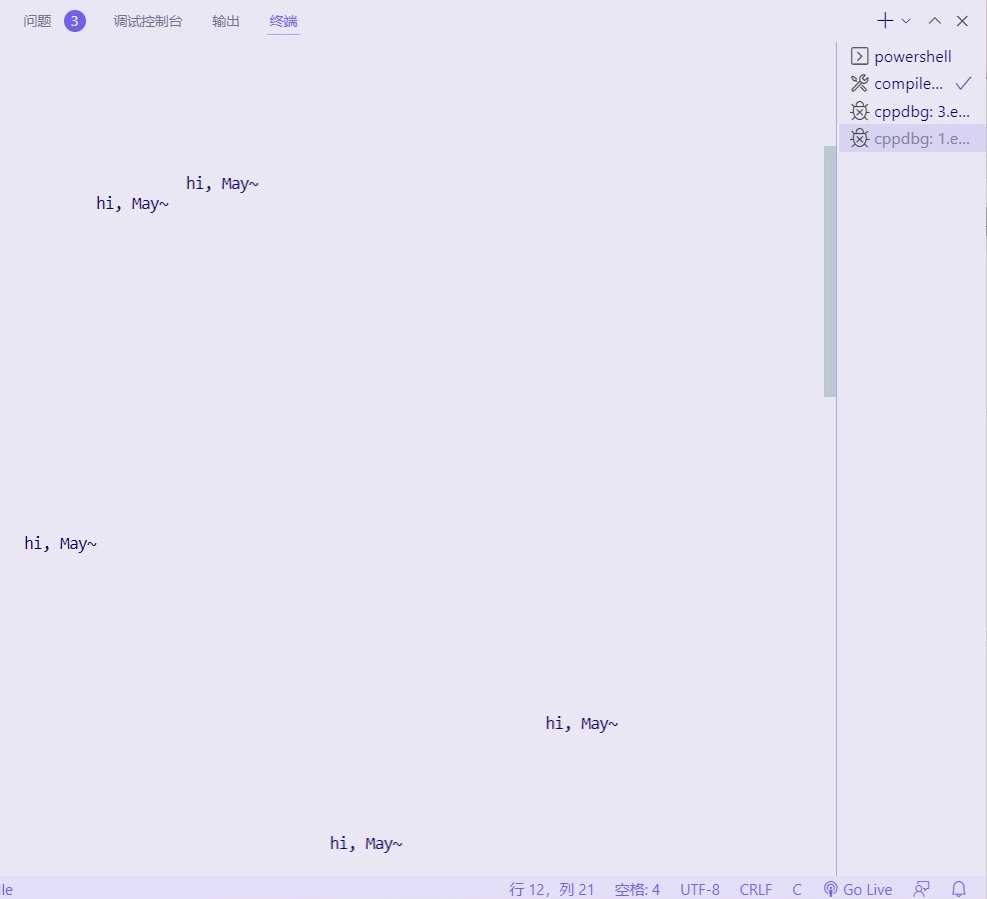
功能:

随机位置生成字符串
2.1
代码:

#include <stdio.h> long long fac(int n); int main(){ int i,n; printf("Enter n:"); scanf("%d",&n); for(i=1;i<=n;++i) printf("%d! = %lld\n",i,fac(i)); return 0; } long long fac(int n){ static long long p =1; p*=n; return p; }
图片:

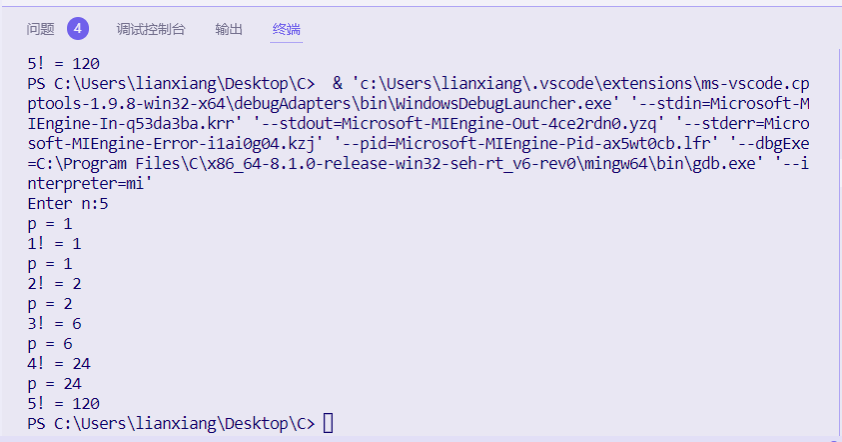
2.2
代码:

