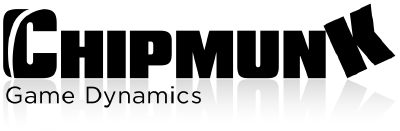
Note: The documentation on this wiki documents Chipmunk v5.0.0. First of all, Chipmunk is a 2D rigid body physics library distributed under the MIT license. Though not yet complete, it is intended to be fast, numerically stable, and easy to use. I've put hundreds of hours of work into making Chipmunk what it is today. If you find Chipmunk has saved you a lot of time, please consider donating. You'll make an indie game developer very happy. I would like to give a Erin Catto a big thank you, as the most of the ideas for the constraint solver I use now come from his Box2D example code. (Now a full fledged physics engine all it's own: Box2D). His contact persistence idea allows for stable stacks of objects with very few iterations of the contact solution. Previously my solver produced mushy piles of objects or required a large number of iterations in order to get a stable behavior. 这个文档针对于v5 .0而言 首先,Chipmunk是一个2D的刚体物理引擎库,遵守MIT协议。虽然现在还没有完成,但是它被设计更快,更稳定,使用更简单。我已经投入了上百个小时在Chipmunk里面如果你发现使用了Chipmunk能给你节省时间,那么你可以考虑捐赠。它能够是游戏开发者变得快乐。 我非常的感谢Erin Catto, 因为它是我从众多的解决方案中Box2D例子得来得。。。。。略。。 There are 4 basic things you need to know about in order to work with Chipmunk. 为了能够使用Chipmunk,你需要按顺序了解以下几个基本特征。 - Rigid Bodies: A rigid body holds the physical properties of an object. (mass, position, rotation, velocity, etc.) It does not have a shape by itself. If you’ve done physics with particles before, rigid bodies differ in that they are able to rotate.
- 刚体,是一个对象的物理属性,他没有自己的形状,如果在物力上对粒子有了解,刚体不同于粒子,它可以旋转。
- Collision Shapes: By attaching shapes to bodies, you can define the a body’s shape. You can attach as many shapes to a single body as you need to in order to define a complex shape. Shapes contain the surface properties of an object such as how much friction or elasticity it has.
- 冲突形状:通过物体形状的附加,你可以定义一个物体的形状。你可以给一个单一的物体附上很多你需要的形状,很复杂的形状。形状包括表面的属性,就好像有多少摩擦或者弹性(elasticity猜的)
- Constraints/Joints: Constraints and joints describe how bodies are attached to each other.
- 限制和连接:描述物体之间如何接触。
- Spaces: Spaces are the basic simulation unit in Chipmunk. You add bodies, shapes and joints to a space, and then update the space as a whole.
- 空间:Chipmunk最基本单元的模拟。添加物体,形状,到一个空间,然后以一个整体进行更新这个空间。
The best way to get support is to visit the Chipmunk Forums. There are plenty of people around using Chipmunk on the just about every platform I've ever heard of. If you are working on a commercial project, Howling Moon Software is also available for contracting. Chipmunk最好的支持就是Chipmunk论坛,它有很多使用Chipmunk的开发人员,它们把它应用在很多我所听过的平台。如果你工作在一个商业的项目上, 签协议? There is often confusion between rigid bodies and their collision shapes in Chipmunk and how they relate to sprites. A sprite would be a visual representation of an object, the sprite is drawn at the position and rotation of the rigid body. Collision shapes representation of the object, and how it should collide with other objects. A sprite and collision shape have little to do with one another other than you probably want the collision shape to match the sprite’s shape. 人们常常混淆这刚体,冲突和精灵的关系。精灵是一个虚拟的,物体的呈现,精灵以一种位置和旋转过的刚体位置来绘制。冲突形态表现的是物体的冲突,它和其他物体冲突的时候应该如何表现。精灵和冲突形态之间需要做的就是,你可能回把冲突形态与精灵的形状向匹配。 Before you do anything else, you must initialize Chipmunk. Otherwise you will crash as soon as the first collision happens. cpInitChipmunk(); // That is all Additionally, if you didn't compile Chipmunk with the NDEBUG flag, it will print out the debug mode message and the current version number to stdout.
再你开始之前,你必须初始化 Chipmunk,否则将会crash,初始化使用以上那个函数。除此之外,如果你还没有通过NDEBUG flag 编译 Chipmunk的话,你需要打印出debug模式的信息,和当前的stdout版本号。 - cpSpaceAlloc() allocates but does not initialize a cpSpace struct.
