不知道大家看没看过《ASP.NET2.0开发指南》这本书。不管这本书怎么样(看到网上骂声不断),对于我这种菜鸟来说还是有些帮助的。既然花了钱买了书,肯定得学点东西才行。下面我就把个人学习ObjectDataSource的一些心得跟大家分享一下。
这里说是使用ObjectDataSource,其实更多的,是要了解三层(N层)架构方面的知识。首先我们可以根据下面的图例,建立三个项目:
1、WEB站点 用户界面层
2、AuthorDB 数据访问层
3、LogicLibrary 业务逻辑层
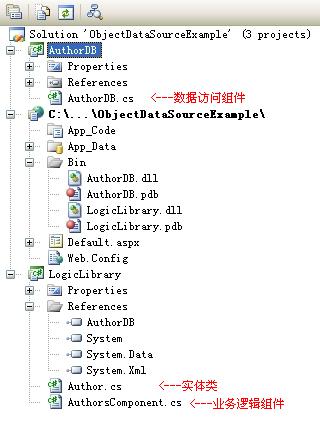
接下来,我们分别实现这三层。
数据访问层的实现:
这里用了一个组件AuthorDB.cs来完成对数据库的操作,具体代码如下
1
using System;
2
using System.Collections.Generic;
3
using System.Text;
4
using System.Data;
5
using System.Data.OleDb;
6
using System.Xml;
7
8
namespace AuthorDB
9

{
10
public class AuthorDB
11
{
12
//数据库连接字符串
13
static string str = System.Configuration.ConfigurationSettings.AppSettings["ConnStrings"].ToString().Trim();
14
//构造函数
15
public AuthorDB()
16
{ }
17
//获取地址
18
public static DataSet GetStates()
19
{
20
string strCmd = "select distinct state from authors";
21
return DataAdapterFill(strCmd,"state");
22
}
23
//通过地址获取信息
24
public static DataSet GetAuthorsByState(string state)
25
{
26
string strCmd = "select * from authors where state='"+state.ToString().Trim()+"'";
27
return DataAdapterFill(strCmd,"authors");
28
29
}
30
//修改信息
31
public static int UpdateAuthor(string au_id,string au_fname,string au_lname,string state)
32
{
33
string strCmd = "update authors set firstname='"+au_fname+"',lastname='"+au_lname+"',state='"+state+"' where id='"+au_id+"'";
34
return DataCommand(strCmd);
35
}
36
/**//// <summary>
37
/// DataAdapterFill
38
/// </summary>
39
/// <param name="strCmd">SQL语句</param>
40
/// <param name="tablename">表名</param>
41
/// <returns>数据集</returns>
42
private static DataSet DataAdapterFill(string strCmd, string tablename)
43
{
44
OleDbConnection conn = new OleDbConnection(str);
45
OleDbDataAdapter da = new OleDbDataAdapter(strCmd, conn);
46
DataSet ds = new DataSet();
47
conn.Open();
48
da.Fill(ds, tablename);
49
conn.Close();
50
return ds;
51
}
52
/**//// <summary>
53
/// DataCommand方法
54
/// </summary>
55
/// <param name="strCmd">SQL语句</param>
56
/// <returns>影响行数</returns>
57
private static int DataCommand(string strCmd)
58
{
59
OleDbConnection conn = new OleDbConnection(str);
60
OleDbCommand cmd = new OleDbCommand(strCmd, conn);
61
int rowsAffected = 0;
62
conn.Open();
63
try
64
{
65
rowsAffected = cmd.ExecuteNonQuery();
66
}
67
catch
68
{ }
69
finally
70
{
71
conn.Close();
72
}
73
return rowsAffected;
74
}
75
}
76
}
77
业务逻辑层的实现:[注:逻辑层要引用AuthorDB.dll]
业务逻辑层主要由业务实体Author.cs和逻辑组件AuthorsComponent.cs组成[为什么要使用业务实体/怎么使用业务实体这里暂时不做介绍,请不择手段自己搜索]
业务实体实现代码
1
using System;
2
using System.Collections.Generic;
3
using System.Text;
4
5
namespace LogicLibrary
6

{
7
public class Author
8
{
9
private string _id;
10
private string _firstname;
11
private string _lastname;
12
