(69)Sqrt(x)
Implement int sqrt(int x)
.
Compute and return the square root of x.
example:
sqrt(3) = 1
sqrt(4) = 2
sqrt(5) = 2
sqrt(10) = 3
challenge:O(log(x))
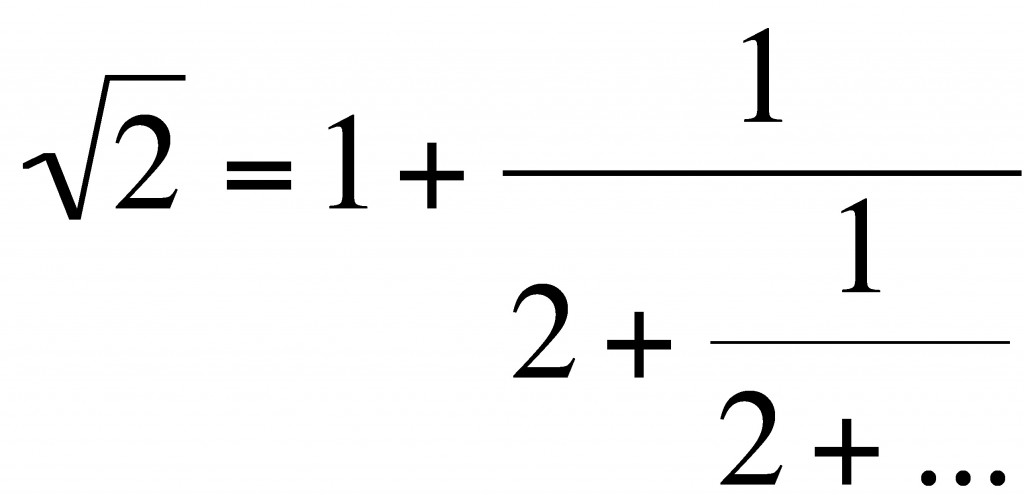
we start with two initial values - one value a below √Q and another value b above √Q, where Q is a positive real number. Since a is a low value, let us denote it by L. Similarly, denote b by H. Let m = (L+H)/2.
If m^2 < Q, let L = m.
If m^2 > Q, let H = m.
Let us find √5. So Q = 5.
since 2^2 <= 5 < 3^2, let L = 2, H = 3.
If m^2 < Q, let L = m.
If m^2 > Q, let H = m.
Let us find √5. So Q = 5.
since 2^2 <= 5 < 3^2, let L = 2, H = 3.
1 public int sqrt(int x) { 2 long lo = 0; 3 long hi = x; 4 while (hi >= lo) { 5 long mid = lo + (hi - lo) / 2; 6 System.out.println("befor " + "lo:" + lo + " hi:" + hi + " mid:" + mid); 7 if (x < mid * mid) { 8 hi = mid - 1; // not hi = mid 9 } else { 10 lo = mid + 1; 11 } 12 System.out.println("after " + "lo:" + lo + " hi:" + hi + " mid:" + mid); 13 } 14 return (int)hi; 15 }