Selenium2学习-017-WebUI自动化实战实例-015-获取浏览器中的 cookie 信息
日常我们在使用浏览器时,尤其是登录 WEB 应用时,我们的一些信息其实是保存在了浏览器的 cookie 信息中。我们可以通过浏览器自带的开发工具,进行查看相应的 cookie 信息,例如在火狐、chrome 中均可通过 F12 打开开发者工具打开。以下截图为在 chrome 中打开易迅网时的 cookie 信息:
若是小主您登录了相应的网页应用,cookie 中还会含有您的一些用户信息内容。那么这些 cookie 信息在我们日常 WebUI 自动化脚本的编写过程中有什么用途呢 ? 可以作为网页打开是否正确的校验依据,可以作为用户是否正确登录的判定依据等等。那么我们如何获取相应的 cookie 信息呢,以下自动化测试脚本以易迅网易迅账号登录获取其 cookie 给出了明确的实例演示,其他网站的登录 cookie 信息获取相同。
闲话少述,小二上码。。。敬请各位小主参阅,希望能对您在日常的 WebUI 自动化脚本编写有一定的启发和帮助。若有不足或错误之处,敬请大神指正,不胜感激!

1 /** 2 * Aaron.ffp Inc. 3 * Copyright (c) 2004-2015 All Rights Reserved. 4 */ 5 package main.aaron.demo.cookie; 6 7 import java.io.BufferedWriter; 8 import java.io.File; 9 import java.io.FileWriter; 10 import java.util.concurrent.TimeUnit; 11 12 import javax.swing.JDialog; 13 import javax.swing.JOptionPane; 14 import javax.swing.JPasswordField; 15 16 import org.openqa.selenium.By; 17 import org.openqa.selenium.Cookie; 18 import org.openqa.selenium.WebElement; 19 import org.testng.annotations.AfterClass; 20 import org.testng.annotations.Test; 21 22 import main.aaron.sele.core.TestCase; 23 24 /** 25 * get cookie info by manual login 26 * 27 * @author Aaron.ffp 28 * @version V1.0.0: autoSeleniumDemo main.aaron.demo.cookie Cookies.java, 2015-6-19 13:53:05 Exp $ 29 */ 30 public class Cookies extends TestCase{ 31 private final String f_cookie = this.PROJECTHOME + this.FILESEPARATOR + "cookie" + this.FILESEPARATOR + "browser.data"; 32 private String baseUrl = ""; 33 File file; 34 35 @Test 36 public void addCookie(){ 37 this.baseUrl = "https://base.yixun.com/login.html?url=http%3A%2F%2Fbase.yixun.com%2F"; 38 39 // wait ten seconds 40 this.webdriver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS); 41 42 // navigate to login page 43 this.webdriver.get(this.baseUrl); 44 45 // change to login frame 46 this.webdriver.switchTo().frame("id_iframe_login"); 47 48 // click yixun_login, change to yixun login tab 49 WebElement yx_login = this.webdriver.findElement(By.cssSelector(".fast_yx")); 50 yx_login.click(); 51 52 // this.webdriver.switchTo().frame("id_iframe_login"); 53 54 WebElement user = this.webdriver.findElement(By.id("yxname")); 55 WebElement pass = this.webdriver.findElement(By.id("yxpw")); 56 WebElement code = this.webdriver.findElement(By.id("yxcode")); 57 WebElement subm = this.webdriver.findElement(By.id("submit-button")); 