Java学习-013-文本文件读取实例源代码(两种数据返回格式)
此文源码主要为应用 Java 读取文本文件内容实例的源代码。若有不足之处,敬请大神指正,不胜感激!
1.读取的文本文件内容以一维数组【LinkedList<String>】的形式返回,源代码如下所示:

1 /** 2 * @function 文本文件操作:读取数据 3 * 4 * @author Aaron.ffp 5 * @version V1.0.0: autoUISelenium main.java.aaron.java.tools FileUtils.java txtRead, 2015-2-2 21:03:46 Exp $ 6 * 7 * @param filename :文本文件全路径 8 * @param encodingType :文本文件编码 9 * 10 * @return LinkedList<String> 文本文件内容 11 */ 12 public LinkedList<String> txtRead(String filename, String encodingType){ 13 LinkedList<String> fileContent = new LinkedList<String>(); 14 15 try{ 16 File f = new File(filename); 17 18 if(f.isFile() && f.exists()){ 19 InputStreamReader read = new InputStreamReader(new FileInputStream(f), encodingType); 20 BufferedReader br = new BufferedReader(read); 21 22 String rowContent; 23 24 while((rowContent = br.readLine()) != null){ 25 fileContent.add(rowContent); 26 } 27 28 if(br != null){ 29 br.close(); 30 } 31 32 if(read != null){ 33 read.close(); 34 } 35 }else{ 36 this.message = "{" + filename + "}为目录或者文件不存在,或文件正在被占用!"; 37 this.logger.error(this.message); 38 } 39 }catch(Exception ioe){ 40 this.message = "读取文件 {" + filename + "}内容出错。" + ioe.getMessage(); 41 this.logger.error(this.message); 42 } 43 44 return fileContent; 45 }
测试:返回一维数组,源代码如下所示:

1 /** 2 * 测试:FileUtils.txtRead(String, String) 3 * 4 * @author Aaron.ffp 5 * @version V1.0.0: autoUISelenium test.java.aaron.java.tools FileUtilsTest.java txtRead, 2015-2-2 22:07:14 Exp $ 6 * 7 */ 8 @Test 9 public void txtRead_row() { 10 this.fu = new FileUtils(); 11 this.message = "\n\n\n测试:FileUtils.txtRead(String, String)"; 12 this.logger.debug(this.message); 13 14 try{ 15 this.filename = this.constantslist.PARAFILEPATH.get("TEST") + "source-txtRead.txt"; 16 this.message = "测试文本文件源为:" + this.filename; 17 this.logger.debug(this.message); 18 19 LinkedList<String> contentlist = this.fu.txtRead(this.filename, "GB2312"); 20 String fileContent = ""; // 文件内容 21 22 if(contentlist.size() > 0){ 23 for(int rowsCount = 1; rowsCount <= contentlist.size()/1000 + 1; rowsCount++){ 24 for(int i = 0; i < contentlist.size(); i++){ 25 fileContent = contentlist.get(i); 26 27 this.logger.debug(fileContent); 28 29 if (i == 5) { 30 return; 31 } 32 } 33 } 34 } 35 }catch(Exception ioe){ 36 ioe.printStackTrace(); 37 this.message = ioe.getMessage(); 38 this.logger.error(this.message); 39 } 40 }
2.读取的文本文件内容以二维数据【LinkedList<String[]>】的形式返回(删除了数据左右空格),源代码如下所示:

