Spring MVC Introduction
Excerpted from <<Pro Spring 3.0>> Chapter 17
Introducing MVC
Figure 17-1 illustrates a commonly used web application pattern, which can be treated as an enhancement to the traditional MVC pattern. A normal view request is handled as follows:
1. Request: A request is submitted to the server. On the server side, most frameworks (for example, Spring MVC, Struts, and so on) will have a dispatcher (in the form of a servlet) to handle the request.
2. Invokes: The dispatcher dispatches the request to the appropriate controller based on the HTTP request information and the web application configuration.
3. Service Call: The controller interacts with the service layer.
4. Response: The controller updates the model and, based on the execution result, returns the corresponding view to the user.
In addition, within a view, Ajax calls will happen. For example, say the user is browsing data within a grid. When the user clicks the next page, instead of a full page refresh, the following flow will happen:
1. Request: An XMLHttpRequest is prepared and submitted to the server. The dispatcher will dispatch the request to the corresponding controller.
2. Response: The controller interacts with the service layer, and the response data will be formatted and sent to the browser. No view is involved in this case. The browser receives the data and performs a partial update of the existing view.
In the Spring Framework, the Spring MVC module provides comprehensive support for the MVC pattern, with support for other features (for example, theming, i18n, validation, type conversion and
formatting, and so on) that ease the implementation of the presentation layer.
In the following sections, we will discuss the main concepts of Spring MVC. Topics include Spring MVC’s WebApplicationContext hierarchy, a typical request-handling life cycle, and configuration.
Spring MVC WebApplicationContext Hierarchy
In Spring MVC, the DispatcherServlet is the central servlet that receives requests and dispatches them to the appropriate controllers. In a Spring MVC application, there can be any number of DispatcherServlets for various purposes (for example, handling user interface requests, RESTful-WS requests, and so on), and each DispatcherServlet has its own WebApplicationContext configuration, which defines the servlet-level characteristics, such as controllers supporting the servlet, handler mapping, view resolving, i18n, theming, validation, type conversion and formatting, and so on.
Underneath the servlet-level WebApplicationContext configurations, Spring MVC also maintains a root WebApplicationContext, which includes the application-level configurations such as backend data source, security, service and persistence layer configuration, and so on. The root WebApplicationContext will be available to all servlet-level WebApplicationContext.
Let’s look at an example. Let’s say in an application we have two DispatcherServlets. One servlet is to support the user interface (we call it the application servlet), and the other is to provide services in the form of RESTful-WS to other applications (we call it the RESTful servlet). In Spring MVC, we will define the configurations for both the root WebApplicationContext and the WebApplicationContext for the two DispatcherServlets.
Figure 17-2 shows the WebApplicationContext hierarchy that will be maintained by Spring MVC for this scenario.
Spring MVC Request Life Cycle
Let’s see how Spring MVC handles a request. Figure 17-3 shows the main components involved in handling a request in Spring MVC. The figure is based on the one described in the Spring Framework
forum (http://forum.springsource.org/showthread.php?21639-Spring-MVC-Request-Lifecycle-Diagram), with modifications.
Figure 17-3. Spring MVC request life cycle
The main components and their purposes are as follows:
• Filter: The filter applies to every request. Several commonly used filters and their purposes are described in the next section.
• Dispatcher servlet: The servlet analyzes the requests and dispatches them to the appropriate controller for processing.
• Common services: The common services will apply to every request to provide supports including i18n, theme, file upload, and so on. Their configuration is defined in the DispatcherServlet’s WebApplicationContext.
• Handler mapping: This maps the request to the handler (a method within a Spring MVC controller class). Since Spring 2.5, in most situations the configuration is not
required because Spring MVC will automatically register the org.springframework.web.servlet.mvc.annotation.DefaultAnnotationHandlerMapping class that maps handlers based on HTTP paths expressed through the @RequestMapping annotation at the type or method level within controller classes.
• Handler interceptor: In Spring MVC, you can register interceptors for the handlers for implementing common checking or logic. For example, a handler interceptor can check and ensure that only the handlers can be invoked during office hours.
• Handler exception resolver: In Spring MVC, the HandlerExceptionResolver interface (under the package org.springframework.web.servlet) is designed to deal with unexpected exceptions thrown during request processing by handlers.
By default, the DispatcherServlet registers the DefaultHandlerExceptionResolver class (under the package org.springframework.web.servlet.mvc.support). This resolver handles certain standard Spring MVC exceptions by setting a specific response status code. You can also implement your own exception handler by annotating a controller method with the @ExceptionHandler annotation and passing in the exception type as the attribute.
