Get CellId and other Imformation from mobile phones via J2ME
Without GPS or Network Operator we can still use the Location Based Service, via the help of CellId(Cell ID the location of Cell Identities of GSM-networks). Google has one enormous database of cell ids which are linked to a longitude and latitude position, that why Google Map client know where you are even you have no embedded GPS--it is not open. But there are other opened cellid DBs that we can use freely: opencellid , mobiforge.com and http://realtimeblog.free.fr/
Ok, one we can get the cell from phone we can query the location from these cellid DB. In GSM networks, all cells in the world have a globally unique ID made up of four numbers:
cell ID, LAC, MNC, and MCC.
For example, for Motorola:
Using JavaME to obtain Cell Positioning information
{ …. String cellid = System.getProperty("CellID"); String lac = System.getProperty("LocAreaCode"); String imsi = System.getProperty("IMSI"); // Example IMSI (O2 UK): 234103530089555 String mcc = imsi.substring(0,3); // 234 (UK) String mnc = imsi.substring(3,5); // 10 (O2) String location = mcc + mnc + lac + cellID; // A globally unique ID for a cell }
Information from Truly Mobile JavaME Applications: Location, Media Capture, and Connectivity
But get Cellid is still limited by mobile phone platform, Sign-certificate and Operator:
Nokia
s40 3rd Fp1 edition, requers operator or manufactureer signing
S60 3rd edition, FP2(released 2008 and newer, does not work on e.g. N95),no singing required
SonyEricsson:
Java platform 7.3 or higher (released around 2006 and newer)
older phone may need firmware update (7.1->7.3)
does not work in SE symbian (ie. UIQ) or windownsmobile(Xperia X1) phone
Information from Terminal-based Mobile Positioning overview
And notice, even you use the right code and sign your application, it is still not possible to query the cellID, because some telecom-operators have limited this service.
The fowllowing J2ME code could help you, J2ME Polish Syntax is used here to make the procedure easier. The functions here is not complete, because some manufactories have not released their J2ME API documents to query the cellID. If you have some information which is not included in the codes please let me and others know, please supplement here, thx!
/** * get the cell id in the phone * * @return */ public static String getCellId(){ String out = ""; try{ out = System.getProperty("Cell-ID"); if(out== null || out.equals("null") || out.equals("")) out = System.getProperty("CellID"); if(out== null ||out.equals("null")|| out.equals("")) System.getProperty("phone.cid"); //#if polish.Vendor == Nokia if(out== null ||out.equals("null")|| out.equals("")) out = System.getProperty("com.nokia.mid.cellid"); //#elif polish.Vendor == Sony-Ericsson if(out== null ||out.equals("null")|| out.equals("")) out = System.getProperty("com.sonyericsson.net.cellid"); //#elif polish.Vendor == Motorola if(out== null ||out.equals("null")|| out.equals("")) out = System.getProperty("phone.cid");//System.getProperty("CellID"); //#elif polish.Vendor == Samsung if(out== null ||out.equals("null")|| out.equals("")) out = System.getProperty("com.samsung.cellid"); //#elif polish.Vendor == Siemens if(out== null ||out.equals("null")|| out.equals("")) out = System.getProperty("com.siemens.cellid"); //#elif polish.Vendor == BlackBerry if(out== null ||out.equals("null")|| out.equals("")) //#= out = GPRSInfo.getCellInfo().getCellId(); //#else if(out== null ||out.equals("null")|| out.equals("")) out = System.getProperty("cid"); //#endif }catch(Exception e){ return out==null?"":out; } return out==null?"":out; } /** * get the lac sring from phone */ public static String getLAC(){ String out = ""; try{ out = System.getProperty("phone.lac"); //#if polish.Vendor == Nokia if(out== null ||out.equals("null")|| out.equals("")) out = System.getProperty("com.nokia.mid.lac"); //#elif polish.Vendor == Sony-Ericsson if(out== null ||out.equals("null")|| out.equals("")) out = System.getProperty("com.sonyericsson.net.lac"); //#elif polish.Vendor == Motorola if(out== null ||out.equals("null")|| out.equals("")) out = System.getProperty("LocAreaCode"); //#elif polish.Vendor == Samsung if(out== null ||out.equals("null")|| out.equals("")) out = System.getProperty("com.samsung.cellid"); //#elif polish.Vendor == Siemens if(out== null ||out.equals("null")|| out.equals("")) out = System.getProperty("com.siemens.cellid"); //#elif polish.Vendor == BlackBerry if(out== null ||out.equals("null")|| out.equals("")) //#= out = GPRSInfo.getCellInfo().getLAC(); //#else if(out== null ||out.equals("null")|| out.equals("")) out = System.getProperty("cid"); //#endif }catch(Exception e){ return out==null?"":out; } return out==null?"":out; } /** * Example IMSI (O2 UK): 234103530089555 String mcc = imsi.substring(0,3); // 234 (UK) String mnc = imsi.substring(3,5); // 10 (O2) * @return */ public static String getIMSI(){ String out = ""; try{ out = System.getProperty("IMSI"); if(out== null ||out.equals("null")|| out.equals("")) out = System.getProperty("phone.imsi") ; //#if polish.Vendor == Nokia if(out== null ||out.equals("null")|| out.equals("")) out = System.getProperty("com.nokia.mid.mobinfo.IMSI"); if(out== null ||out.equals("null")|| out.equals("")) out = System.getProperty("com.nokia.mid.imsi"); //#elif polish.Vendor == Sony-Ericsson /* if(out== null ||out.equals("null")|| out.equals("")) out = System.getProperty("com.sonyericsson.imsi");*/ //#elif polish.Vendor == Motorola if(out== null ||out.equals("null")|| out.equals("")) out = System.getProperty("IMSI"); //#elif polish.Vendor == Samsung /* if(out== null ||out.equals("null")|| out.equals("")) out = System.getProperty("com.samsung.imei");*/ //#elif polish.Vendor == Siemens /* if(out== null ||out.equals("null")|| out.equals("")) out = System.getProperty("com.siemens.imei");*/ //#elif polish.Vendor == BlackBerry if(out== null ||out.equals("null")|| out.equals("")) //#= out = GPRSInfo.getCellInfo().getBSIC(); //#else if(out== null ||out.equals("null")|| out.equals("")) out = System.getProperty("imsi"); //#endif }catch(Exception e){ return out==null?"":out; } return out==null?"":out; } /** * * For moto, Example IMSI (O2 UK): 234103530089555 String mcc = imsi.substring(0,3); // 234 (UK) * @return */ public static String getMCC(){ String out = ""; try{ if(out== null ||out.equals("null")|| out.equals("")) out = System.getProperty("phone.mcc") ; //#if polish.Vendor == Nokia if(out== null ||out.equals("null")|| out.equals("")) //out = System.getProperty("com.nokia.mid.mobinfo.IMSI"); //#elif polish.Vendor == Sony-Ericsson if(out== null ||out.equals("null")|| out.equals("")) out = System.getProperty("com.sonyericsson.net.mcc"); //#elif polish.Vendor == Motorola if(out== null ||out.equals("null")|| out.equals("")){ out = getIMSI().equals("")?"": getIMSI().substring(0,3); } //#elif polish.Vendor == Samsung /* if(out== null ||out.equals("null")|| out.equals("")) out = System.getProperty("com.samsung.imei");*/ //#elif polish.Vendor == Siemens /* if(out== null ||out.equals("null")|| out.equals("")) out = System.getProperty("com.siemens.imei");*/ //#elif polish.Vendor == BlackBerry if(out== null ||out.equals("null")|| out.equals(""))//getMNC() //#= out = GPRSInfo.getCellInfo().getMCC(); //#else if(out== null ||out.equals("null")|| out.equals("")) out = System.getProperty("mcc"); //#endif }catch(Exception e){ return out==null?"":out; } return out==null?"":out; } /** * * For moto, Example IMSI (O2 UK): 234103530089555 String mnc = imsi.substring(3,5); // 10 (O2) * @return */ public static String getMNC(){ String out = ""; try{ if(out== null ||out.equals("null")|| out.equals("")) out = System.getProperty("phone.mnc") ; //#if polish.Vendor == Nokia if(out== null ||out.equals("null")|| out.equals("")) out = getIMSI().equals("")?"": getIMSI().substring(3,5); //#elif polish.Vendor == Sony-Ericsson if(out== null ||out.equals("null")|| out.equals("")) out = System.getProperty("com.sonyericsson.net.mnc"); //#elif polish.Vendor == Motorola if(out== null ||out.equals("null")|| out.equals("")){ out = getIMSI().equals("")?"": getIMSI().substring(3,5); } //#elif polish.Vendor == Samsung /* if(out== null ||out.equals("null")|| out.equals("")) out = System.getProperty("com.samsung.imei");*/ //#elif polish.Vendor == Siemens /* if(out== null ||out.equals("null")|| out.equals("")) out = System.getProperty("com.siemens.imei");*/ //#elif polish.Vendor == BlackBerry if(out== null ||out.equals("null")|| out.equals(""))//getMNC() //#= out = GPRSInfo.getCellInfo().getMCC(); //#else if(out== null ||out.equals("null")|| out.equals("")) out = System.getProperty("mnc"); //#endif }catch(Exception e){ return out==null?"":out; } return out==null?"":out; } /** * not used now * get the IMEI (International Mobile Equipment Identity (IMEI)) in the phone * * @return */ public static String getIMEI(){ String out = ""; try{ out = System.getProperty("com.imei"); //#if polish.Vendor == Nokia if(out== null ||out.equals("null")|| out.equals("")) out = System.getProperty("phone.imei"); if(out== null ||out.equals("null")|| out.equals("")) out = System.getProperty("com.nokia.IMEI"); if(out== null ||out.equals("null")|| out.equals("")) out = System.getProperty("com.nokia.mid.imei"); if(out== null ||out.equals("null")|| out.equals("")) out = System.getProperty("com.nokia.mid.imei"); //#elif polish.Vendor == Sony-Ericsson if(out== null ||out.equals("null")|| out.equals("")) out = System.getProperty("com.sonyericsson.imei"); //#elif polish.Vendor == Motorola if(out== null ||out.equals("null")|| out.equals("")) out = System.getProperty("IMEI"); if(out== null ||out.equals("null")|| out.equals("")) out = System.getProperty("com.motorola.IMEI"); //#elif polish.Vendor == Samsung if(out== null ||out.equals("null")|| out.equals("")) out = System.getProperty("com.samsung.imei"); //#elif polish.Vendor == Siemens if(out== null ||out.equals("null")|| out.equals("")) out = System.getProperty("com.siemens.imei"); //#else if(out== null ||out.equals("null")|| out.equals("")) out = System.getProperty("imei"); //#endif }catch(Exception e){ return out==null?"":out; } return out==null?"":out; }
Some J2ME applications are also available:
http://www.ottaky.com/midlets.php#netmon2
http://www.cellspotting.com/webpages/cellspotting.html
Ref:
http://en.wikipedia.org/wiki/GSM_localization
http://www.paxmodept.com/telesto/blogitem.htm?id=531
http://www.slideshare.net/jaakl/terminalbased-mobile-positioning-overview-presentation
http://www.ottaky.com/midlets.php#netmon2
http://www.cellspotting.com/webpages/cellspotting.html
原文出处:http://www.easywms.com/easywms/?q=zh-hant/node/3589
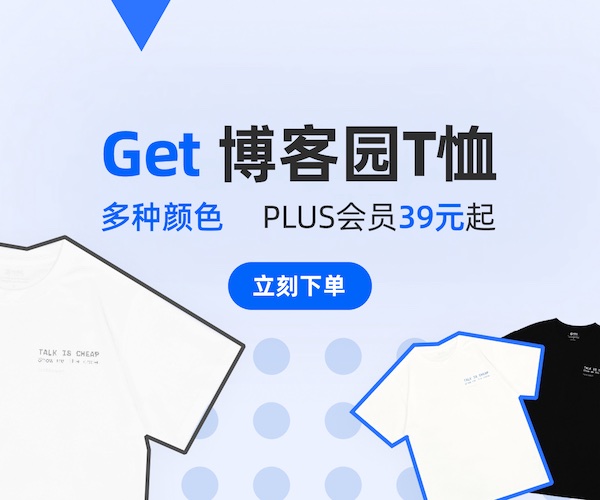