C#设置开机启动
原理就是在注册表启动项里添加一项。
路径:SOFTWARE\Microsoft\Windows\CurrentVersion\Run
或者直接:运行->regedit找到这个路径添加一项。
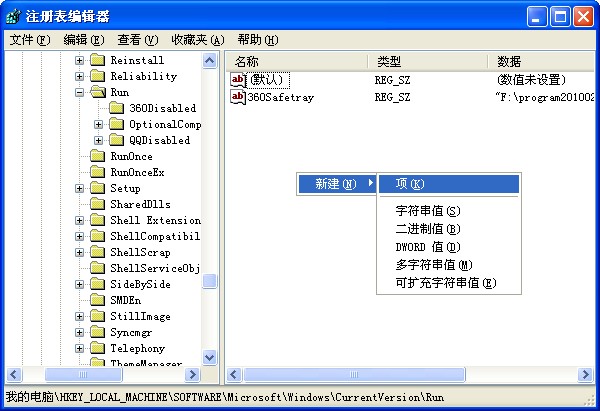
url:http://greatverve.cnblogs.com/archive/2012/02/18/csharp-autorun.html
参考:
路径:SOFTWARE\Microsoft\Windows\CurrentVersion\Run
或者直接:运行->regedit找到这个路径添加一项。
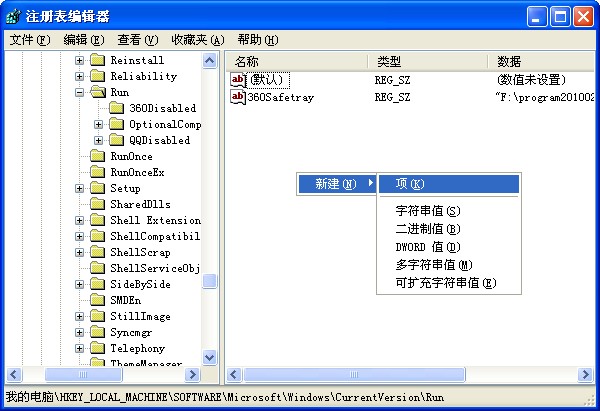
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using Microsoft.Win32;
namespace CSharpStart
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void btnSet_Click(object sender, EventArgs e)
{
SetAutoRun(@"D:\CSharpStart.exe",true);
}
/// <summary>
/// 设置应用程序开机自动运行
/// </summary>
/// <param name="fileName">应用程序的文件名</param>
/// <param name="isAutoRun">是否自动运行,为false时,取消自动运行</param>
/// <exception cref="System.Exception">设置不成功时抛出异常</exception>
public static void SetAutoRun(string fileName, bool isAutoRun)
{
RegistryKey reg = null;
try
{
if (!System.IO.File.Exists(fileName))
throw new Exception("该文件不存在!");
String name = fileName.Substring(fileName.LastIndexOf(@"\") + 1);
reg = Registry.LocalMachine.OpenSubKey(@"SOFTWARE\Microsoft\Windows\CurrentVersion\Run", true);
if (reg == null)
reg = Registry.LocalMachine.CreateSubKey(@"SOFTWARE\Microsoft\Windows\CurrentVersion\Run");
if (isAutoRun)
reg.SetValue(name, fileName);
else
reg.SetValue(name, false);
}
catch (Exception ex)
{
throw new Exception(ex.ToString());
}
finally
{
if (reg != null)
reg.Close();
}
}
//另外也可以写成服务,不过服务的话一般是在后台执行的,没有程序界面。
}
}
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using Microsoft.Win32;
namespace CSharpStart
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void btnSet_Click(object sender, EventArgs e)
{
SetAutoRun(@"D:\CSharpStart.exe",true);
}
/// <summary>
/// 设置应用程序开机自动运行
/// </summary>
/// <param name="fileName">应用程序的文件名</param>
/// <param name="isAutoRun">是否自动运行,为false时,取消自动运行</param>
/// <exception cref="System.Exception">设置不成功时抛出异常</exception>
public static void SetAutoRun(string fileName, bool isAutoRun)
{
RegistryKey reg = null;
try
{
if (!System.IO.File.Exists(fileName))
throw new Exception("该文件不存在!");
String name = fileName.Substring(fileName.LastIndexOf(@"\") + 1);
reg = Registry.LocalMachine.OpenSubKey(@"SOFTWARE\Microsoft\Windows\CurrentVersion\Run", true);
if (reg == null)
reg = Registry.LocalMachine.CreateSubKey(@"SOFTWARE\Microsoft\Windows\CurrentVersion\Run");
if (isAutoRun)
reg.SetValue(name, fileName);
else
reg.SetValue(name, false);
}
catch (Exception ex)
{
throw new Exception(ex.ToString());
}
finally
{
if (reg != null)
reg.Close();
}
}
//另外也可以写成服务,不过服务的话一般是在后台执行的,没有程序界面。
}
}
参考:
C# winform程序设置开机启动,当读取配置文件,或者加载图片如果设置的是相对路径时,开机启动时会出现问题(直接运程程序是没问题的)。这是因为开机启动的程序要使用绝对路径,相对路径不行。我们可以通过Application .StartupPath属性经过处理得到文件的绝对路径问题就解决了。
C# 通过读写注册表来设置开机启动想方法很简单,网上很多:
/// <summary>
/// 开机启动项
/// </summary>
/// <param name="Started">是否启动</param>
/// <param name="name">启动值的名称</param>
/// <param name="path">启动程序的路径</param>
public void RunWhenStart(bool Started, string name, string path)
{
RegistryKey HKLM = Registry.LocalMachine;
RegistryKey Run = HKLM.CreateSubKey(@"SOFTWARE/Microsoft/Windows/CurrentVersion/Run");
if (Started == true)
{
try
{
Run.SetValue(name, path);
HKLM.Close();
}
catch//没有权限会异常
{ }
}
else
{
try
{
Run.DeleteValue(name);
HKLM.Close();
}
catch//没有权限会异常
{ }
}
}
/// 开机启动项
/// </summary>
/// <param name="Started">是否启动</param>
/// <param name="name">启动值的名称</param>
/// <param name="path">启动程序的路径</param>
public void RunWhenStart(bool Started, string name, string path)
{
RegistryKey HKLM = Registry.LocalMachine;
RegistryKey Run = HKLM.CreateSubKey(@"SOFTWARE/Microsoft/Windows/CurrentVersion/Run");
if (Started == true)
{
try
{
Run.SetValue(name, path);
HKLM.Close();
}
catch//没有权限会异常
{ }
}
else
{
try
{
Run.DeleteValue(name);
HKLM.Close();
}
catch//没有权限会异常
{ }
}
}
或者直接:
- //添加启动
- RegistryKey ms_run = Registry.LocalMachine.OpenSubKey("SOFTWARE//Microsoft//Windows//CurrentVersion//Run", true);
- ms_run.SetValue("mistysoft", Application.ExecutablePath.ToString());
- //删除启动(设为控,注册表项还在)
- RegistryKey ms_run = Registry.LocalMachine.OpenSubKey("SOFTWARE//Microsoft//Windows//CurrentVersion//Run", true);
- ms_run.SetValue("mistysoft", "");
我这个博客废弃不用了,今天想寻找外链的时候,突然想到这个博客权重很高。
有需要免费外链的,留言即可,我准备把这个博客变成免费的友情链接站点。