.net Core EF统一配置实体类型
一般情况需要对某个实体进行一些配置时代码如下:
protected override void OnModelCreating(ModelBuilder modelBuilder) { base.OnModelCreating(modelBuilder); modelBuilder.Entity<Product>().Property(p => p.Name).HasMaxLength(50).IsRequired(); }
如果按照上面代码对实体类型进行配置,当实体Entity特别多时难免不便于维护,如果能自动加载执行实体配置的相关类再好不过了
以下代码即可实现统一加载实体配置类并执行
using Microsoft.EntityFrameworkCore; using System; using System.Collections.Generic; using System.Text; using Microsoft.EntityFrameworkCore.Metadata.Builders; using System.Reflection; using System.Linq; namespace DAL.Mapping { public interface IEntityMappingConfiguration { void Map(ModelBuilder b); } public interface IEntityMappingConfiguration<T> : IEntityMappingConfiguration where T : class { void Map(EntityTypeBuilder<T> builder); } public abstract class EntityMappingConfiguration<T> : IEntityMappingConfiguration<T> where T : class { public abstract void Map(EntityTypeBuilder<T> b); public void Map(ModelBuilder b) { Map(b.Entity<T>()); } } public static class ModelBuilderExtenions { private static IEnumerable<Type> GetMappingTypes(this Assembly assembly, Type mappingInterface) { return assembly.GetTypes().Where(x => !x.IsAbstract && x.GetInterfaces().Any(y => y.GetTypeInfo().IsGenericType && y.GetGenericTypeDefinition() == mappingInterface)); } public static void AddEntityConfigurationsFromAssembly(this ModelBuilder modelBuilder, Assembly assembly) { var mappingTypes = assembly.GetMappingTypes(typeof(IEntityMappingConfiguration<>)); foreach (var config in mappingTypes.Select(Activator.CreateInstance).Cast<IEntityMappingConfiguration>()) { config.Map(modelBuilder); } } } }
使用:
using Entity.Table; using Microsoft.EntityFrameworkCore; using Microsoft.EntityFrameworkCore.Metadata.Builders; using System; using System.Collections.Generic; using System.Text; namespace DAL.Mapping { public class ProductConfiguration : EntityMappingConfiguration<Product> { public override void Map(EntityTypeBuilder<Product> b) { b.ToTable("Product", "HumanResources") .HasKey(p => p.Id); b.Property(p => p.Name).HasMaxLength(50).IsRequired(); } } }
自动加载加载并执行继承了EntityMappingConfiguration的类达到统一对实体类型进行配置的目的
protected override void OnModelCreating(ModelBuilder modelBuilder) { base.OnModelCreating(modelBuilder); modelBuilder.AddEntityConfigurationsFromAssembly(GetType().Assembly); }
参考:
https://stackoverflow.com/questions/26957519/ef-core-mapping-entitytypeconfiguration#comment46244976_26957519

作者:Chaunce
GitHub:https://github.com/liuyl1992
公众号请搜:架构师高级俱乐部 SmartLife_com
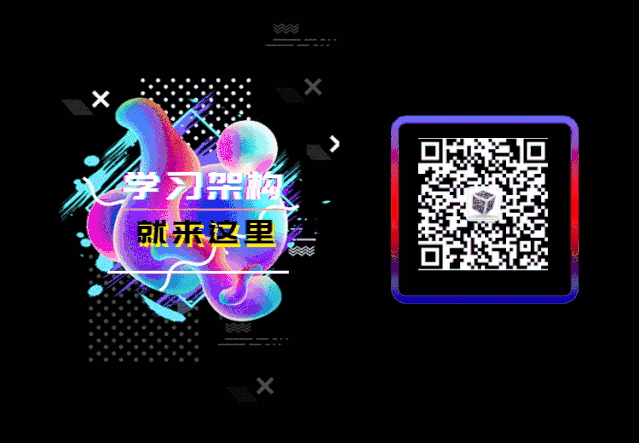
声明:原创博客请在转载时保留原文链接或者在文章开头加上本人博客地址,如发现错误,欢迎批评指正。凡是转载于本人的文章,不能设置打赏功能等盈利行为