• View Resolver: Spring MVC’s ViewResolver interface (under the package org.springframework.web.servlet) supports view resolution based on a logical name returned by the controller. There are many implementation classes to support various view resolving mechanisms. For example, the UrlBasedViewResolver class supports direct resolution of logical names to URLs. The ContentNegotiatingViewResolver class supports dynamic resolving of views depending on the media type supported by the client (such as XML, PDF, JSON, and so on). There also exists a number of implementations to integrate with different view technologies, such as FreeMarker (FreeMarkerViewResolver), Velocity (VelocityViewResolver), and JasperReports (JasperReportsViewResolver).
These descriptions cover only a few commonly used handlers and resolvers. For a full description, please refer to the Spring Framework reference documentation and its Javadoc.
Spring MVC Configuration
To enable Spring MVC within a web application, some initial configuration is required, especially for the web deployment descriptor web.xml, residing in the folder /src/main/webapp/WEB-INF (Spring 3.1 introduced a new feature that supports code-based configuration within a Servlet 3.0 web container, which we will discuss in “Support for Servlet 3 Code-Based Configuration” in this chapter).
To configure Spring MVC support for web applications, we need to perform the following configurations in the web deployment descriptor:
• Configuring the root WebApplicationContext
• Configuring the servlet filters required by Spring MVC
• Configuring the dispatcher servlets within the application
<?xml version="1.0" encoding="UTF-8"?> <web-app version="3.0" xmlns="http://java.sun.com/xml/ns/javaee" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd"> <!-- The definition of the Root Spring Container shared by all Servlets and Filters --> <context-param> <param-name>contextConfigLocation</param-name> <param-value>/WEB-INF/spring/root-context.xml</param-value> </context-param> <!-- Spring MVC filters --> <filter> <filter-name>CharacterEncodingFilter</filter-name> <filter-class> org.springframework.web.filter.CharacterEncodingFilter </filter-class> <init-param> <param-name>encoding</param-name> <param-value>UTF-8</param-value> </init-param> <init-param> <param-name>forceEncoding</param-name> <param-value>true</param-value> </init-param> </filter> <filter> <filter-name>HttpMethodFilter</filter-name> <filter-class> org.springframework.web.filter.HiddenHttpMethodFilter </filter-class> </filter> <filter> <filter-name>Spring OpenEntityManagerInViewFilter</filter-name> <filter-class> org.springframework.orm.jpa.support.OpenEntityManagerInViewFilter </filter-class> </filter> <filter-mapping> <filter-name>CharacterEncodingFilter</filter-name> <url-pattern>/*</url-pattern> </filter-mapping> <filter-mapping> <filter-name>HttpMethodFilter</filter-name> <url-pattern>/*</url-pattern> </filter-mapping> <filter-mapping> <filter-name>Spring OpenEntityManagerInViewFilter</filter-name> <url-pattern>/*</url-pattern> </filter-mapping> <!-- Creates the Spring Container shared by all Servlets and Filters --> <listener> <listener-class> org.springframework.web.context.ContextLoaderListener </listener-class> </listener> <!-- Processes application requests --> <servlet> <servlet-name>appServlet</servlet-name> <servlet-class> org.springframework.web.servlet.DispatcherServlet </servlet-class> <init-param> <param-name>contextConfigLocation</param-name> <param-value> /WEB-INF/spring/appServlet/servlet-context.xml </param-value> </init-param> <load-on-startup>1</load-on-startup> </servlet> <servlet-mapping> <servlet-name>appServlet</servlet-name> <url-pattern>/</url-pattern> </servlet-mapping> </web-app>
The main points for Listing 17-9 are as follows:
• In the <web-app> tag, the version attribute and the corresponding URL are changed to version 3.0 to indicate to the web container that the web application will use Servlet 3.0.
• In the <context-param> tag, the contextConfigLocation param is provided, which defines the location of Spring’s root WebApplicationContext configuration file.
• A number of servlet filters provided by Spring MVC are defined, and all filters are mapped to the web application root context URL. Those filters are commonly used in web applications. Table 17-1 shows the filters configured and their purpose.
• A listener of class org.springframework.web.context.ContextLoaderListener is defined. This is for Spring to bootstrap and shut down the root WebApplicationContext.
• One dispatcher servlet (called appServlet) is defined. We use the one generated by the template project for the contact application’s presentation layer. The WebApplicationContext for the dispatcher servlet is located at
/src/main/webapp/WEB-INF/spring/appServlet/servlet-context.xml.
Table 17-1. Commonly used Spring MVC Servlet Filters
Filter Class Name |
Description |
org.springframework.web.filter.Chara |
This filter is used to specify the character encoding for |
org.springframework.web.filter.Hidde |
This filter provides support for HTTP methods other than |
org.springframework.orm.jpa.support. |
This filter binds the JPA EntityManager to the thread for the |
--------------------------------------
Regards,
FangwenYu
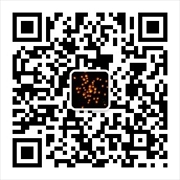