1 /** 2 * @function 文本文件操作:读取数据 3 * 4 * @author Aaron.ffp 5 * @version V1.0.0: autoUISelenium main.java.aaron.java.tools FileUtils.java txtRead, 2015-2-2 21:03:46 Exp $ 6 * 7 * @param filename :文本文件全路径 8 * @param encodingType :文本文件编码 9 * @param split : 行分隔符 10 * 11 * @return LinkedList<String[]> 文本文件内容 12 */ 13 public LinkedList<String[]> txtRead(String filename, String encodingType, String split){ 14 LinkedList<String[]> fileContent = new LinkedList<String[]>(); 15 16 try{ 17 File f = new File(filename); 18 19 if(f.isFile() && f.exists()){ 20 InputStreamReader read = new InputStreamReader(new FileInputStream(f), encodingType); 21 BufferedReader br = new BufferedReader(read); 22 23 String rowContent; 24 25 while((rowContent = br.readLine()) != null){ 26 String[] content = rowContent.split(split); 27 String[] rowdata = new String[content.length]; 28 29 for (int i = 0; i < content.length; i++) { 30 rowdata[i] = content[i].toString().trim(); 31 } 32 33 fileContent.add(rowdata); 34 } 35 36 if(br != null){ 37 br.close(); 38 } 39 40 if(read != null){ 41 read.close(); 42 } 43 }else{ 44 this.message = "{" + filename + "}为目录或者文件不存在,或文件正在被占用!"; 45 this.logger.error(this.message); 46 } 47 }catch(Exception ioe){ 48 this.message = "读取文件 {" + filename + "}内容出错。" + ioe.getMessage(); 49 this.logger.error(this.message); 50 } 51 52 return fileContent; 53 }
测试:返回二维数组,源代码如下所示:

1 /** 2 * 测试:FileUtils.txtRead(String, String, String) 3 * 4 * @author Aaron.ffp 5 * @version V1.0.0: autoUISelenium test.java.aaron.java.tools FileUtilsTest.java txtRead, 2015-2-2 22:17:14 Exp $ 6 * 7 */ 8 @Test 9 public void txtRead_rowcontent() { 10 this.fu = new FileUtils(); 11 this.message = "\n\n\n测试:FileUtils.txtRead(String, String, String)"; 12 this.logger.debug(this.message); 13 14 try{ 15 this.filename = this.constantslist.PARAFILEPATH.get("TEST") + "source-txtRead.txt"; 16 this.message = "测试文本文件源为:" + this.filename; 17 this.logger.debug(this.message); 18 19 LinkedList<String[]> fdata = this.fu.txtRead(this.filename, "GB2312", "\\|"); 20 21 if(fdata.size() > 0){ 22 for(int rowsCount = 1; rowsCount <= 5; rowsCount++){ 23 String[] arr_row = fdata.get(rowsCount); 24 String rowdata = ""; 25 26 for (int i = 0; i < arr_row.length; i++) { 27 rowdata += arr_row[i] + "\t"; 28 } 29 30 this.logger.debug(rowdata); 31 } 32 } 33 }catch(Exception ioe){ 34 ioe.printStackTrace(); 35 this.message = ioe.getMessage(); 36 this.logger.error(this.message); 37 } 38 }
3.文本文件内容如下所示:

