分享一个实用的网络连接类
手机开发中常常需要用到网络连接发送数据,像web一样,手机中的请求也有GET请求和POST请求之分。
以下是一个HttpProcess类,它提供了POST和GET请求的方式。
package com.thinkrace.UCHome.network; import java.io.ByteArrayInputStream; import java.io.ByteArrayOutputStream; import java.io.DataInputStream; import java.io.DataOutputStream; import java.io.IOException; import java.io.InputStream; import java.io.InputStreamReader; import java.io.OutputStream; import javax.microedition.io.Connector; import javax.microedition.io.HttpConnection; /** * * @author Administrator */ public class HttpProcess { /** * HTTP GET请求方式 * 此方法读取返回的xml时是一个字符一个字符读取的。 * @param url * @return */ public static String HttpGET(String url) { String content = null; try { //打开网络连接 HttpConnection hc = (HttpConnection) Connector.open(url); hc.setRequestMethod(HttpConnection.GET); InputStreamReader isr = new InputStreamReader(hc.openInputStream(), "UTF-8"); int ch = 0; StringBuffer buf = new StringBuffer(); while ((ch = isr.read()) != -1) { buf.append((char) ch); } //关闭流 isr.close(); hc.close(); } catch (IOException ex) { System.out.println("HttpGET方法出现异常"); ex.printStackTrace(); } return content; } /** * 分配固定内存的GET请求,无论返回的xml有多大,总是只显示5k * 超出的部分会被截断,剩余的将会用空白补齐 * @param url * @return */ public static String HttpGETByAssignMemory(String url) { String content = null; try { HttpConnection hc = (HttpConnection) Connector.open(url); hc.setRequestMethod(HttpConnection.GET); DataInputStream dis = new DataInputStream(hc.openDataInputStream()); //分配5K的内存 byte[] data = new byte[1024 * 5]; dis.read(data); content = new String(data, "UTF-8"); } catch (IOException ex) { System.out.println("HttpGETByAllocateMemory方法出现异常"); ex.printStackTrace(); } return content; } public static String HttpPOST(String url, String argument) { String xml = null; try { HttpConnection hc = (HttpConnection) Connector.open(url, Connector.READ_WRITE); hc.setRequestMethod(HttpConnection.POST); //设置POST的头信息,UserAgent(设置代理),Content-Language(语言),Content-Type(类型),Content-Length(POST的长度) hc.setRequestProperty("User-Agent", "Profile/MIDP-2.0 Configuration/CLDC-1.1"); hc.setRequestProperty("Content-Language", "en-US"); hc.setRequestProperty("Content-Type", "application/x-www-form-urlencoded"); byte[] postData = argument.getBytes("UTF-8"); hc.setRequestProperty("Content-Length", Integer.toString(postData != null ? postData.length : 0)); OutputStream out = hc.openOutputStream(); out.write(postData); InputStream in = hc.openInputStream(); DataInputStream dis = new DataInputStream(in); int ch = 0; StringBuffer buf = new StringBuffer(); while((ch = dis.read()) != -1){ buf.append((char)ch); } xml = buf.toString(); } catch (IOException ex) { System.out.println("HttpPOST方法出现异常"); ex.printStackTrace(); } return xml; } /** * String转换成byte数组 * @param str * @return */ public static byte[] StringtoBytes(String str) { byte[] data = null; try { ByteArrayOutputStream baos = new ByteArrayOutputStream(); DataOutputStream dos = new DataOutputStream(baos); dos.writeUTF(str); data = baos.toByteArray(); baos.close(); dos.close(); } catch (Exception ex) { System.out.println("StringtoBytes方法出现异常"); ex.printStackTrace(); } return data; } /** * byte[]数组转换成String * @param data * @return */ public static String BytesToString(byte[] data) { ByteArrayInputStream bais = new ByteArrayInputStream(data); DataInputStream dis = new DataInputStream(bais); String str = null; try { str = dis.readUTF(); bais.close(); dis.close(); } catch (Exception ex) { System.out.println("BytesToString方法出现异常"); ex.printStackTrace(); } return str; } }
以下载一张小图片为例子,一个较为简单的HTTP连接的demo。
支持CMNET及CMWAP两种不同的接入方式,以及在CMWAP接入方式下,过滤移动资费页面。
在6120c上测试通过。
以下载一张小图片为例子,一个较为简单的HTTP连接的demo。
支持CMNET及CMWAP两种不同的接入方式,以及在CMWAP接入方式下,过滤移动资费页面。
在6120c上测试通过。