58 59 // clean the input dialog 60 user.clear(); 61 pass.clear(); 62 code.clear(); 63 64 // input login info 65 user.sendKeys("请小主们自行注册易迅网易迅账号"); 66 pass.sendKeys("请小主们自行注册易迅网易迅账号"); 67 code.sendKeys(this.inputDialog()); 68 69 // click login button 70 subm.click(); 71 72 this.file = new File(this.f_cookie); 73 74 // store login cookie 75 try { 76 Thread.sleep(15000); 77 78 this.file.delete(); 79 80 this.file.createNewFile(); 81 82 FileWriter fw = new FileWriter(this.f_cookie); 83 84 BufferedWriter bw = new BufferedWriter(fw); 85 86 for (Cookie cookie : this.webdriver.manage().getCookies()) { 87 bw.write(cookie.getName() + ";" + 88 ("".equals(cookie.getValue()) || (cookie.getValue() == null) ? "": cookie.getValue()) + ";" + 89 cookie.getDomain() + ";" + 90 cookie.getPath() + ";" + 91 cookie.getExpiry() + ";" + 92 cookie.isSecure()); 93 bw.newLine(); 94 } 95 96 bw.flush(); 97 bw.close(); 98 fw.close(); 99 } catch (Exception e) { 100 e.printStackTrace(); 101 } finally { 102 System.out.println("cookie write to file : " + this.f_cookie); 103 } 104 } 105 106 /** 107 * input dialog 108 * 109 * @author Aaron.ffp 110 * @version V1.0.0: autoSeleniumDemo main.aaron.demo.cookie Cookies.java inputDialog, 2015-6-19 13:55:05 Exp $ 111 * 112 * @return 113 */ 114 public String inputDialog() { 115 JPasswordField pass = new JPasswordField(30); 116 JOptionPane id = new JOptionPane("Please input verify code...", JOptionPane.PLAIN_MESSAGE); 117 118 id.add(pass); 119 120 pass.setEchoChar('*'); 121 JDialog inputPassDialog = id.createDialog(id, "inputdialog"); 122 123 inputPassDialog.setVisible(true); 124 125 String inStr = String.valueOf(pass.getPassword()); 126 return inStr; 127 } 128 129 @AfterClass 130 public void afterClass(){ 131 this.webdriver.close(); 132 this.webdriver.quit(); 133 } 134 }
注意:在实际的使用中, 小主们需要将 cookie 文件保存的位置修改为本地的合法路径才可,对应代码如下:
private final String f_cookie = this.PROJECTHOME + this.FILESEPARATOR + "cookie" + this.FILESEPARATOR + "browser.data";
至此,WebUI 自动化功能测试脚本第 015-获取浏览器中的 cookie 信息 顺利完结,希望此文能够给初学 Selenium 的您一份参考。
最后,非常感谢亲的驻足,希望此文能对亲有所帮助。热烈欢迎亲一起探讨,共同进步。非常感谢! ^_^
欢迎 【 留言 || 关注 || 打赏 】 。您的每一份心意都是对我的鼓励和支持!非常感谢!欢迎互加,相互交流学习!
作者:范丰平,本文链接:https://www.cnblogs.com/fengpingfan/p/4593283.html
Copyright @范丰平 版权所有,如需转载请标明本文原始链接出处,严禁商业用途! 我的个人博客链接地址:http://www.cnblogs.com/fengpingfan
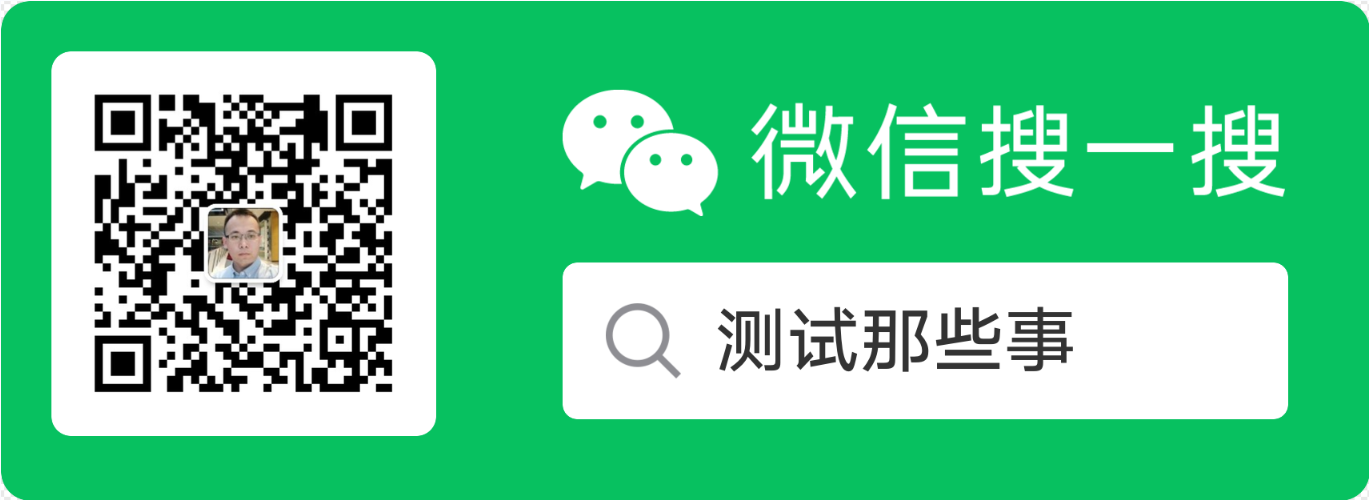