1 01|20130131| | | 48104| | | | | 2 READ00|长安|1|000001| 18653471415| 11240| 10000|1|1|1 3 READ04|哈佛|1|000001| 1150000000| 7650| 10000|1|1|1 4 READ05|武钢|1|000001| 10093779823| 2990| 10000|1|1|1 5 READ06|东风|1|000001| 2000000000| 3250| 10000|1|1|1 6 READ07|现代|1|000001| 1007282534| 11540| 10000|1|1|1 7 READ08|本田|1|000001| 2200000000| 4500| 10000|1|1|1 8 READ09|猎豹|1|000001| 1926958448| 13210| 10000|1|1|1 9 READ10|陆风|1|000001| 6423643659| 5250| 10000|1|1|1 10 READ11|东风|1|000001| 10500000000| 6380| 10000|1|1|1 11 READ12|华晨|1|000001| 1165READ00| 4200| 10000|1|1|1 12 READ15|昨天|1|000001| 6849725776| 11540| 10000|1|1|1 13 READ16|今天|1|000001| 22587602387| 10360| 10000|1|1|1 14 READ17|山东|1|000001| 3075653888| 2950| 10000|1|1|1 15 READ18|上海|1|000001| 22755179650| 2990| 10000|1|1|1 16 READ19|北京|1|000001| 17122048088| 5030| 10000|1|1|1 17 READ20|天津|1|000001| 2247371832| 2520| 10000|1|1|1 18 READ21|南京|1|000001| 2139739257| 4720| 10000|1|1|1 19 READ22|江苏|1|000001| 6436295797| 2250| 10000|1|1|1 20 READ26|河北|1|000001| 2108552613| 4770| 10000|1|1|1 21 READ27|海南|1|000001| 5940056200| 3820| 10000|1|1|1 22 READ28|丽江|1|000001| 70039798886| 7110| 10000|1|1|1 23 READ29|天界|1|000001| 7022650000| 4130| 10000|1|1|1
脚本运行结果:
至此, Java学习-013-文本文件读取实例源代码(两种数据返回格式) 顺利完结,希望此文能够给初学 Java 的您一份参考。
最后,非常感谢亲的驻足,希望此文能对亲有所帮助。热烈欢迎亲一起探讨,共同进步。非常感谢! ^_^
欢迎 【 留言 || 关注 || 打赏 】 。您的每一份心意都是对我的鼓励和支持!非常感谢!欢迎互加,相互交流学习!
作者:范丰平,本文链接:https://www.cnblogs.com/fengpingfan/p/4326342.html
Copyright @范丰平 版权所有,如需转载请标明本文原始链接出处,严禁商业用途! 我的个人博客链接地址:http://www.cnblogs.com/fengpingfan
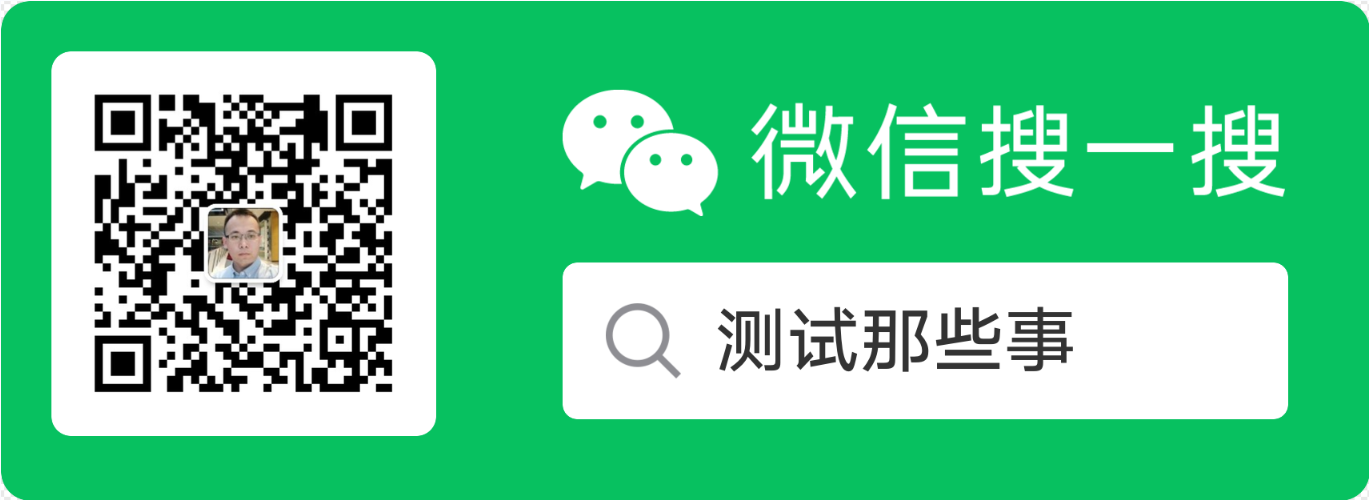