1 #include <stdio.h> 2 #define P(m) printf("%d\n",m) 3 int func(int,int); 4 int main(){ 5 int k=4,m=1,p1,p2; 6 p1=func(k,m); 7 P(p1); 8 p2=func(k,m); 9 P(p2); 10 printf("%d,%d\n",p1,p2); 11 return 0; 12 } 13 14 int func(int a,int b){ 15 static int m=0,i=2; 16 i+=m+1; 17 m=i+a+b; 18 return m; 19 }
图片:

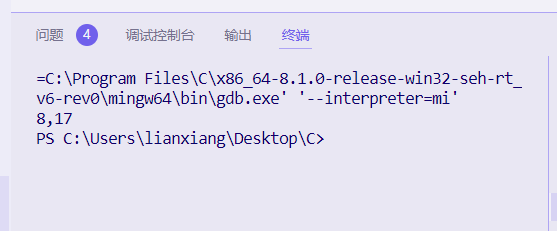
2.3 理解

1、一致 2、static变量不会被再次初始化
3
代码:

1 #include <stdio.h> 2 3 int fun(int n); 4 int main(){ 5 long long i,j; 6 while(scanf("%lld",&i)!=EOF){ 7 j=fun(i); 8 printf("n = %lld,f = %lld\n",i,j); 9 } 10 return 0; 11 12 13 } 14 int fun(int n){ 15 long long f; 16 if(n==0) 17 f=0; 18 else 19 f=2*(fun(n-1)+1)-1; 20 21 return(f); 22 }

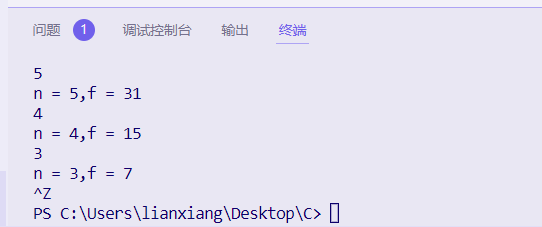
4
代码:

1 #include <stdio.h> 2 void movePlate(int n,char from,char to); 3 void Hanoi(unsigned int n,char from,char temp,char to); 4 int m; 5 int main(){ 6 int n; 7 while(scanf("%d",&n)!=EOF){ 8 Hanoi(n,'A','B','C'); 9 printg("一共移动了%d次\n",m); 10 m=0; 11 } 12 return 0; 13 } 14 void movePlate(int n,char from,char to){ 15 printf("%u %u:%c-->%c\n",++m,n,from,to); 16 } 17 void Hanoi(unsigned int n,char from,char temp,char to){ 18 if(n==1) 19 movePlate(n,from,to); 20 else{ 21 Hanoi(n-1,from,to,temp); 22 movePlate(n,from,to); 23 Hanoi(n-1,temp,from,to); 24 } 25 }

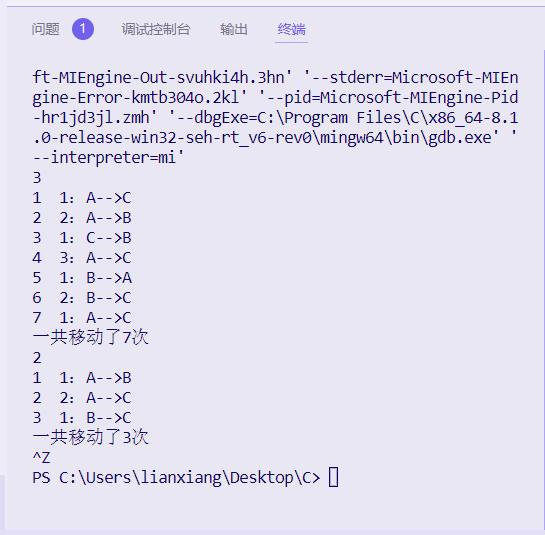
5.1(原始)
代码:

