用jersey写简单Restful接口
1.在myeclipse中新建一个Dynamic Web Project
2.下载jar包,地址在这里
3.restful service代码
package com.qy; import javax.ws.rs.GET; import javax.ws.rs.Path; import javax.ws.rs.PathParam; import javax.ws.rs.Produces; import javax.ws.rs.core.MediaType; //这里@Path定义了类的层次路径。 //指定了资源类提供服务的URI路径。 @Path("userService") public class HelloRestFul { private String name= ""; @GET //get请求 @Path("/hello/{name}") //指定了资源类提供服务的URI路径。 @Produces(MediaType.TEXT_XML) //返回xml类型 public String sayHello(@PathParam("name") String name){ this.name = name; System.out.println("Hello "+this.name); return "<root>" + "<Say>" + "Hello "+name + "</Say>" + "</root>"; } @GET @Path("/bye/{name}") @Produces(MediaType.TEXT_XML) public String bye(@PathParam("name") String name) { System.out.println("Bye "+ name); return "<root>" + "<Say>" + "bye "+name + "</Say>" + "</root>"; } }
4.在web.xml中配置
<?xml version="1.0" encoding="UTF-8"?> <web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd" version="3.0"> <display-name>RESTful</display-name> <servlet> <servlet-name>Jersey REST Service</servlet-name> <servlet-class>com.sun.jersey.spi.container.servlet.ServletContainer</servlet-class> <!--restful容器类--> <init-param> <param-name>com.sun.jersey.config.property.packages</param-name> <!--自动加载的包的参数--> <param-value>com.qy</param-value> <!--restful自动加载指定包下的controller--> </init-param> <load-on-startup>1</load-on-startup> </servlet> <servlet-mapping> <servlet-name>Jersey REST Service</servlet-name> <url-pattern>/rest/*</url-pattern> <!--请求地址时需要在项目和servicepath之间加上这个rest--> </servlet-mapping> </web-app>
5.测试service接口是否成功
6.确定service接口没问题了,在java中写调用接口的方法,jersey调用接口方法如下
//创建请求对象 ClientConfig config = new DefaultClientConfig(); Client client = Client.create(config); WebResource resource = client.resource(BASE_URI); //具体项目的WebResource //具体路径的WebResource WebResource helloResource = resource.path("rest").path(PATH_Hello + name);
//返回请求结果的xml String responseString = helloResource.accept(MediaType.TEXT_XML).get(String.class);
//返回请求结果的状态 String clientResponseString = helloResource.accept(MediaType.TEXT_XML).get(ClientResponse.class).toString();
7.调用接口
package com.qy; import javax.ws.rs.core.MediaType; import com.sun.jersey.api.client.Client; import com.sun.jersey.api.client.ClientResponse; import com.sun.jersey.api.client.WebResource; import com.sun.jersey.api.client.config.ClientConfig; import com.sun.jersey.api.client.config.DefaultClientConfig; /** * 调用接口 */ public class HelloRestFulClient { public static final String BASE_URI = "http://localhost:8080/RESTful"; public static final String PATH_Hello = "/userService/hello/"; public static final String PATH_Bye = "/userService/bye/"; public static void main(String[] args) { //参数 String name = "qy"; //创建请求对象 ClientConfig config = new DefaultClientConfig(); Client client = Client.create(config); WebResource resource = client.resource(BASE_URI); //具体项目的WebResource //路径 WebResource helloResource = resource.path("rest").path(PATH_Hello + name); System.out.println("Client Response 返回状态"); getClientResponse(helloResource); System.out.println("Response 返回 xml" ); getResponse(helloResource); System.out.println("----------------------------------------------------"); WebResource byeResource = resource.path("rest").path(PATH_Bye + name); System.out.println("Client Response 返回状态"); getClientResponse(byeResource); System.out.println("Response 返回 xml" ); getResponse(byeResource); } /** * 调用WebResource,返回客户端请求状态。 * GET http://localhost:8080/RESTful/rest/userService/hello/qiuyu * 返回请求结果状态“200 OK”。 */ private static String getClientResponse(WebResource resource) { String clientResponseString = resource.accept(MediaType.TEXT_XML) .get(ClientResponse.class).toString(); System.out.println(clientResponseString); return clientResponseString; } /** * 调用WebResource,返回请求结果XML * <root><Say>bye qiuyu</Say></root> */ private static String getResponse(WebResource resource) { String responseString = resource.accept(MediaType.TEXT_XML) .get(String.class); System.out.println(responseString); return responseString; } }
8.执行返回结果
如果这篇文章对你有用,可以关注本人微信公众号获取更多ヽ(^ω^)ノ ~
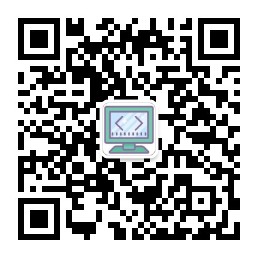