import java.io.IOException; import java.io.InputStream; import javax.microedition.io.Connector; import javax.microedition.io.HttpConnection; import javax.microedition.lcdui.Command; import javax.microedition.lcdui.CommandListener; import javax.microedition.lcdui.Display; import javax.microedition.lcdui.Displayable; import javax.microedition.lcdui.Form; import javax.microedition.lcdui.Image; import javax.microedition.lcdui.StringItem; import javax.microedition.midlet.MIDlet; /** * HttpDemo * * @author kf156(亚日) * */ public class HttpTest extends MIDlet implements CommandListener, Runnable { public static final byte WAIT = 0;// 等待 public static final byte CONNECT = 1;// 连接中 public static final byte SUCCESS = 2;// 成功 public static final byte FAIL = 3;// 失败 int state;// 当前状态 Display display = Display.getDisplay(this); Form form = new Form("HttpTest"); boolean cmnet = true;// 接入点为cmnet还是cmwap StringBuffer sb = new StringBuffer("当前接入方式为:CMNET\n"); StringItem si = new StringItem(null, sb.toString()); Command connect = new Command("联网", Command.OK, 1); Command change = new Command("改变接入点方式", Command.OK, 2); Command exit = new Command("退出", Command.EXIT, 1); HttpConnection http; String host = "wiki.huihoo.com"; String path = "/images/9/9c/Java.gif"; public HttpTest() { state = WAIT;// 等待状态 form.append(si); form.addCommand(connect); form.addCommand(change); form.addCommand(exit); form.setCommandListener(this); } protected void destroyApp(boolean b) { } protected void pauseApp() { } protected void startApp() { display.setCurrent(form); } public void commandAction(Command c, Displayable d) { if (c == change) {// 改变接入点 if (isConnect()) return; cmnet = !cmnet; form.deleteAll(); sb.delete(0, sb.length()); addStr("当前接入方式为:" + (cmnet ? "CMNET" : "CMWAP")); form.append(si); } else if (c == connect) {// 联网 if (isConnect()) return; new Thread(this).start(); } else if (c == exit) {// 退出 destroyApp(true); notifyDestroyed(); } } public void run() { form.deleteAll(); sb.delete(0, sb.length()); addStr("当前接入方式为:" + (cmnet ? "CMNET" : "CMWAP")); form.append(si); state = CONNECT; addStr("网络连接中..."); InputStream is = null; try { String url = null; url = cmnet ? ("http://" + host + path) : ("http://10.0.0.172:80" + path); http = (HttpConnection) Connector.open(url, Connector.READ_WRITE, true); if (!cmnet) http.setRequestProperty("X-Online-Host", host); http.setRequestMethod(HttpConnection.GET); String contentType = http.getHeaderField("Content-Type"); System.out.println(contentType); addStr(contentType); if (contentType != null && contentType.indexOf("text/vnd.wap.wml") != -1) {// 过滤移动资费页面 addStr("移动资费页面,过滤!"); http.close(); http = null; http = (HttpConnection) Connector.open(url, Connector.READ_WRITE, true); if (!cmnet) http.setRequestProperty("X-Online-Host", host); http.setRequestMethod(HttpConnection.GET); contentType = http.getHeaderField("Content-Type"); } addStr("Content-Type=" + contentType); int code = http.getResponseCode(); addStr("HTTP Code :" + code); if (code == 200) { addStr("网络联网成功,接收数据..."); is = http.openInputStream(); Image image = Image.createImage(is); addStr("数据接收完毕,显示图片"); form.append(image); // 普通字节数据接收 // byte[] b = new byte[1024]; // int len = 0; // ByteArrayOutputStream baos = new ByteArrayOutputStream(); // DataOutputStream dos = new DataOutputStream(baos); // while ((len = is.read(b)) != -1) { // dos.write(b, 0, len); // } // dos.close(); // dos = null; // is.close(); // is = null; state = SUCCESS; return; } else { addStr("访问页面失败"); } } catch (IOException e) { addStr("联网发生异常:" + e.toString()); e.printStackTrace(); } catch (Exception e) { addStr("发生异常:" + e.toString()); e.printStackTrace(); } finally { if (is != null) { try { is.close(); } catch (IOException e) { e.printStackTrace(); } is = null; } if (http != null) try { http.close(); } catch (IOException e) { e.printStackTrace(); } http = null; } state = FAIL; } /** * 判断是否正在连接 * * @return */ private boolean isConnect() { if (state == CONNECT) { addStr("网络连接中,请稍候..."); return true; } return false; } /** * 添加文字 * * @param str * 要添加的文字 */ private void addStr(String str) { sb.append(str + "\n"); si.setText(sb.toString()); } }
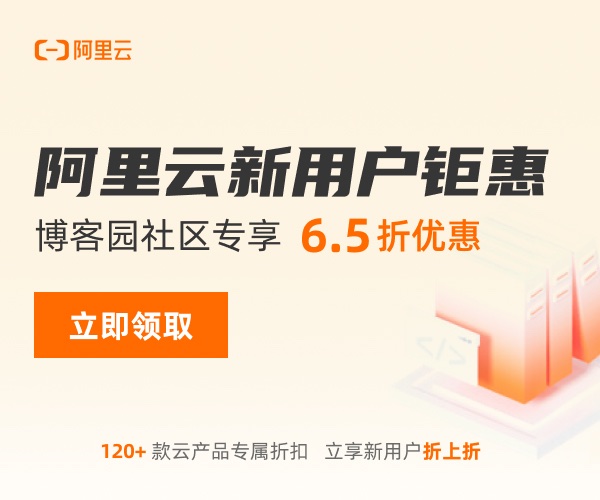
