外观模式(Facade)
1 /* 2 * 一个保安系统的,由录像机、电灯、红外线监控和警报器组成。保安系统的操作 3 * 人员需要经常将这些仪器启动和关闭。保安类需要用到所有的录像机(Camera)、 4 * 电灯(Light)、感应器(Sensor)和警报器(Alarm)对象,保安觉得使用不方便。应 5 * 用外观模式,用C#控制台应用程序改进该设计。 6 */ 7 using System; 8 using System.Collections.Generic; 9 using System.Linq; 10 using System.Text; 11 12 namespace Facade 13 { 14 //照相机类 15 class Camera 16 { 17 public void Start() 18 { 19 Console.WriteLine("启动录像机。"); 20 } 21 public void Close() 22 { 23 Console.WriteLine("关闭录像机。"); 24 } 25 } 26 //灯光类 27 class Light 28 { 29 public void Start() 30 { 31 Console.WriteLine("启动灯光。"); 32 } 33 public void Close() 34 { 35 Console.WriteLine("关闭灯光。"); 36 } 37 } 38 //感应器类 39 class Sensor 40 { 41 public void Start() 42 { 43 Console.WriteLine("启动感应器。"); 44 } 45 public void Close() 46 { 47 Console.WriteLine("关闭感应器。"); 48 } 49 } 50 //警报器类 51 class Alarm 52 { 53 public void Start() 54 { 55 Console.WriteLine("启动警报器。"); 56 } 57 public void Close() 58 { 59 Console.WriteLine("关闭警报器。"); 60 } 61 } 62 //遥控器类 63 class RemoteControl 64 { 65 private Alarm alarm; 66 private Sensor sensor; 67 private Light light; 68 private Camera camera; 69 70 public RemoteControl() 71 { 72 alarm = new Alarm(); 73 sensor = new Sensor(); 74 light = new Light(); 75 camera = new Camera(); 76 } 77 public void StartCamera() 78 { 79 camera.Start(); 80 } 81 public void CloseCamera() 82 { 83 camera.Close(); 84 } 85 public void StartLight() 86 { 87 light.Start(); 88 } 89 public void CloseLight() 90 { 91 light.Close(); 92 } 93 public void StartSensor() 94 { 95 sensor.Start(); 96 } 97 public void CloseSensor() 98 { 99 sensor.Close(); 100 } 101 public void StartAlarm() 102 { 103 alarm.Start(); 104 } 105 public void CloseAlarm() 106 { 107 alarm.Close(); 108 } 109 } 110 //保安类 111 class Security 112 { 113 private string name; 114 private RemoteControl rc; 115 public Security(string name,RemoteControl rc) 116 { 117 this.name = name; 118 this.rc = rc; 119 Console.WriteLine("{0}值班中。他手里拿了一个遥控器。\n", name); 120 } 121 public RemoteControl Control() 122 { 123 return this.rc; 124 } 125 } 126 class Program 127 { 128 static void Main(string[] args) 129 { 130 Security baoan = new Security("包鞍",new RemoteControl()); 131 132 baoan.Control().StartAlarm(); 133 baoan.Control().StartCamera(); 134 baoan.Control().StartLight(); 135 baoan.Control().StartSensor(); 136 137 Console.WriteLine(""); 138 baoan.Control().CloseAlarm(); 139 baoan.Control().CloseCamera(); 140 baoan.Control().CloseLight(); 141 baoan.Control().CloseSensor(); 142 } 143 } 144 }
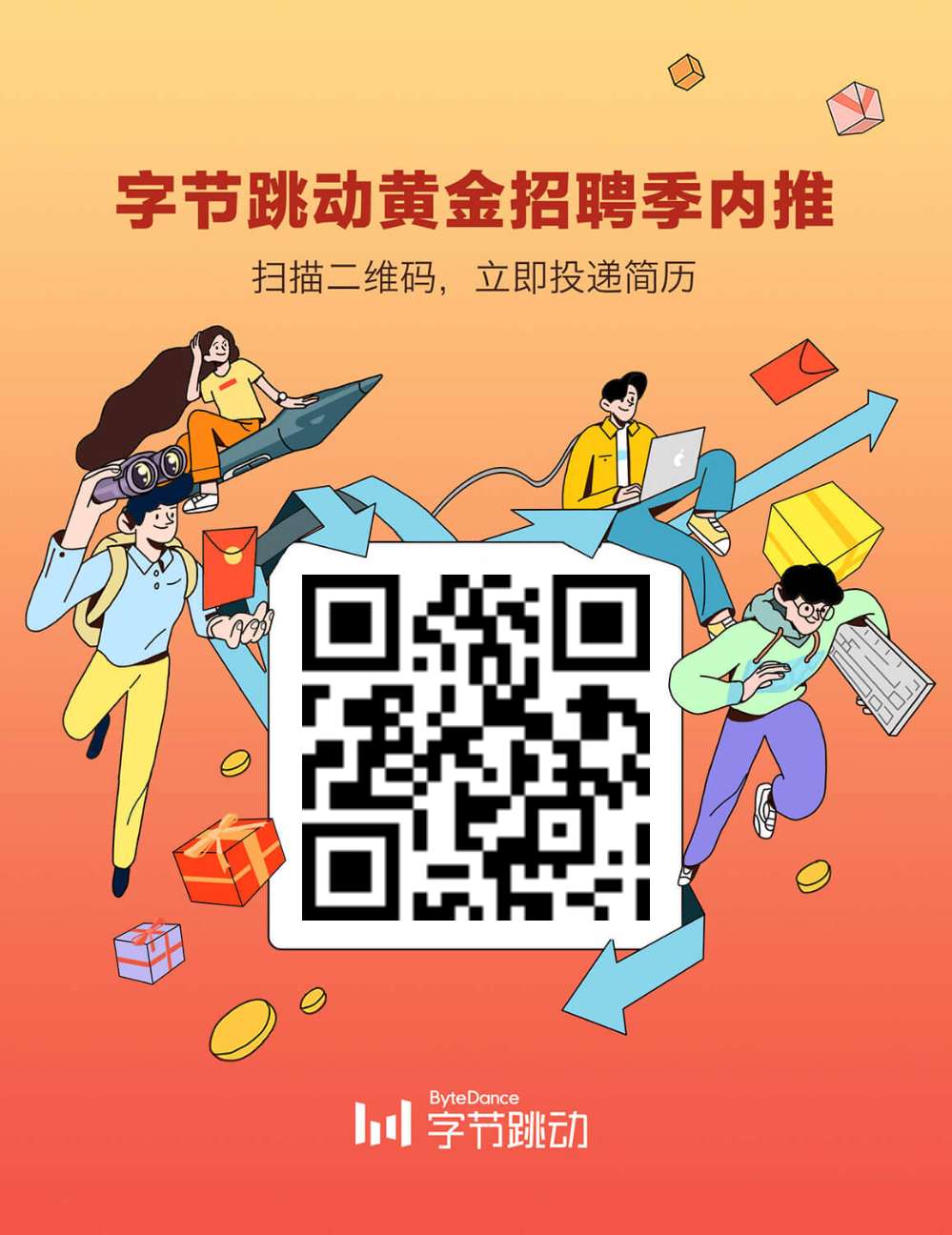
找我内推: 字节跳动各种岗位
作者:
ZH奶酪(张贺)
邮箱:
cheesezh@qq.com
出处:
http://www.cnblogs.com/CheeseZH/
*
本文版权归作者和博客园共有,欢迎转载,但未经作者同意必须保留此段声明,且在文章页面明显位置给出原文连接,否则保留追究法律责任的权利。