day1
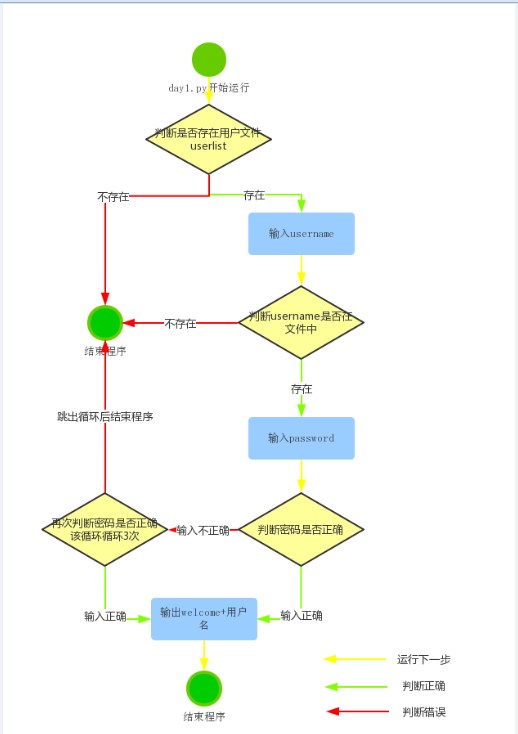
day1.py
#!/usr/bin/env python3
# -*- coding: utf-8 -*-
#user:Felix
#date:2016-10-11
#mail:wudonghang@wudonghang.com
#If you have questions or suggestions, please contact the author.
import getpass
import os
import json
#退出程序用,后续会重复调用。
def end():
print('\nProgram is over!')
exit()
#判断是否有用户文件
def file_exists():
if os.path.exists(userlist):
pass
else:
print('User list file not defined!')
end() #没有检测到用户文件,
#判断用户是否存在文件中
def user_exists():
if username not in userlist_message:
print('User name does not exist in the system!')
end()
#开始登陆流程
userlist = 'userlist'
file_exists()
username = input("Please input your username:")
with open(userlist, 'r') as userlist_json: #将文件内容读取出来
userlist_message = json.load(userlist_json) #转换json
user_exists()
#判断用户名密码是否正确,输入正确显示welcome+用户名,输入不正确3次后将跳出循环并锁定该用户。
real_password = userlist_message[username][0]['password'] #根据用户输入username取出json中的password
if int(userlist_message[username][0]['lock']) == 0:
print('Your user has been locked, please contact the administrator to unlock.!')
end()
elif int(userlist_message[username][0]['lock']) == 1:
pass
else:
print('Your user status is abnormal, please contact the administrator!')
end()
password = getpass.getpass(prompt='please input your password: ') #输入密码
for count in range(3):
if username == username in userlist_message and password == real_password:
print("Welcome! %s"%username)
end()
elif count == 2:
print('Sorry, your user name has been locked, temporarily not allowed to use!')
userlist_message[username][0]['lock'] = 0
json.dump(userlist_message, open(userlist, 'w'))
break #输错次数到3次跳出循环,并加入黑名单
else:
print("wrong username or password!") #提示密码输入错误
password = getpass.getpass(prompt='Please enter your password again: ') #重新输入一次密码
end()
userlist:
{"huangxu": [{"password": "huangxu123", "lock": 1}], "liuyao": [{"password": "liuyao123", "lock": 1}], "wudonghang": [{"password": "wudonghang123", "lock": 1}], "administrator": [{"password": "administrator123", "lock": 1}]}
readme:
###博客园地址:[Python之路,Day1 - Python基础1](http://www.cnblogs.com/wudonghang/p/d40bdbbd1a87e6c907ac76f8c6f4c024.html "我的博客园地址")
***
###程序简介:
>背景
[作业:](http://www.cnblogs.com/alex3714/articles/5465198.html#3519330 "大王留下的作业")
* 编写登陆接口
* 输入用户名密码
* 认证成功后显示欢迎信息
* 输错三次后锁定
***
>构想流程图

***
>实现方式
1. 用户数据文件形式采用了json形式 (lock值为0时,用户为锁定状态)
json格式如下
{
"wudonghang": [
{
"password": "wudonghang123",
"lock": 0
}
],
"administrator": [
{
"password": "administrator123",
"lock": 1
}
]
}
2. 查询json文件数据方法
with open(userlist, 'r') as userlist_json:
userlist_message = json.load(userlist_json)
real_password = userlist_message[username][0]['password'] #查询文件中password
real_lock = userlist_message[username][0]['lock'] #查询用户锁定状态
3. 采集username以及password方法
input("Please input your username:") #输入用户名
getpass.getpass(prompt='Please enter your password again: ') #这里我采用了getpass模块
4. 利用for循环与if判断来实现对username和password的判断,以及用户lock值的修改
for count in range(3):
if username == username in userlist_message and password == real_password:
print("Welcome! %s"%username)
end() #登陆成功跳出程序
elif count == 2:
print('Sorry, your user name has been locked, temporarily not allowed to use!')
userlist_message[username][0]['lock'] = 0
json.dump(userlist_message, open(userlist, 'w'))
break #输错次数到3次跳出循环,并加入黑名单
else:
print("wrong username or password!") #提示密码输入错误
password = getpass.getpass(prompt='Please enter your password again: ') #重新输入一次密码
***
user:Felix
mail:wudonghang@wudonghang.com
If you have questions or suggestions, please contact the author.