private string _state;
13
14
public Author()
15
{ }
16
public Author(string id,string firstname,string lastname,string state)
17
{
18
this.ID = id;
19
this.FirstName = firstname;
20
this.LastName = lastname;
21
this.State = state;
22
}
23
public string ID
24
{
25
get
26
{
27
return _id;
28
}
29
set
30
{
31
_id = value;
32
}
33
}
34
public string FirstName
35
{
36
get
37
{
38
return _firstname;
39
}
40
set
41
{
42
_firstname = value;
43
}
44
}
45
public string LastName
46
{
47
get
48
{
49
return _lastname;
50
}
51
set
52
{
53
_lastname = value;
54
}
55
}
56
public string State
57
{
58
get
59
{
60
return _state;
61
}
62
set
63
{
64
_state = value;
65
}
66
}
67
68
}
69
}
70
逻辑组件实现代码
1
using System;
2
using System.Collections.Generic;
3
using System.Text;
4
using System.Data;
5
6
namespace LogicLibrary
7

{
8
public class AuthorsComponent
9
{
10
//构造函数
11
public AuthorsComponent()
12
{ }
13
//通过地址获取信息
14
public List<Author> GetAuthorsByState(string state, string sortExpression)
15
{
16
List<Author> authors = new List<Author>();
17
DataSet ds = AuthorDB.AuthorDB.GetAuthorsByState(state);
18
foreach (DataRow row in ds.Tables["authors"].Rows)
19
{
20
authors.Add(new Author((string)row["id"],(string)row["firstname"],(string)row["lastname"],(string)row["state"]));
21
}
22
authors.Sort(new AuthorComparer(sortExpression));
23
return authors;
24
}
25
//获取地址
26
public List<string> GetStates()
27
{
28
List<string> states = new List<string>();
29
DataSet ds = AuthorDB.AuthorDB.GetStates();
30
foreach (DataRow row in ds.Tables["state"].Rows)
31
states.Add((string)row["state"]);
32
return states;
33
}
34
//修改信息
35
public int UpdateAuthor(Author a)
36
{
37
return AuthorDB.AuthorDB.UpdateAuthor(a.ID,a.LastName,a.FirstName,a.State);
38
}
39
//修改信息
40
public int UpdateAuthor(string ID,string FirstName,string LastName,string State)
41
{
42
return AuthorDB.AuthorDB.UpdateAuthor(ID,FirstName,LastName,State);
43
}
44
}
45
//自定义排序 实现IComparer接口
46
public class AuthorComparer : IComparer<Author>
47
{
48
private string _sortColumn;
49
private bool _reverse;
50
//构造函数
51
public AuthorComparer(string sortExpression)
52
{
53
_reverse = sortExpression.ToLowerInvariant().EndsWith(" desc");
54
if (_reverse)
55
{
56
_sortColumn = sortExpression.Substring(0, sortExpression.Length - 5);
57
}
58
else
59
{
60
_sortColumn = sortExpression;
61
}
62
}
63
//实现接口的Compare方法 比较两个Author对象实例
64
public int Compare(Author a,Author b)
65
{
66
int retVal = 0;
67
switch (_sortColumn)
68
{
69
case "ID":
70
retVal = String.Compare(a.ID, b.ID, StringComparison.InvariantCultureIgnoreCase);
71
break;
72
case "FirstName":
73
retVal = String.Compare(a.FirstName, b.FirstName, StringComparison.InvariantCultureIgnoreCase);
