LeetCode——Letter Combinations of a Phone Number
Given a digit string, return all possible letter combinations that the number could represent.
A mapping of digit to letters (just like on the telephone buttons) is given below.
Input: Digit string "23"
Output: ["ad", "ae", "af", "bd", "be", "bf", "cd", "ce", "cf"].
Note:
Although the above answer is in lexicographical order, your answer could be in any order you want.
解题思路:
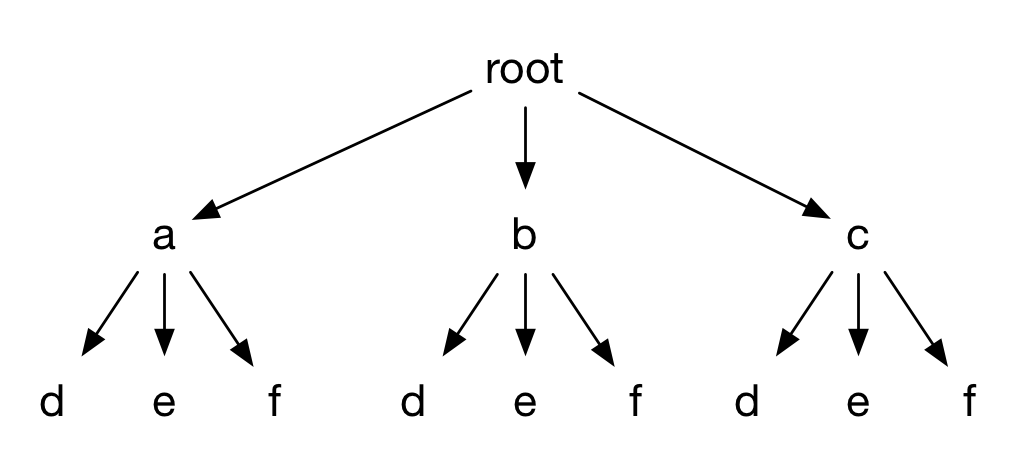
心想要是我们可以得到这样一棵树,那么我们的问题就变得很简单了,只需要利用DFS遍历一遍问题就解决了。
当然不建立这棵树,也是可以进行DFS遍历的。
#include <iostream>
#include <map>
#include <vector>
using namespace std;
class Solution {
public:
vector<string> letterCombinations(string digits) {
if (digits.size() == 0)
return result;
init();
dfs(0, (int)digits.size(), digits, "");
return result;
}
private:
vector<string> result;
map<char, string> dict;
void init() {
dict['0'] = " ";
dict['1'] = "";
dict['2'] = "abc";
dict['3'] = "def";
dict['4'] = "ghi";
dict['5'] = "jkl";
dict['6'] = "mno";
dict['7'] = "pqrs";
dict['8'] = "tuv";
dict['9'] = "wxyz";
}
void dfs(int dep, int max_dep, string digits, string tmp) {
if (dep == max_dep) {
result.push_back(tmp);
return;
}
//for循环遍历每个数字所对应的所有字符的情况,dfs递归是不断的深入
for (int i = 0; i < dict[digits[dep]].size(); i++) {
dfs(dep + 1, max_dep, digits, tmp + dict[digits[dep]][i]);
}
}
};
int main() {
string input = "23";
Solution* solution = new Solution();
vector<string> result = solution->letterCombinations(input);
for (int i = 0; i < result.size(); i++) {
cout << result[i] << endl;
}
return 0;
}