1 #include <stdio.h> 2 int isPrime(int n); 3 int main() { 4 int i, j, x; 5 for (x = 4; x <= 20; x += 2) { 6 for (i = 2; i < x; i++) 7 if (isPrime(i)) { 8 for (j = 2; j < x; j++) 9 if (isPrime(j)) 10 if (x == i + j) 11 break; 12 if (j < x) { 13 printf("%d=%d+%d\n", x, i, j); 14 break; 15 } 16 } 17 } 18 return 0; 19 } 20 int isPrime(int n) { 21 int i; 22 for (i = 2; i < n; i++) 23 if (n % i == 0) 24 break; 25 if (i == n) 26 return 1; 27 else 28 return 0; 29 }
5.2(改进)
代码:

1 #include <stdio.h> 2 int isPrime(int n); 3 int main() { 4 int i, x; 5 for (x = 4; x <= 20; x += 2) { 6 for (i = 2; i < x; i++) 7 if (isPrime(i) && isPrime(x - i)) { 8 printf("%2d=%d+%d\n", x, i, x - i); 9 break; 10 } 11 } 12 return 0; 13 } 14 int isPrime(int n) { 15 int i; 16 for (i = 2; i < n; i++) 17 if (n % i == 0) 18 break; 19 if (i == n) 20 return 1; 21 else 22 return 0; 23 }

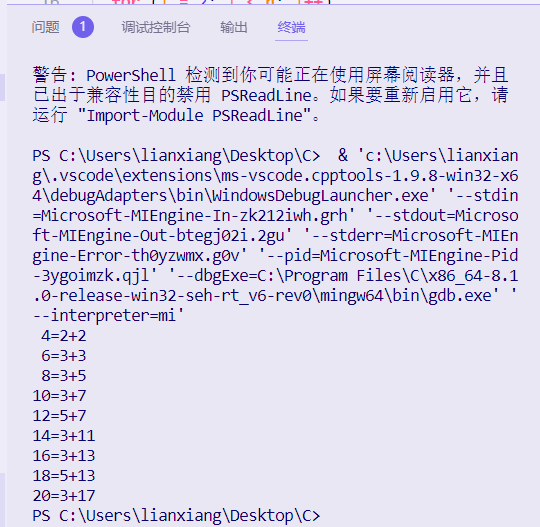
6
代码:

1 #include <stdio.h> 2 #define CRT_SECURE_NO_WARNINGS 3 long fun(long s); 4 int main(){ 5 long s,t; 6 printf("Enter a number:"); 7 while(scanf("%ld",&s)!=EOF){ 8 t = fun(s); 9 printf("new number is:%ld\n\n",t); 10 printf("Enter a new number:"); 11 12 } 13 return 0; 14 } 15 long fun(long s){ 16 int a[10],i=0; 17 while(s>0){ 18 a[i++]=s%10; 19 s=s/10; 20 } 21 for(i--;i>=0;i--) 22 if(a[i]%2) 23 s=s*10+a[i]; 24 return s; 25 }
图片:

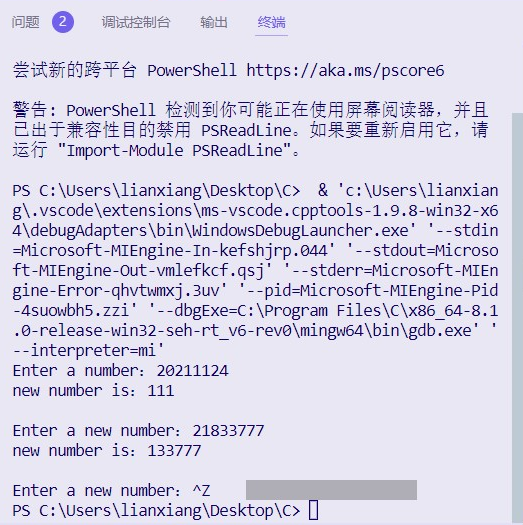
二、实验总结
学会了stastic变量的用法,编写代码更方便了。在教别人的同时优化了自己的算法,教学相长也ヽ(゚∀゚)メ(゚∀゚)ノ