- cpSpaceInit(cpSpace *space) initializes a cpSpace struct.
- cpSpaceDestroy(cpSpace *space) frees all memory allocated by the cpSpaceInit(), but does not free the cpSpace struct itself.
- cpSpaceNew() allocates and initializes a cpSpace struct (calls cpSpaceAlloc () and cpSpaceInit()).
- cpSpaceFree(cpSpace *space) calls cpSpaceDestroy() and then frees the cpSpace struct.
While you will probably use the new/free versions exclusively if you are using C/C++, but the alloc/init/destroy functions can be helpful when writing language extensions so that you can more easily work with a garbage collector. In general, you are responsible for freeing any structs that you allocate. Chipmunk does not have any fancy reference counting or garbage collection. 当你使用c/c++的时候,你可能选择不去使用new/free分配内存。但是分配/初始化/销毁 函数将会给你的语言扩展带来很大的帮助,所以会使得垃圾回收机制变得更加容易的工作。 通常来讲,你要对你自行分配的任何结构使用free来销毁这是你的责任。 Chipmunk没有任何引用记数和垃圾回收?(没看懂什么意思) Math the Chipmunk way 匹配First of all, Chipmunk uses double precision floating point numbers throughout it's calculations by default. This is likely to be faster on most modern desktop processors, and means you have to worry less about floating point round off errors. You can change the floating point type used by Chipmunk when compiling the library. Look in chipmunk.h. 首先, Chipmunk在计算的时候,缺省使用的双精度浮点数。这是对于当前的桌面处理器来说,很快速。并且意味着你不用去担心舍去所出现的问题。你可以改变浮点的type,当你编译库的时候。详细的参看 Chipmunk.h Chipmunk defines a number of aliases for common math functions so that you can choose to use floats or doubles for Chipmunk's floating point type. In your own code, there probably isn't a strong reason to use these aliases unless you expect you might want to change Chipmunk's floating point type later and a 2% performance hit from using the wrong float/double version of math functions will matter.
Chipmunk定义了一些列的数学运算函数,这使得你可以选择使用单精度或者是双精度浮点类型。 在你自己的代码中,这可能不是一个很大的因素去使用这些函数,除非你期望改变Chipmunk浮点数的类型,使用了float/double造成的并且一个2%性能损失,并不会太大。 That said, there are a few functions you will probably find very useful: cpFloat cpfclamp(cpFloat f, cpFloat min, cpFloat max) Clamp f to be between min and max. cpFloat cpflerp(cpFloat f1, cpFloat f2, cpFloat t) Linearly interpolate between f1 and f2. f1和f2之间额外的直线 cpFloat cpflerpconst(cpFloat f1, cpFloat f2, cpFloat d) Linearly interpolate from f1 towards f2 by d. To represent vectors, Chipmunk defines the cpVect type and a set of inline functions for working with them (cpv, cpvadd, cpvmult, etc). See the API reference for more information.. Chipmunk C API (c语言API)Main API 主要的API- cpVect - Create and manipulate 2D vectors. 创建和操作一个2D的矢量
- cpBB - Create and manipulate 2D axis-aligned bounding boxes. 创建和操作一个2D坐标轴绑定的box
- cpBody - Create and work with rigid bodies. 创建一个具有刚体属性的物体
- cpShape - Attach collision shapes to rigid bodies. 给刚体加上一个冲突形状
- cpSpace - Create a "space" to put your objects into and simulate them. 给物体和模拟它们之间创建一个空间
- cpConstraint - Create joints and other constraints. 创建一个连接和其他约束
- Learn about how CollisionDetection in Chipmunk works. 学习如何处理
Chipmunk冲突工作 - Learn about Chipmunk's CallbackSystem and cpArbiter struct for recieving collision events and adding custom behavior to your physics. 以上两个结构接受冲突时间,加入一个自定义的物理表现。
- Learn about Queries. Point queries and segment queries (raycasting). 学习如何查询,指出查询的片断。
Links (以下就是相关的链接) 本来说好下午翻译,或者先看看cocos2d的应用的。结果郁闷的无所事事的墨迹到晚上。。。k。。 只能翻译好了再睡觉。。MACOS输入法好难用啊。。 注:本文不是全文一句句的翻译。大概就是那么个意思。大体的讲讲。不重要的一概忽略了。。需要的,自己详细读吧。不过这也只是一个概述而已。。。具体还要深入分析研究才是。。 |