74
break;
75
case "LastName":
76
retVal = String.Compare(a.LastName, b.LastName, StringComparison.InvariantCultureIgnoreCase);
77
break;
78
case "State":
79
retVal = String.Compare(a.State, b.State, StringComparison.InvariantCultureIgnoreCase);
80
break;
81
}
82
return (retVal*(_reverse?-1:1));
83
}
84
}
85
}
86
接下来我们要实现的是用户界面层:[注:界面层要引用LogicLibrary项目生成的dll文件]
用户界面层实现比较简单,这要归功于asp.net2.0中为我们提供的两个用户控件GridView和ObjectDataSource.[具体使用方法自己搜索]
我们先在页面上拖放1个DropDownList,2个ObjectDataSource,1个GridView控件。
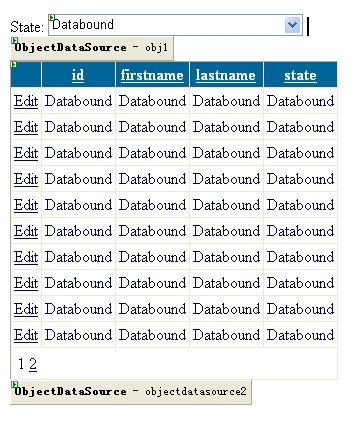
具体设置请参见如下页面代码[注:加粗部分要特别注意]
1
<%
@ Page Language="C#" AutoEventWireup="true" CodeFile="Default.aspx.cs" Inherits="_Default" %>
2
3
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
4
<html xmlns="http://www.w3.org/1999/xhtml">
5
<head runat="server">
6
<title>Untitled Page</title>
7
</head>
8
<body>
9
<form id="form1" runat="server">
10
<div>
11
State:
12
<asp:DropDownList ID="DropDownList1" runat="server" DataSourceID="obj1" AutoPostBack="True"
13
Width="255px">
14
</asp:DropDownList>
15
<asp:ObjectDataSource ID="obj1" runat="server" TypeName="LogicLibrary.AuthorsComponent"
16
SelectMethod="GetStates"></asp:ObjectDataSource>
17
<asp:GridView ID="gridview1" runat="server" DataSourceID="objectdatasource2" AllowPaging="True"
18
AllowSorting="True" AutoGenerateColumns="False" EnableTheming="True" BackColor="White"
19
BorderColor="#CCCCCC" BorderStyle="None" BorderWidth="1px" CellPadding="3" Width="308px">
20
<Columns>
21
<asp:CommandField ShowEditButton="True" CancelText="Cancle" />
22
<asp:BoundField DataField="id" HeaderText="id" SortExpression="ID" />
23
<asp:BoundField DataField="firstname" HeaderText="firstname" SortExpression="FristName" />
24
<asp:BoundField DataField="lastname" HeaderText="lastname" SortExpression="LastName" />
25
<asp:BoundField DataField="state" HeaderText="state" SortExpression="State" />
26
</Columns>
27
<FooterStyle BackColor="White" ForeColor="#000066" />
28
<RowStyle ForeColor="#000066" />
29
<SelectedRowStyle BackColor="#669999" Font-Bold="True" ForeColor="White" />
30
<PagerStyle BackColor="White" ForeColor="#000066" HorizontalAlign="Left" />
31
<HeaderStyle BackColor="#006699" Font-Bold="True" ForeColor="White" />
32
</asp:GridView>
33
<asp:ObjectDataSource ID="objectdatasource2" runat="server" TypeName="LogicLibrary.AuthorsComponent"
34
DataObjectTypeName="LogicLibrary.Author" SelectMethod="GetAuthorsByState" UpdateMethod="UpdateAuthor"
35
SortParameterName="sortExpression">
36
<SelectParameters>
37
<asp:ControlParameter ControlID="DropDownList1" Name="state" PropertyName="SelectedValue" />
38
</SelectParameters>
