Exception Management in .NET阅读笔记
Exception Management in .NET阅读笔记
今天阅读了《Exception Management in .NET》一文,虽然是老文章了,但是其中的一些理念不但正确而且非常有价值。我比较懒,直接把要点复制出来了,呵呵。
异常管理
Ø An exception management system should be well encapsulated and should abstract the details of logging and reporting from the application’s business logic.
Ø Exceptions represent a breach of an implicit assumption made within code.
Ø Exceptions are not necessarily errors.
Ø You should derive your custom application exceptions from ApplicationException.
异常检测
You should only catch exceptions when you need to specifically perform any of the
following actions:
Ø Gather information for logging
Ø Add any relevant information to the exception
Ø Execute cleanup code
Ø Attempt to recover
适当使用异常
Throwing exceptions is more expensive than simply returning a result to a caller. Therefore they should not be used to control the normal flow of execution through your code. In addition, excessive use of exceptions can create unreadable and unmanageable code.
异常传播
There are three main ways to propagate exceptions:
Ø Let the exception propagate automatically:With this approach, you do nothing and deliberately ignore the exception.
Ø Catch and rethrow the exception:With this approach, you catch and react to the exception, and clean up or perform any other required processing within the scope of the current method. If you cannot recover from the exception, you rethrow the same exception to your caller.
catch(TypeAException e) { // Code to do any processing needed. // Rethrow the exception throw; } |
Ø Catch, wrap, and throw the wrapped exception:If you cannot recover, wrap the exception in a new exception, and throw the new exception back to the caller. The InnerException property of the Exception class explicitly allows you to preserve a previously caught exception. This allows the original exception to be wrapped as an inner exception inside a new and more relevant outer exception.
catch(TypeBException e) { // Code to do any processing needed. // Wrap the current exception in a more relevant // outer exception and rethrow the new exception. throw(new TypeCException(strMessage, e)); } |
Note:As the exception propagates up the call stack, catch blocks can only catch the outer exception. Inner exceptions are programmatically accessible through the InnerException property, but they cannot be matched to a catch block.
什么时候使用内部异常
For example, consider a hypothetical method called LoadUserInfo. This method may load a user’s information from a file that is assumed to exist when the method Exception Management in .NET 9 tries to access it. If the file does not exist, a FileNotFoundException is thrown,which has meaning within the context of the LoadUserInfo method. However, as the exception propagates back up the call stack—for example, to a LogonUser method—a FileNotFoundException exception does not provide any valuable information. If you wrap the FileNotFoundException in a custom exception class (discussed later in this document)—for example, one called FailedToLoadUserInfoException—and throw the wrapper exception, it provides more information and is more relevant to the calling LogonUser method. You can then catch the FailedToLoadUserInfoException exception in the LogonUser method and react to that particular exception type rather than having to catch the FileNotFoundException, which is an implementation detail of another method.
自定义异常
This hierarchy allows your application to benefit from the following:
Ø Easier development because you can define properties and methods on your base application exception class that can be inherited by your other application exception classes.
Ø New exception classes created after your application has been deployed can derive from an existing exception type within the hierarchy. Existing exception handling code that catches one of the base classes of the new exception object will catch the new exception without any modifications to the existing code, interpreting it as one of the object’s base classes.
You should only create a new application exception class for an exception type that you need to react to and handle within your code that is not already available in your application exception hierarchy or in the Framework.
管理未处理异常
Ø You should configure exception management settings within your application’s Web.config file.
<customErrors defaultredirect="http://hostname/error.aspx" mode="on"> <error statuscode="500" redirect="/errorpages/servererror.aspx" /> <error statuscode="404" redirect="/errorpages/filenotfound.htm" /> </customErrors> |
Ø You should be aware that these settings only apply to ASP.NET files (that is, files with specific extensions, such as .aspx and .asmx).
