用原生JS进行CSS格式化和压缩
前言
一直比较喜欢收集网页特效,很多时候都会遇到CSS被压缩过的情况,这时查看起来就会非常不方便,有时为了减少文件大小,也会对自己的CSS进行压缩,网上提供这样服务的很多,但都不尽如人意,因此打算自己动手写一个JS来进行CSS的格式化和压缩
原理
CSS的结构如下:
选择器{
css属性声明:值;
}
所以对CSS格式化也就比较简单,大致分为以下几步;
1、把多个空格合并成一个,去掉换行
2、对处理后的字符串按"{"进行分组
3、遍历分组,对含有"}"的部分再次以"}"进行分组
4、对分组后的数据进行处理,主要是加上空格和换行
对CSS压缩就比较简单了,把空格合并,去掉换行就可以了
格式化
下面分步对以上步骤进行实现。
初始化:
function formathtmljscss(source, spaceWidth, formatType) {
this.source = source;
this.spaceStr = " ";
if (!isNaN(spaceWidth)) {
if (spaceWidth > 1) {
this.spaceStr = "";
for (var i = 0; i < spaceWidth; i++) {
this.spaceStr += " ";
}
}
else {
this.spaceStr = "\t";
}
}
this.formatType = formatType;
this.output = [];
}
这里几个参数分别是要格式化的CSS代码、CSS属性声明前空格宽度,类型(格式化/压缩)
1、把多个空格合并成一个,去掉换行:
formathtmljscss.prototype.removeSpace = function () {
this.source = this.source.replace(/\s+|\n/g, " ")
.replace(/\s*{\s*/g, "{")
.replace(/\s*}\s*/g, "}")
.replace(/\s*:\s*/g, ":")
.replace(/\s*;\s*/g, ";");
}
2、对处理后的字符串按"{"进行分组
formathtmljscss.prototype.split = function () {
var bigqleft = this.source.split("{");
}
3、遍历分组,对含有"}"的部分再次以"}"进行分组
formathtmljscss.prototype.split = function () {
var bigqleft = this.source.split("{");
var bigqright;
for (var i = 0; i < bigqleft.length; i++) {
if (bigqleft[i].indexOf("}") != -1) {
bigqright = bigqleft[i].split("}");
}
else {
}
}
}
4、对分组后的数据进行处理,主要是加上空格和换行
这里的处理主要分为,把CSS属性声明和值部分取出来,然后加上空格和换行:
formathtmljscss.prototype.split = function () {
var bigqleft = this.source.split("{");
var bigqright;
for (var i = 0; i < bigqleft.length; i++) {
if (bigqleft[i].indexOf("}") != -1) {
bigqright = bigqleft[i].split("}");
var pv = bigqright[0].split(";");
for (var j = 0; j < pv.length; j++) {
pv[j] = this.formatStatement(this.trim(pv[j]),true);
if (pv[j].length > 0) {
this.output.push(this.spaceStr + pv[j] + ";\n");
}
}
this.output.push("}\n");
bigqright[1] = this.trim(this.formatSelect(bigqright[1]));
if (bigqright[1].length > 0) {
this.output.push(bigqright[1], " {\n");
}
}
else {
this.output.push(this.trim(this.formatSelect(bigqleft[i])), " {\n");
}
}
}
这里调用了几个方法:trim、formatSelect、formatStatement,下面一一说明。
trim:从命名就可以看出是去除首尾空格;
formathtmljscss.prototype.trim = function (str) {
return str.replace(/(^\s*)|(\s*$)/g, "");
}
formatSelect:是处理选择器部分语法,做法就是给"."前面加上空格,把","前后的空格去掉,把多个空格合并为一个:
formathtmljscss.prototype.formatSelect = function (str) {
return str.replace(/\./g, " .")
.replace(/\s+/g, " ")
.replace(/\. /g, ".")
.replace(/\s*,\s*/g, ",");
}
formatStatement:是处理“css属性声明:值;”部分的语法,做法就是给":"后面加上空格,把多个空格合并为一个,去掉“#”后面的空格,去掉"px"前面的空格,去掉"-"两边的空格,去掉":"前面的空格:
formathtmljscss.prototype.formatStatement = function (str, autoCorrect) {
str = str.replace(/:/g, " : ")
.replace(/\s+/g, " ")
.replace("# ", "#")
.replace(/\s*px/ig, "px")
.replace(/\s*-\s*/g, "-")
.replace(/\s*:/g, ":");
return str;
}
调用
调用部分比较简单,对于格式化来说就是去掉空格和换行,然后分组处理,对于压缩来说就是去掉空格和换行:
formathtmljscss.prototype.formatcss = function () {
if (this.formatType == "compress") {
this.removeSpace();
}
else {
this.removeSpace();
this.split();
this.source = this.output.join("");
}
}
界面HTML代码:

<div id="content">
<div class="container">
<div class="box">
<div class="main">
<h2>CSS格式化/压缩</h2>
<div id="blurb">
<fieldset id="options">
<button id="submit">
<span>格式化 / 压缩 <img alt="格式化" src="/images/29.png"/></span>
</button><br/>
<span>缩进:</span>
<ul>
<li>
<select name="tabsize" id="tabsize">
<option value="1">tab键缩进</option>
<option value="2">2空格缩进</option>
<option selected="selected" value="4">4空格缩进</option>
</select>
</li>
</ul><br />
<span>类型:</span><br />
<input type="radio" name="format_type" value="format" checked="checked" id="format_format" /><label for="format_format">格式化</label>
<input type="radio" name="format_type" value="compress" id="format_compress" /><label for="format_compress">压缩</label>
</fieldset>
</div>
<div id="beauty">
<fieldset id="textarea-wrap">
<textarea rows="20" cols="40" id="source"></textarea>
</fieldset>
</div>
</div>
</div>
</div>
</div>
跟页面元素按钮绑定事件:

window.onload = function () {
var submitBtn = document.getElementById("submit");
var tabsize = document.getElementById("tabsize");
var sourceCon = document.getElementById("source");
var size = 4;
var formatType = "format";
submitBtn.onclick = function () {
var radios = document.getElementsByName("format_type");
for (i = 0; i < radios.length; i++) {
if (radios[i].checked) {
formatType = radios[i].value;
break;
}
}
var format = new formathtmljscss(sourceCon.value, size, formatType);
format.formatcss();
sourceCon.value = format.source;
}
tabsize.onchange = function () {
size = this.options[this.options.selectedIndex].value;
submitBtn.click();
return false;
}
}
演示
欢迎大家测试挑刺哈!
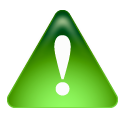