39
</asp:ObjectDataSource>
40
</div>
41
</form>
42
</body>
43
</html>
44
OK,一切都搞定了。以上代码已经在winxp+vs.net2005上测试通过。它可以实现数据的显示、筛选、排序功能。我们来看看运行结果:
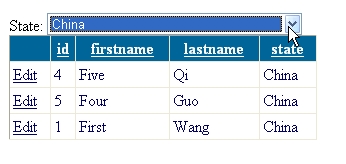
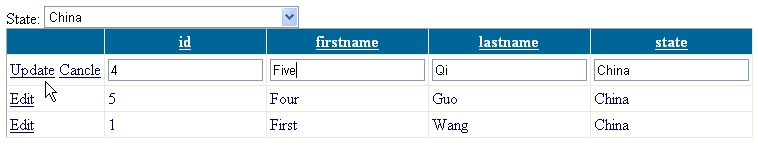
附:Access数据库的XML文件
1
<?xml version="1.0" encoding="UTF-8"?>
2
<root xmlns:xsd="http://www.w3.org/2001/XMLSchema" xmlns:od="urn:schemas-microsoft-com:officedata">
3
<xsd:schema>
4
<xsd:element name="dataroot">
5
<xsd:complexType>
6
<xsd:sequence>
7
<xsd:element ref="authors" minOccurs="0" maxOccurs="unbounded"/>
8
</xsd:sequence>
9
<xsd:attribute name="generated" type="xsd:dateTime"/>
10
</xsd:complexType>
11
</xsd:element>
12
<xsd:element name="authors">
13
<xsd:annotation>
14
<xsd:appinfo>
15
<od:index index-name="id" index-key="id " primary="no" unique="no" clustered="no"/>
16
</xsd:appinfo>
17
</xsd:annotation>
18
<xsd:complexType>
19
<xsd:sequence>
20
<xsd:element name="id" minOccurs="0" od:jetType="text" od:sqlSType="nvarchar">
21
<xsd:simpleType>
22
<xsd:restriction base="xsd:string">
23
<xsd:maxLength value="50"/>
24
</xsd:restriction>
25
</xsd:simpleType>
26
</xsd:element>
27
<xsd:element name="firstname" minOccurs="0" od:jetType="text" od:sqlSType="nvarchar">
28
<xsd:simpleType>
29
<xsd:restriction base="xsd:string">
30
<xsd:maxLength value="50"/>
31
</xsd:restriction>
32
</xsd:simpleType>
33
</xsd:element>
34
<xsd:element name="lastname" minOccurs="0" od:jetType="text" od:sqlSType="nvarchar">
35
<xsd:simpleType>
36
<xsd:restriction base="xsd:string">
37
<xsd:maxLength value="50"/>
38
</xsd:restriction>
39
</xsd:simpleType>
40
</xsd:element>
41
<xsd:element name="state" minOccurs="0" od:jetType="text" od:sqlSType="nvarchar">
42
<xsd:simpleType>
43
<xsd:restriction base="xsd:string">
44
<xsd:maxLength value="50"/>
45
</xsd:restriction>
46
</xsd:simpleType>
47
</xsd:element>
48
</xsd:sequence>
49
</xsd:complexType>
50
</xsd:element>
51
</xsd:schema>
52
<dataroot xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" generated="2007-04-16T15:32:00">
53
<authors>
54
<id>1</id>
55
<firstname>Wang</firstname>
56
<lastname>First</lastname>
57
<state>usa</state>
58
</authors>
59
<authors>
60
<id>2</id>
61
<firstname>Second</firstname>
62
<lastname>Li</lastname>
63
<state>Japan</state>
64
</authors>
65
<authors>
66
<id>3</id>
67
<firstname>Third</firstname>
68
<lastname>Zhao</lastname>
69
<state>USA</state>
70
</authors>
71
<authors>
72
<id>5</id>
73
<firstname>Four</firstname>
74
<lastname>Guo</lastname>
75
<state>China</state>
76
</authors>
77
<authors>
78
<id>4</id>
79
<firstname>Five</firstname>
80
<lastname>Qi</lastname>
81
<state>China</state>
82
</authors>
83
</dataroot>
84
</root>
85