Ø Page_Error:Page.Error += new System.EventHandler(Page_Error);
Ø Application_Error:
protected void Application_Error(Object sender, EventArgs e) { Exception exc = Server.GetLastError(); // Perform logging, send any notifications, etc. } |
补充一个,对于网站程序,我们是使用HttpModule来处理未处理异常的,非常方便。
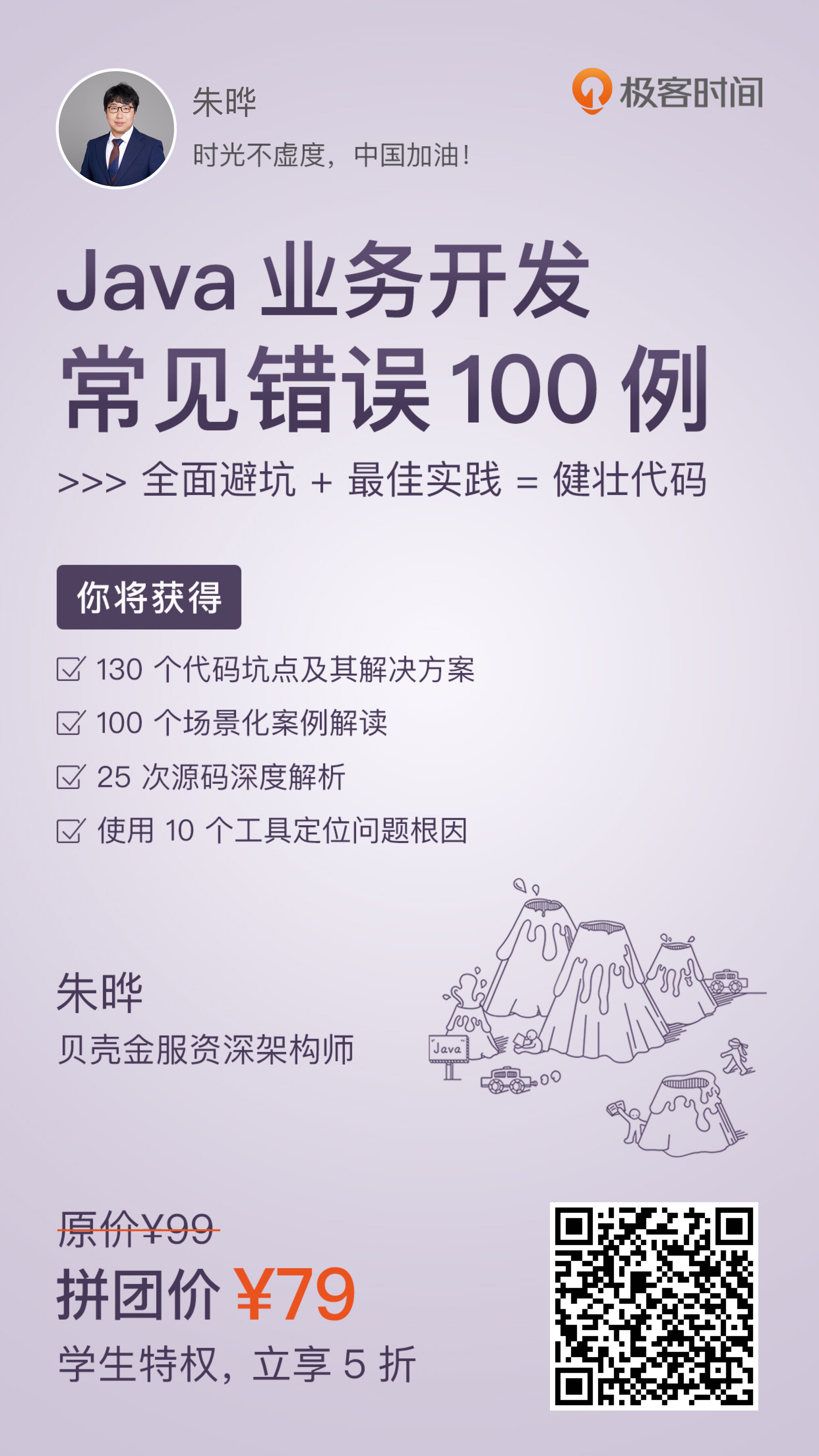