适配器模式(Adapter)
1 /* 2 * 一个软件团队开发绘图系统,设计了圆对象(Circle)、矩形对象(Rectangle),线对象(Line) 3 * 都支持Draw()函数,即可以通过Draw()函数绘制图形。为了加快项目进度,将角度对象(Angle) 4 * 绘制功能交给了合作团队实现。但合作团队将角度对象绘制函数定为了DrawAngle()。 5 * 绘图系统提供给用户后,用户不满意,希望能统一的调用,不用记太多命令。 6 * 应用适配器模式,用C#控制台应用程序完善该设计。 7 */ 8 using System; 9 using System.Collections.Generic; 10 using System.Linq; 11 using System.Text; 12 13 namespace Adapter 14 { 15 abstract class Graphic 16 { 17 public abstract void Draw(); 18 } 19 class Circle : Graphic 20 { 21 public override void Draw() 22 { 23 Console.WriteLine("画了一个圆。"); 24 } 25 } 26 class Rectangle : Graphic 27 { 28 public override void Draw() 29 { 30 Console.WriteLine("画了一个矩形。"); 31 } 32 } 33 class Line : Graphic 34 { 35 public override void Draw() 36 { 37 Console.WriteLine("画了一条直线。"); 38 } 39 } 40 class Angle 41 { 42 public void DrawAngle() 43 { 44 Console.WriteLine("画了个角。"); 45 } 46 } 47 class Adapter : Graphic 48 { 49 private Angle angle = new Angle(); 50 public override void Draw() 51 { 52 angle.DrawAngle(); 53 } 54 } 55 class Program 56 { 57 static void Main(string[] args) 58 { 59 Graphic circle = new Circle(); 60 Graphic rectangle = new Rectangle(); 61 Graphic line = new Line(); 62 Graphic angle = new Adapter(); 63 circle.Draw(); 64 rectangle.Draw(); 65 line.Draw(); 66 angle.Draw(); 67 } 68 } 69 }
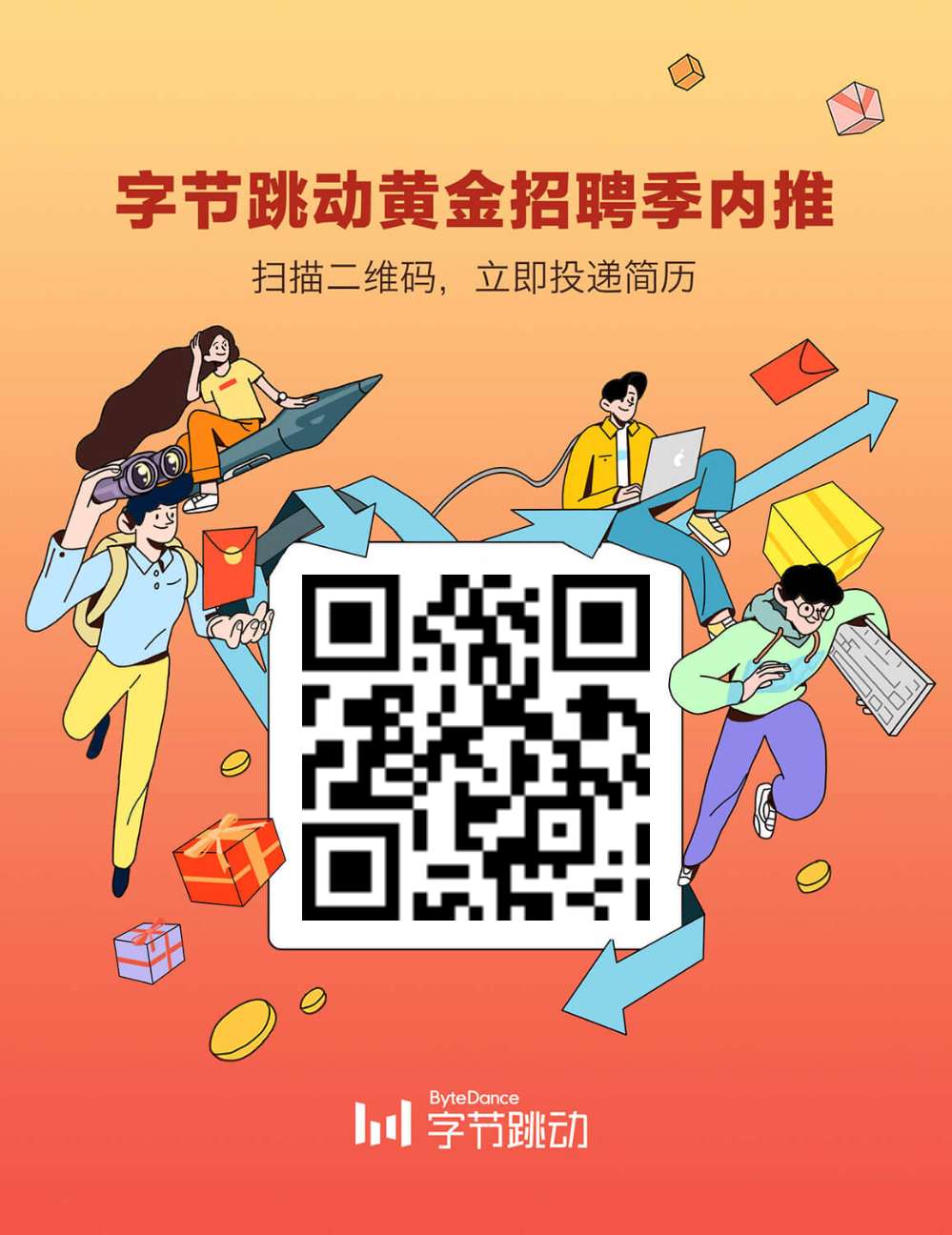
找我内推: 字节跳动各种岗位
作者:
ZH奶酪(张贺)
邮箱:
cheesezh@qq.com
出处:
http://www.cnblogs.com/CheeseZH/
*
本文版权归作者和博客园共有,欢迎转载,但未经作者同意必须保留此段声明,且在文章页面明显位置给出原文连接,否则保留追究法律责任的权利。