Leetcode——2. 两数相加
难度: 中等
题目
You are given two non-empty linked lists representing two non-negative integers. The digits are stored in reverse order and each of their nodes contain a single digit. Add the two numbers and return it as a linked list.
You may assume the two numbers do not contain any leading zero, except the number 0 itself.
给出两个 非空
的链表用来表示两个非负的整数。其中,它们各自的位数是按照 逆序
的方式存储的,并且它们的每个节点只能存储 一位 数字。
如果,我们将这两个数相加起来,则会返回一个新的链表来表示它们的和。
您可以假设除了数字 0 之外,这两个数都不会以 0 开头。
示例:
输入:(2 -> 4 -> 3) + (5 -> 6 -> 4)
输出:7 -> 0 -> 8
原因:342 + 465 = 807
PHP
这个题目相当于是两个链表进行相加,把链表每一节点的值存到新链表对应的节点,涉及到进位。
/**
* Definition for a singly-linked list.
* class ListNode {
* public $val = 0;
* public $next = null;
* function __construct($val) { $this->val = $val; }
* }
*/
class Solution {
/**
* @param ListNode $l1
* @param ListNode $l2
* @return ListNode
*/
function addTwoNumbers($l1, $l2) {
$add_flag = 0;
$result_list = new ListNode(0);
$curr = $result_list; //指向当前链表
do {
$val = $l1->val + $l2->val + $add_flag;
if ($val >= 10) {
$add_flag = 1;
$val -= 10; //减去,因为进位了
} else {
$add_flag = 0;
}
$tmp_list = new ListNode($val);
$curr->next = $tmp_list;
$curr = $curr->next;
$l1 = $l1->next;
$l2 = $l2->next;
} while ($l1 || $l2 || $add_flag);
return $result_list->next;
}
}
运行结果:
PHP链表打印出来的结构:
ListNode Object
(
[val] => 0
[next] => ListNode Object
(
[val] => 7
[next] => ListNode Object
(
[val] => 0
[next] => ListNode Object
(
[val] => 8
[next] =>
)
)
)
)
来源
链接:https://leetcode-cn.com/problems/add-two-numbers/
参考
1、PHP 链表的使用 - 简书
https://www.jianshu.com/p/e409ec512caa
2、2. Add Two Numbers · leetcode
https://leetcode.wang/leetCode-2-Add-Two-Numbers.html
本文优先在公众号"飞鸿影的博客(fhyblog)"发布,欢迎关注公众号及时获取最新文章推送!

作者:飞鸿影
出处:http://52fhy.cnblogs.com/
版权申明:没有标明转载或特殊申明均为作者原创。本文采用以下协议进行授权,自由转载 - 非商用 - 非衍生 - 保持署名 | Creative Commons BY-NC-ND 3.0,转载请注明作者及出处。
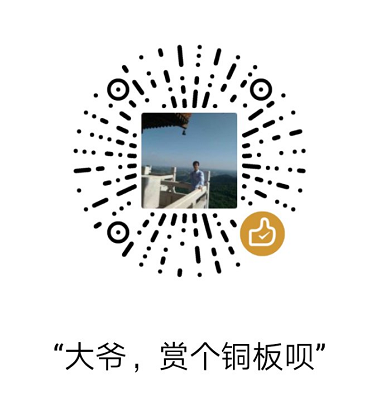