IGPMatrix 的属性与方法:
//方法 IGPMatrix.Reset; { 重置 Matrix 得到单位矩阵(斜对角线是1其他是0) } IGPMatrix.Translate(); { 平移 } IGPMatrix.Scale(); { 放缩 } IGPMatrix.Rotate(); { 旋转(沿(0,0)点, 顺时针) } IGPMatrix.RotateAt(); { 沿指定点, 顺时针旋转 } IGPMatrix.Shear(); { 剪切(或叫错切、斜切) } IGPMatrix.Invert; { 反转 } IGPMatrix.Multiply(); { 与指定的 Matrix 相乘 } IGPMatrix.TransformPoint(); { 对指定点应用此 Matrix 变换 } IGPMatrix.TransformPoints(); { 对点数组应用此 Matrix 变换 } IGPMatrix.TransformVector(); { 对指定点应用此 Matrix 变换中的缩放和旋转变换 } IGPMatrix.TransformVectors(); { 对点数组应用此 Matrix 变换中的缩放和旋转变换 } IGPMatrix.SetElements(); { 设置 Matrix 各元素 } IGPMatrix.Clone; { 克隆副本 } IGPMatrix.Equals(); { 判断是否相等 } //属性 IGPMatrix.Elements; { 获取 Matrix 各元素构成的数组 } IGPMatrix.OffsetX; { 获取 Matrix.Elements.DX } IGPMatrix.OffsetY; { 获取 Matrix.Elements.DY } IGPMatrix.IsInvertible; { 判断 Matrix 是否可逆转 } IGPMatrix.IsIdentity; { 判断 Matrix 是否是单位矩阵(斜对角线是1其他是0) }
下面的例子用到了其中的 Reset、Translate、Scale、Rotate、Shear、Invert 方法:
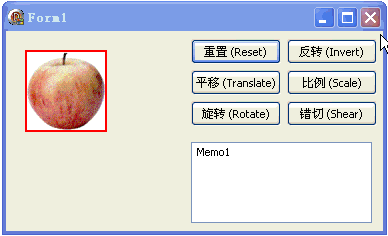
代码:
unit Unit1; interface uses Windows, Messages, SysUtils, Variants, Classes, Graphics, Controls, Forms, Dialogs, StdCtrls; type TForm1 = class(TForm) Button1: TButton; Button2: TButton; Button3: TButton; Button4: TButton; Button5: TButton; Button6: TButton; Memo1: TMemo; procedure FormCreate(Sender: TObject); procedure FormPaint(Sender: TObject); procedure Button1Click(Sender: TObject); procedure Button2Click(Sender: TObject); procedure Button3Click(Sender: TObject); procedure Button4Click(Sender: TObject); procedure Button5Click(Sender: TObject); procedure Button6Click(Sender: TObject); end; var Form1: TForm1; implementation {$R *.dfm} uses GdiPlus; var Matrix: IGPMatrix; //用字符串的形式返回 Matrix 数据, 用于查看 function ViewMatrix(Matrix: IGPMatrix): string; const fmt = '%f'#9'%f'#9'0'#13#10#13#10'%f'#9'%f'#9'0'#13#10#13#10'%f'#9'%f'#9'1'; begin Result := Format(fmt, [Matrix.Elements.M11, Matrix.Elements.M12, Matrix.Elements.M21, Matrix.Elements.M22, Matrix.Elements.DX, Matrix.Elements.DY]); end; //初识化 procedure TForm1.FormCreate(Sender: TObject); begin Button1.Caption := '重置 (Reset)'; Button2.Caption := '反转 (Invert)'; Button3.Caption := '平移 (Translate)'; Button4.Caption := '比例 (Scale)'; Button5.Caption := '旋转 (Rotate)'; Button6.Caption := '错切 (Shear)'; Matrix := TGPMatrix.Create; end; //绘图 procedure TForm1.FormPaint(Sender: TObject); var Image: IGPImage; Graphics: IGPGraphics; Rect: TGPRect; begin Image := TGPImage.Create('C:\GdiPlusImg\Apple.gif'); Rect.Initialize(20, 20, 80, 80); Graphics := TGPGraphics.Create(Handle); Graphics.MultiplyTransform(Matrix); Graphics.DrawImage(Image, Rect); Graphics.DrawRectangle(TGPPen.Create($FFFF0000, 2), Rect); end; //重置到单位矩阵 procedure TForm1.Button1Click(Sender: TObject); begin Matrix.Reset; Memo1.Text := ViewMatrix(Matrix); Repaint; end; //反转变换 procedure TForm1.Button2Click(Sender: TObject); begin Matrix.Invert; Memo1.Text := ViewMatrix(Matrix); Repaint; end; //平移变换 procedure TForm1.Button3Click(Sender: TObject); begin Matrix.Translate(1.5, 3); Memo1.Text := ViewMatrix(Matrix); Repaint; end; //比例变换 procedure TForm1.Button4Click(Sender: TObject); begin Matrix.Scale(0.75, 1.5); Memo1.Text := ViewMatrix(Matrix); Repaint; end; //旋转变换 procedure TForm1.Button5Click(Sender: TObject); begin Matrix.Rotate(15); Memo1.Text := ViewMatrix(Matrix); Repaint; end; //错切变换 procedure TForm1.Button6Click(Sender: TObject); begin Matrix.Shear(0.2, 0.5); Memo1.Text := ViewMatrix(Matrix); Repaint; end; end.
窗体:
object Form1: TForm1 Left = 0 Top = 0 Caption = 'Form1' ClientHeight = 200 ClientWidth = 377 Color = clBtnFace Font.Charset = DEFAULT_CHARSET Font.Color = clWindowText Font.Height = -11 Font.Name = 'Tahoma' Font.Style = [] OldCreateOrder = False OnCreate = FormCreate OnPaint = FormPaint PixelsPerInch = 96 TextHeight = 13 object Button1: TButton Left = 185 Top = 8 Width = 90 Height = 25 Caption = 'Button1' TabOrder = 0 OnClick = Button1Click end object Button2: TButton Left = 281 Top = 8 Width = 90 Height = 25 Caption = 'Button2' TabOrder = 1 OnClick = Button2Click end object Button3: TButton Left = 185 Top = 39 Width = 90 Height = 25 Caption = 'Button3' TabOrder = 2 OnClick = Button3Click end object Button4: TButton Left = 281 Top = 39 Width = 90 Height = 25 Caption = 'Button4' TabOrder = 3 OnClick = Button4Click end object Button5: TButton Left = 185 Top = 70 Width = 90 Height = 25 Caption = 'Button5' TabOrder = 4 OnClick = Button5Click end object Memo1: TMemo Left = 185 Top = 111 Width = 181 Height = 81 Lines.Strings = ( 'Memo1') TabOrder = 5 end object Button6: TButton Left = 281 Top = 70 Width = 90 Height = 25 Caption = 'Button6' TabOrder = 6 OnClick = Button6Click end end