Create DataStore using FDO API in Map 3D
A data store is a repository of an integrated set of objects. The objects in a data store are modeled either by classes or feature classes defined within one or more schemas. For example, a data store may contain data for both a LandUse schema and a TelcoOutsidePlant schema. Some data stores can represent data in only one schema, while other data stores can represent data in many schemas (for example, RDBMS-based data stores, such as MySQL).
In this sample, I will create a data store in SQL Server 2008 using OSGEO FDO Provider for SQL Server Spatial. By default the Sql Server, Oracle, MySQL, and SDF providers create data stores that contain FDO metadata. By default the SQL Server Spatial provider creates a data store that does not contain the FDO metadata. To make this provider create a data store that does contain the FDO metadata, you must set the IDataStorePropertyDictionary property IsFdoEnabled to true. The PostGIS provider creates a data store without the FDO metadata.
A provider uses the FDO metadata to:
- Assign a default spatial context to a Geometric Property Definition during schema creation.
Firstly, create a class library in Visutal Studion 2010 or 2008, add references to AutoCAD managed assembly and FDO assebmly, you can find them in Map 3D/Civil 3D installation folder and FDO\bin folder
C:\Program Files\Autodesk\AutoCAD Civil 3D 2011
C:\Program Files\Autodesk\AutoCAD Civil 3D 2011\FDO\bin
By default the SQL Server Spatial provider creates a data store that does not contain the FDO metadata. To make this provider create a data store that does contain the FDO metadata, we need set parameter IsFdoEnabled to true. the value '”true” is case sensetive.
code snippet goes as below:
[CommandMethod("CreateDataStore")] public void CreateDataStore() { ICreateDataStore createDS = m_pConnection.CreateCommand(CommandType.CommandType_CreateDataStore) as ICreateDataStore; IDataStorePropertyDictionary properties = createDS.DataStoreProperties; properties.SetProperty("DataStore", "TestDataStore"); properties.SetProperty("Description", "This is a test datastore"); properties.SetProperty("IsFdoEnabled", "true"); // case sensitive createDS.Execute(); }
Here is the screen-shot we can see from SQL Server Managemet Studio.
Following code enable us to select fdo provider and input parameter at run time.
using Autodesk.AutoCAD; using Autodesk.AutoCAD.Runtime; using OSGeo.FDO; using OSGeo.FDO.Commands; using OSGeo.FDO.Commands.Schema; using OSGeo.FDO.Connections; using Autodesk.AutoCAD.EditorInput; using Autodesk.AutoCAD.ApplicationServices; using OSGeo.FDO.Schema; using OSGeo.FDO.Connections.Capabilities; using OSGeo.FDO.ClientServices; using OSGeo.FDO.Commands.DataStore;
public class Class1 { IConnection m_pConnection = null; Editor ed = Application.DocumentManager.MdiActiveDocument.Editor; FeatureClass m_baseClass; FeatureClass m_riverClass; ProviderCollection m_Providers; string m_sProviderName; [CommandMethod("connect")] public void Connect() { IConnectionManager connMgr; int index; Provider provider; IProviderRegistry registry = FeatureAccessManager.GetProviderRegistry(); m_Providers = registry.GetProviders(); for (int i = 0; i < m_Providers.Count; i++) { provider = m_Providers[i]; ed.WriteMessage(string.Format("FDO support provider {0}, its index is {1} \n", provider.Name, i)); } PromptIntegerResult intRes = ed.GetInteger("please input the provider's index"); if (intRes.Status == PromptStatus.OK) { index = intRes.Value; provider = m_Providers[index]; m_sProviderName = provider.Name; string shortName = provider.Name.Split('.')[1]; try { connMgr = FeatureAccessManager.GetConnectionManager(); m_pConnection = connMgr.CreateConnection(m_sProviderName); IConnectionInfo connInfo = m_pConnection.ConnectionInfo; IConnectionPropertyDictionary properties = connInfo.ConnectionProperties; InputParametersValue(properties); ConnectionState connState = m_pConnection.Open(); ed.WriteMessage("connect status is "+connState.ToString() + "\n"); } catch (OSGeo.FDO.Common.Exception exception) { ed.WriteMessage("There are some exceptions with message : " + exception.Message + "\n"); } } else { ed.WriteMessage("you did not select a correct provider , exit \n"); return; } } [CommandMethod("DisConnect")] public void CloseConnection() { m_pConnection.Close(); } private void InputParametersValue(IConnectionPropertyDictionary properties) { string[] propertiesNames = properties.PropertyNames; foreach (string name in propertiesNames) { PromptStringOptions pso = new PromptStringOptions("Please input the value for \"" + name + "\":"); PromptResult psr = ed.GetString(pso); if (properties.IsPropertyRequired(name)) { while (psr.Status != PromptStatus.OK) { ed.WriteMessage(string.Format("Parameter \"{0}\" is required, please input value again\n", name)); psr = ed.GetString(pso); } properties.SetProperty(name, psr.StringResult); } } } private void ListPropertiesParameters(IDataStorePropertyDictionary properties) { foreach (string name in properties.PropertyNames) { ed.WriteMessage(name + "\n"); } } [CommandMethod("CreateDataStore")] public void CreateDataStore() { ICreateDataStore createDS = m_pConnection.CreateCommand(CommandType.CommandType_CreateDataStore) as ICreateDataStore; IDataStorePropertyDictionary properties = createDS.DataStoreProperties; InputParametersValue(properties); createDS.Execute(); } }
Cheers,
Daniel 峻祁连
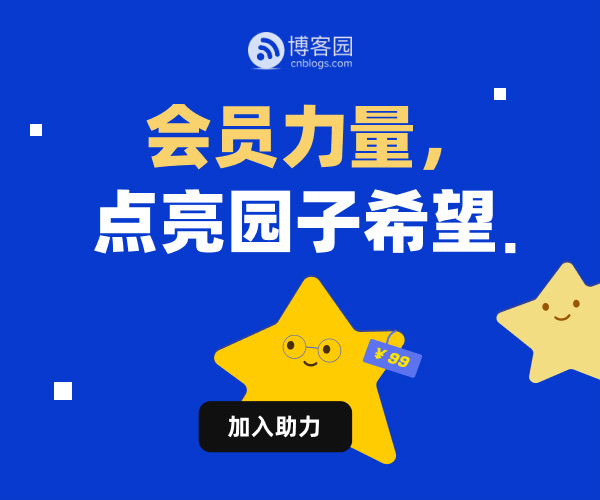