实验3 转移指令跳转原理及其简单应用编程
实验任务1
assume cs:code, ds:data data segment x db 1, 9, 3 len1 equ $ - x ; y dw 1, 9, 3 len2 equ $ - y ; data ends code segment start: mov ax, data mov ds, ax mov si, offset x ; mov cx, len1 ; mov ah, 2 s1:mov dl, [si] or dl, 30h int 21h mov dl, ' ' int 21h ; inc si loop s1 mov ah, 2 mov dl, 0ah int 21h ; mov si, offset y ; mov cx, len2/2 ; mov ah, 2 s2:mov dx, [si] or dl, 30h int 21h mov dl, ' ' int 21h ; add si, 2 loop s2 mov ah, 4ch int 21h code ends end start
运行结果:输出两行1 9 3
① line27, 汇编指令 loop s1 跳转时,是根据位移量跳转的。通过debug反汇编,查看其机器码,分析其跳转的位移量是多少?(位移量数值以十进制数值回答)从CPU的角度,说明 是如何计算得到跳转后标号s1其后指令的偏移地址的。
位移量是:14(Loop指令结束地址为:001B,s1指令开始地址为000D,001B-000D=14)
分析:or dl, 30h命令占三个字节,inc指令占一个字节,其他指令各占两个字节,加起来一共14字节。
② line44,汇编指令 loop s2 跳转时,是根据位移量跳转的。通过debug反汇编,查看其机 器码,分析其跳转的位移量是多少?(位移量数值以十进制数值回答)从CPU的角度,说明 是如何计算得到跳转后标号s2其后指令的偏移地址的。
位移量是:16(Loop指令结束地址为:0039,s1指令开始地址为0029,001B-000D=16)
分析:or dl, 30h命令占三个字节,其他指令各占两个字节,加起来一共16字节。
实验任务2
assume cs:code, ds:data data segment dw 200h, 0h, 230h, 0h data ends stack segment db 16 dup(0) stack ends code segment start: mov ax, data mov ds, ax mov word ptr ds:[0], offset s1 mov word ptr ds:[2], offset s2 mov ds:[4], cs mov ax, stack mov ss, ax mov sp, 16 call word ptr ds:[0] s1: pop ax call dword ptr ds:[2] s2: pop bx pop cx mov ah, 4ch int 21h code ends end start
问题①
根据call指令的跳转原理,先从理论上分析,程序执行到退出(line31)之前,寄存器(ax) = ? 寄存器
(bx) = ? 寄存器(cx) = ?
ax = offset s1,即s1的偏移地址;ax=0021h
bx = offset s2 ,即s2的偏移地址;bx=0026h
cx=cs,即s2的cs值 cx=076C
call指令执行时,会将下一条指令的IP入栈
call word ptr ds:[0],将其下一条指令pop ax的IP入栈,然后跳转。所以pop ax中ax保存的是s1的IP
call dword ptr ds:[0] 将其下一条指令的cs和IP同时入栈,然后进行跳转。所以pop bx中bx保存的是s2的IP,
pop cx中cx保存的是s2的cs值
问题②
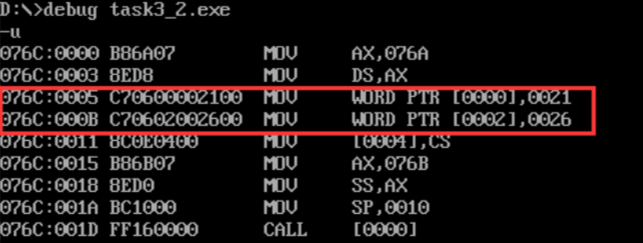
实验任务3
assume cs:code, ds:data data segment x db 99, 72, 85, 63, 89, 97, 55 len equ $ - x data ends code segment start: mov ax,data mov ds,ax mov byte ptr ds:[len],10 mov cx,7 mov bx,0 s: mov al,ds:[bx] mov ah,0 inc bx call printNumber call printSpace loop s mov ah,4ch int 21h printNumber: div byte ptr ds:[len] mov dx,ax mov ah,2 or dl,30h int 21h mov ah,2 mov dl,dh or dl,30h int 21h ret printSpace: mov dl,' ' mov ah,2 int 21h ret code ends end start
运行测试截图
实验任务4
assume cs:code,ds:data data segment str db 'try' len equ $ - str data ends code segment start: mov ax, data mov ds, ax mov bl, 2 mov bh, 0 call printStr mov bl, 4 mov bh, 24 call printStr mov ax, 4c00h int 21h printStr: mov ax, 0b800h mov es, ax mov si, offset str mov cx, len mov al,0A0h mul bh mov di, ax s: mov al,[si] mov es:[di], al mov es:[di+1], bl inc si add di, 2 loop s ret code ends end start
运行测试截图:
实验任务5
assume ds:data, cs:code data segment stu_no db '201983290260' len = $ - stu_no data ends code segment start: mov ax, data mov ds, ax mov cx, 4000 mov si, offset stu_no mov ax, 0b800h mov es, ax mov di, 0 mov ah,17h s: mov al, 0 mov es:[di], al mov es:[di+1], ah inc si add di, 2 loop s mov di, 3840 mov si, offset stu_no mov cx, 74 mov ah, 17h s1: call printgang add di, 2 loop s1 mov di, 3908 mov si, offset stu_no mov cx, len mov ah, 17h s2: call printStu_no inc si add di, 2 loop s2 mov di, 3932 mov si, offset stu_no mov cx, 74 mov ah, 17h s3: call printgang add di, 2 loop s3 mov ax, 4c00h int 21h printStu_no:mov al, [si] mov es:[di], al mov es:[di+1], ah ret printgang:mov al, 45 mov es:[di], al mov es:[di + 1], ah ret code ends end start
运行结果截图: