主要实现三个功能:显示备忘录,新建备忘录,备忘录的详细信息(可以显示图片)
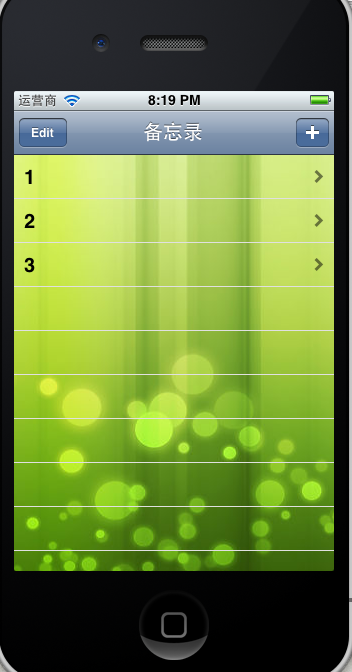
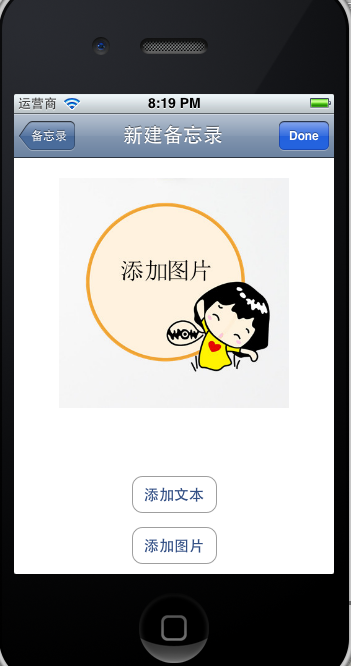
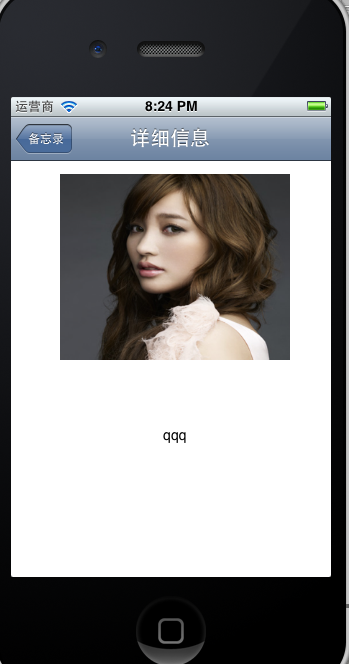
这个备忘录程序是一个很简单的小项目,不过里边包含了不少重要的知识,如TableView的用法,文本、图片的保存等。
新建“Empty Application” ,命名为MemorandumBook
新建三个UIViewController视图,分别命名为HomeViewController,AddViewController,DetailsViewController。三个视图分别来实现对应的三个功能。
1.在AppDelegate.m中添加代码:
(1)添加导航控制器
(2) 将homeViewController添加到导航器
(3) 将导航器添加到启动的主视图
self.window = [[[UIWindow alloc] initWithFrame:[[UIScreen mainScreen] bounds]] autorelease];
// Override point for customization after application launch.
self.window.backgroundColor = [UIColor whiteColor];
navigationController = [[UINavigationController alloc] init];
HomeViewController *homeViewController = [[HomeViewController alloc] init];
homeViewController.title = @"备忘录";
[navigationController pushViewController:homeViewController animated:NO];//压入栈
[self.window addSubview:navigationController.view];
[homeViewController release];
[self.window makeKeyAndVisible];
return YES;
}
2.
下面在HomeViewController视图,来实现上图的效果:
添加一个data.plist,存放备忘录
在该视图上添加一个TableView, 在HomeViewController上添加 NSMutableArray *booksArray 存放备忘录信息,添加 - (void)initData方法,来读取plist中的数据
// HomeViewController.h
#import <UIKit/UIKit.h>
@interface HomeViewController : UIViewController
@property (retain, nonatomic) IBOutlet UITableView *tableView;
@property (nonatomic, retain) NSMutableArray *booksArray;
- (void)initData;
- (void)didFinishAddingNewBook:(NSDictionary *)theBook;
@end
// HomeViewController.m
#import "HomeViewController.h"
#import "AddBookViewController.h"
#import "DetailsViewController.h"
@interface HomeViewController ()
@end
@implementation HomeViewController
@synthesize tableView;
@synthesize booksArray;
//数据显示到主视图中
- (void)initData
{
//读取data.plist数据
NSString *filePath = [[NSBundle mainBundle]pathForResource:@"data" ofType:@"plist"];
booksArray = [[NSMutableArray alloc]initWithContentsOfFile:filePath];
}
- (void)viewDidLoad
{
[super viewDidLoad];
//数据保存
/*booksArray是程序的数据源,在开发的时候,从data.plist中读出数据赋值给它。在程序结束之前,所有的更改都需要被保存到data.plist中去。要做到这点,可以让BookTableViewController在“感知”到程序快要结束时保存数据*/
//为对象添加一个“观察者”
UIApplication *app = [UIApplication sharedApplication];
[[NSNotificationCenter defaultCenter] addObserver:self selector:@selector(applicationWillTerminate:) name:UIApplicationWillTerminateNotification object:app];
[self initData];//显示数据
//左Bar
UIBarButtonItem *leftBarBtn = [[[UIBarButtonItem alloc] initWithBarButtonSystemItem:UIBarButtonSystemItemEdit target:self action:@selector(editAction:)] autorelease];
self.navigationItem.leftBarButtonItem = leftBarBtn;
//右Bar
UIBarButtonItem *rightBarBtn = [[[UIBarButtonItem alloc] initWithBarButtonSystemItem:UIBarButtonSystemItemAdd target:self action:@selector(addAction:)] autorelease];
self.navigationItem.rightBarButtonItem = rightBarBtn;
//self.view.backgroundColor =[UIColor colorWithPatternImage:[UIImage imageNamed:@"bg.png"]];
//设置背景
CGRect frame = self.view.bounds;
UIImageView *bg = [[UIImageView alloc] initWithFrame:frame];
bg.image = [UIImage imageNamed:@"bg.png"];
[self.view addSubview:bg];
[bg release];
self.tableView = [[UITableView alloc] initWithFrame:frame style:UITableViewStylePlain];
self.tableView.delegate = self;
self.tableView.dataSource = self;
self.tableView.backgroundColor = [UIColor clearColor];
self.tableView.indicatorStyle = UIScrollViewIndicatorStyleWhite;
[self.view addSubview:self.tableView];
}
- (void)addAction:(id)sender
{
//导航到添加视图
AddBookViewController *addBookViewController = [[AddBookViewController alloc] init];
addBookViewController.delegate = self;//添加完成后回调到本视图(BookViewController)的方法
[self.navigationController pushViewController:addBookViewController animated:YES];
[addBookViewController release];
}
- (void)editAction:(id)sender
{
[self.tableView setEditing:YES animated:YES];
UIBarButtonItem *leftBarBtn = [[[UIBarButtonItem alloc] initWithBarButtonSystemItem:UIBarButtonSystemItemDone target:self action:@selector(doneAction:)] autorelease];
self.navigationItem.leftBarButtonItem = leftBarBtn;
}
//又把视图的编辑转态改了回去,同时也把左按钮改了回去。
- (void)doneAction:(id)sender
{
[self.tableView setEditing:NO animated:YES];
UIBarButtonItem *leftBarBtn = [[[UIBarButtonItem alloc] initWithBarButtonSystemItem:UIBarButtonSystemItemEdit target:self action:@selector(editAction:)] autorelease];
self.navigationItem.leftBarButtonItem = leftBarBtn;
}
//表视图的委托方法
//指定单元格的个数
- (NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section
{
return [booksArray count];
}
//单元格对象
- (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath
{
static NSString *CellIdentifier = @"Cell";
UITableViewCell *cell = [tableView dequeueReusableCellWithIdentifier:CellIdentifier];
if (cell == nil)
{
cell = [[[UITableViewCell alloc] initWithStyle:UITableViewCellStyleDefault reuseIdentifier:CellIdentifier] autorelease];
}
cell.textLabel.text = [[booksArray objectAtIndex:indexPath.row] valueForKey:@"Text"];
cell.accessoryType = UITableViewCellAccessoryDisclosureIndicator;//单元格右边的显示为一个箭头,用以提示用户点击后展开
return cell;
}
//选中某行
- (void)tableView:(UITableView *)tableView didSelectRowAtIndexPath:(NSIndexPath *)indexPath
{
DetailsViewController *detailsViewController = [[DetailsViewController alloc] init];
detailsViewController.bookDictionary = [booksArray objectAtIndex:indexPath.row];//将选中的值传递到详细视图页
[self.navigationController pushViewController:detailsViewController animated:YES];//导航到详细视图
[detailsViewController release];
[tableView deselectRowAtIndexPath:indexPath animated:YES];
}
//编辑,删除
- (void)tableView:(UITableView *)tableView commitEditingStyle:(UITableViewCellEditingStyle)editingStyle forRowAtIndexPath:(NSIndexPath *)indexPath
{
if (editingStyle == UITableViewCellEditingStyleDelete)
{
//从数据源中删除该行
//删除图片
NSString *filePath = [[booksArray objectAtIndex:indexPath.row] valueForKey:@"Image"];
if ([[NSFileManager defaultManager] fileExistsAtPath:filePath])
{
[[NSFileManager defaultManager] removeItemAtPath:filePath error:nil];
}
[booksArray removeObjectAtIndex:indexPath.row];
[tableView deleteRowsAtIndexPaths:[NSArray arrayWithObject:indexPath] withRowAnimation:UITableViewRowAnimationRight];
}
else if (editingStyle == UITableViewCellEditingStyleInsert)
{
//创建相应类的实例,插入到数组中,并且向表中增加一行
}
}
- (void)didFinishAddingNewBook:(NSDictionary *)theBook
{
[booksArray addObject:theBook];
[self.tableView reloadData];
}
- (void)viewDidUnload
{
[super viewDidUnload];
}
//程序快要结束时调用
- (void)applicationWillTerminate:(UIApplication *)application
{
NSString *path = [[NSBundle mainBundle] pathForResource:@"data" ofType:@"plist"];//找到文件路径
[booksArray writeToFile:path atomically:YES];//写入
}
- (BOOL)shouldAutorotateToInterfaceOrientation:(UIInterfaceOrientation)interfaceOrientation
{
return (interfaceOrientation == UIInterfaceOrientationPortrait);
}
- (void)dealloc {
[tableView release];
[super dealloc];
}
@end
3.
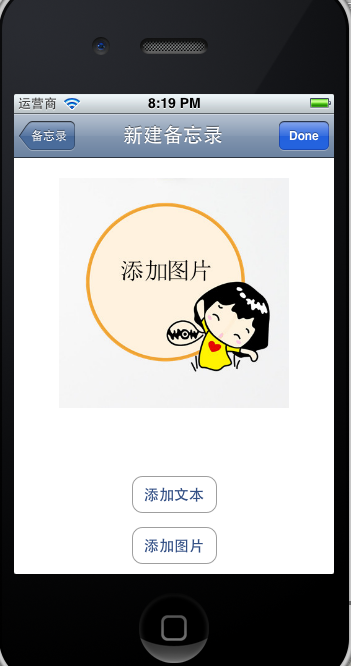
下面来实现AddViewController视图:
在xib上添加ImageView,Label,2个Button,如上图布局。
Button“添加文本”的方法:
用到UITextViewDelegate,UINavigationControllerDelegate,UIImagePickerControllerDelegate,UIActionSheetDelegate 等协议
UIBarButtonItem *rightBarBtn = [[[UIBarButtonItem alloc] initWithBarButtonSystemItem:UIBarButtonSystemItemDone target:self action:@selector(doneEditing:)] autorelease];
self.navigationItem.rightBarButtonItem = rightBarBtn;
textView = [[UITextView alloc] initWithFrame:CGRectMake(0, -240, 320, 240)];
textView.text = textLabel.text;
[self.view addSubview:textView];
[UIView beginAnimations:@"pushAnimation" context:textView];
[UIView setAnimationCurve:0.2];
[textView setFrame:CGRectMake(0, 0, 320, 240)];
[UIView commitAnimations];
[textView becomeFirstResponder];
}
//完成编辑
- (void)doneEditing:(id)sender
{
//textView弹回,取消其第一响应者身份(键盘弹回)
[textView resignFirstResponder];
[UIView beginAnimations:@"popAnimation" context:textView];
[UIView setAnimationDuration:0.2];
[textView setFrame:CGRectMake(0, -240, 320, 240)];
[UIView commitAnimations];
[textLabel setText:textView.text];
//右bar恢复“done”
UIBarButtonItem *rightBarBtn = [[[UIBarButtonItem alloc] initWithBarButtonSystemItem:UIBarButtonSystemItemDone
target:self
action:@selector(doneAction:)] autorelease];
self.navigationItem.rightBarButtonItem = rightBarBtn;
}
Button“添加图片”的方法:
cancelButtonTitle:@"取消"
destructiveButtonTitle:@"拍照"
otherButtonTitles:@"选择照片", nil];
[actionSheet showInView:[[UIApplication sharedApplication] keyWindow]];
}
//UIImagePickerControllerDelegate的委托方法
- (void)imagePickerController:(UIImagePickerController *)picker didFinishPickingMediaWithInfo:(NSDictionary *)info
{
image = [info objectForKey:UIImagePickerControllerEditedImage];
[imageView setImage:image];
[picker dismissModalViewControllerAnimated:YES];
}
- (void)imagePickerControllerDidCancel:(UIImagePickerController *)picker
{
[picker dismissModalViewControllerAnimated:YES];
[[picker parentViewController] dismissModalViewControllerAnimated:YES];
}
- (void)actionSheet:(UIActionSheet *)actionSheet didDismissWithButtonIndex:(NSInteger)buttonIndex
{
UIImagePickerController *imagePicker = [[[UIImagePickerController alloc] init] autorelease];
imagePicker.allowsImageEditing = YES;
if (buttonIndex == 0)
{
if ([UIImagePickerController isSourceTypeAvailable:UIImagePickerControllerSourceTypeCamera])
{
//imagePicker.sourceType = UIImagePickerControllerSourceTypeCamera;
}
else
{
UIAlertView *alert = [[UIAlertView alloc] initWithTitle:@"没有相机"
message:nil
delegate:nil
cancelButtonTitle:@"关闭"
otherButtonTitles:nil];
[alert show];
[alert release];
return;
}
}
else if (buttonIndex == 1)
{
//imagePicker.sourceType = UIImagePickerControllerSourceTypePhotoLibrary;
}
else if (buttonIndex == 2)
{
return;
}
imagePicker.delegate = self;
[self presentModalViewController:imagePicker animated:YES];
}
- (void)doneAction:(id)sender
{
NSArray *paths = NSSearchPathForDirectoriesInDomains(NSCachesDirectory, NSUserDomainMask, YES);
NSString *filePath = [paths objectAtIndex:0];
NSString *fileName = [NSString stringWithFormat:@"%d.png",random()];
filePath = [filePath stringByAppendingPathComponent:fileName];
//NSData *imageData = UIImageJPEGRepresentation(image,1.0f);//取1.0代表最佳品质
NSData *imageData = UIImagePNGRepresentation(image);//取1.0代表最佳品质
if (imageData)
{
[[NSFileManager defaultManager] createFileAtPath:filePath contents:imageData attributes:nil];
}
else
{
UIAlertView *alert = [[UIAlertView alloc] initWithTitle:nil
message:@"请选择照片"
delegate:nil
cancelButtonTitle:@"确认"
otherButtonTitles:nil];
[alert show];
[alert release];
return;
}
if ([textLabel.text isEqualToString:@""])
{
UIAlertView *alert = [[UIAlertView alloc] initWithTitle:nil message:@"请输入备忘" delegate:nil cancelButtonTitle:@"确认" otherButtonTitles:nil];
[alert show];
[alert release];
return;
}
NSDictionary *newBook = [[NSDictionary alloc] initWithObjectsAndKeys:textLabel.text, @"Text", filePath, @"Image", nil];
[delegate performSelector:@selector(didFinishAddingNewBook:) withObject:newBook];
[self.navigationController popViewControllerAnimated:YES];
}
4.
下面来实现DetailsViewController视图:
这个只需要将文本和图片读出来,所以比较简单
// DetailsViewController.m
#import "DetailsViewController.h"
@interface DetailsViewController ()
@end
@implementation DetailsViewController
@synthesize imageView;
@synthesize textView;
@synthesize bookDictionary;
- (void)viewDidLoad
{
[super viewDidLoad];
self.navigationItem.title = @"详细信息";
UIImage *image = [[UIImage alloc] initWithContentsOfFile:[bookDictionary valueForKey:@"Image"]];
if (image)
{
[imageView setImage:image];
}
[image release];
textView.text = [bookDictionary valueForKey:@"Text"];
}
- (void)viewDidUnload
{
[self setTextView:nil];
[self setImageView:nil];
[super viewDidUnload];
// Release any retained subviews of the main view.
// e.g. self.myOutlet = nil;
}
- (BOOL)shouldAutorotateToInterfaceOrientation:(UIInterfaceOrientation)interfaceOrientation
{
return (interfaceOrientation == UIInterfaceOrientationPortrait);
}
- (void)dealloc {
[textView release];
[imageView release];
[super dealloc];
}
@end