Selenium webdriver 操作日历控件
一般的日期控件都是input标签下弹出来的,如果使用webdriver 去设置日期,
1. 定位到该input
2. 使用sendKeys 方法
比如:
但是,有的日期控件是readonly的
比如12306的这个
<input id="train_date" class="inp-txt" type="text" value="2015-03-15" name="back_train_date" autocomplete="off" maxlength="10" readonly="readonly" disabled="disabled">
这个时候,没法调用WebElement的sendKeys()
方案一:使用JS remove readonly attribute,然后sendKeys
还是以万恶的12306为例:
使用出发日期,将input标签的readonly熟悉去掉
JavascriptExecutor removeAttribute = (JavascriptExecutor)driver; //remove readonly attribute removeAttribute.executeScript("var setDate=document.getElementById(\"train_date\");setDate.removeAttribute('readonly');") ;
方案二:采用click直接选择日期,日期控件是一个iframe,首先switch iframe,之后找到想要设置的日期button click,然后switch出来
WebElement dayElement=driver.findElement(By.xpath("//span[@id='from_imageClick']"));
dayElement.click();
// WebElement frameElement=driver.findElement(By.xpath("//iframe[@border='0']"));
driver.switchTo().frame(1);
driver.findElement(By.xpath("//tr/td[@onclick='day_Click(2015,2,21);']")).click();
driver.switchTo().defaultContent();
具体代码如下:
WebDriver driver=DriverFactory.getFirefoxDriver(); driver.get("https://kyfw.12306.cn/otn/"); driver.manage().window().maximize(); driver.manage().timeouts().pageLoadTimeout(60, TimeUnit.SECONDS); driver.manage().window().maximize(); driver.manage().timeouts().implicitlyWait(60, TimeUnit.SECONDS); driver.manage().timeouts().pageLoadTimeout(30, TimeUnit.SECONDS); WebElement fromStation=driver.findElement(By.xpath("//input[@id='fromStationText']")); fromStation.click(); fromStation.sendKeys("郑州"); WebElement choseFrom =driver.findElement(By.xpath("//div/span[@class='ralign' and text()='郑州']")); choseFrom.click(); WebElement toStation=driver.findElement(By.xpath("//input[@id='toStationText']")); toStation.click(); toStation.sendKeys("上海"); WebElement choseElement =driver.findElement(By.xpath("//div/span[@class='ralign' and text()='上海']")); choseElement.click(); JavascriptExecutor removeAttribute = (JavascriptExecutor)driver; //remove readonly attribute removeAttribute.executeScript("var setDate=document.getElementById(\"train_date\");setDate.removeAttribute('readonly');") ; WebElement setDatElement=driver.findElement(By.xpath("//input[@id='train_date']")); setDatElement.clear(); setDatElement.sendKeys("2015-02-18"); WebElement dayElement=driver.findElement(By.xpath("//span[@id='from_imageClick']")); dayElement.click(); // WebElement frameElement=driver.findElement(By.xpath("//iframe[@border='0']")); driver.switchTo().frame(1); driver.findElement(By.xpath("//tr/td[@onclick='day_Click(2015,2,21);']")).click(); driver.switchTo().defaultContent(); WebElement searchElement=driver.findElement(By.xpath("//div/a[@id='a_search_ticket']")); searchElement.click();
其中:DriverFactory

package com.packt.webdriver.chapter3; import java.io.File; import java.io.IOException; import java.util.Arrays; import org.openqa.selenium.Proxy; import org.openqa.selenium.WebDriver; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.chrome.ChromeDriverService; import org.openqa.selenium.chrome.ChromeOptions; import org.openqa.selenium.firefox.FirefoxDriver; import org.openqa.selenium.firefox.FirefoxProfile; import org.openqa.selenium.firefox.internal.ProfilesIni; import org.openqa.selenium.htmlunit.HtmlUnitDriver; import org.openqa.selenium.ie.InternetExplorerDriver; import org.openqa.selenium.os.WindowsUtils; import org.openqa.selenium.remote.CapabilityType; import org.openqa.selenium.remote.DesiredCapabilities; public class DriverFactory { public static WebDriver getHtmlUnit() { HtmlUnitDriver ht=new HtmlUnitDriver(); return ht; } public static WebDriver getChromeDriver() { // TODO Auto-generated method stub String chromdriver="E:\\chromedriver.exe"; System.setProperty("webdriver.chrome.driver", chromdriver); ChromeDriverService.Builder builder=new ChromeDriverService.Builder(); File file=new File(chromdriver); // int port=12643; // ChromeDriverService service=builder.usingDriverExecutable(file).usingPort(port).build(); // try { // service.start(); // } catch (IOException e) { // // TODO Auto-generated catch block // e.printStackTrace(); // } ChromeOptions options = new ChromeOptions(); options.addExtensions(new File("")); DesiredCapabilities capabilities = DesiredCapabilities.chrome(); capabilities.setCapability("chrome.switches", Arrays.asList("--start-maximized")); options.addArguments("--test-type", "--start-maximized"); WebDriver driver=new ChromeDriver(options); return driver; } public static WebDriver getFirefoxDriver() { try { WindowsUtils.tryToKillByName("firefox.exe"); } catch(Exception e) { System.out.println("can not find firefox process"); } File file=new File("d:\\firebug-2.0.4-fx.xpi"); FirefoxProfile profile = new FirefoxProfile(); // profile.setPreference("network.proxy.type", 2); // profile.setPreference("network.proxy.autoconfig_url", "http://proxy.successfactors.com:8083"); // profile.setPreference("network.proxy.no_proxies_on", "localhost"); // // profile.setPreference("network.proxy.http", "proxy.domain.example.com"); // profile.setPreference("network.proxy.http_port", 8080); // profile.setPreference("network.proxy.ssl", "proxy.domain.example.com"); // profile.setPreference("network.proxy.ssl_port", 8080); // profile.setPreference("network.proxy.ftp", "proxy.domain.example.com"); // profile.setPreference("network.proxy.ftp_port", 8080); // profile.setPreference("network.proxy.socks", "proxy.domain.example.com"); // profile.setPreference("network.proxy.socks_port", 8080); try { profile.addExtension(file); profile.setPreference("extensions.firebug.currentVersion", "2.0.4"); profile.setPreference("extensions.firebug.allPagesActivation", "on"); } catch (IOException e3) { // TODO Auto-generated catch block e3.printStackTrace(); } WebDriver driver = new FirefoxDriver(profile); return driver; } public static WebDriver getIEDriver() { String IEDriverServer="E:\\IEDriverServer.exe"; System.setProperty("webdriver.ie.driver",IEDriverServer); WebDriver driver=new InternetExplorerDriver(); return driver; } }
转载请注明出处:http://www.cnblogs.com/tobecrazy/
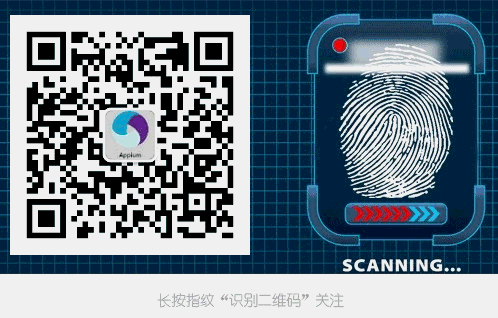