日期:2008年11月25日
30.Rule:
1. 如果A,B都有值,优先取A
2. 如果A有值,B没值,取A
3. 如果A没值,B有值,取B
4. 如果A,B都没值,都不取
5. 如果有值交叉的情况,都不取
A B
|
1 null
|
null 1
|
null 1
|
1 1
|
//创建RoomPriceList副本
HotelRoomPriceEntity[] hotelArr = new HotelRoomPriceEntity[roomPriceList.Count];
roomPriceList.CopyTo( hotelArr );
//数据同步副本
List<HotelRoomPriceEntity> roomPriceListOrigin = new List<HotelRoomPriceEntity>();
foreach ( HotelRoomPriceEntity roomPrice in hotelArr ) {
roomPriceListOrigin.Add( roomPrice );
}
//RoomPriceList ForEach
roomPriceList.ForEach( delegate( HotelRoomPriceEntity roomPrice ) {
//查询与当前RoomID相关的副本中的记录集
List<HotelRoomPriceEntity> roomPriceListInSegment = roomPriceListOrigin.FindAll( delegate( HotelRoomPriceEntity eachRoomInSegment ) {
return roomPrice.Room == eachRoomInSegment.Room;
} );
if ( null == roomPriceListInSegment ) {
return;
}
//根据A和B的规则,计算总值
roomPrice.SumValue = CalculateSumValue( roomPriceListInSegment );
} );
private static Decimal CalculateSumValue ( List<HotelRoomPriceEntity> roomPriceListInSegment ) {
Decimal result = 0.0M;
//在“与当前RoomID相关的副本中的记录集”中查询出A有值的记录集
List<HotelRoomPriceEntity> AList = roomPriceListInSegment.FindAll( delegate( HotelRoomPriceEntity hotelRoom ) {
return hotelRoom.A > 0;
} );
//A都值,取A的总和
if (AList != null && AList.Count == roomPriceListInSegment.Count ) {
AList.ForEach( delegate( HotelRoomPriceEntity room ) {
result += room.A;
} );
}
else {
//B都值,取B的总和,否则其他情况
List<HotelRoomPriceEntity> BList = roomPriceListInSegment.FindAll( delegate( HotelRoomPriceEntity hotelRoom ) {
return hotelRoom.B > 0;
} );
if (BList!= null && BList.Count == roomPriceListInSegment.Count ) {
BList.ForEach( delegate( HotelRoomPriceEntity room ) {
result += room.B;
} );
}
else { //其他情况
result = 0.0M;
}
}
return result;
}
29.DbCommand & In
你在DbCommand中使用select查询时,如果遇到sql语句中用到in时
记住这样用:(拼接Sql方式)
String sqlSearchHotelNoteList = String.Concat( @"SELECT hotel,startdate,enddate,note
FROM OrderDB.dbo.Cii_Hotel_Des WITH(NOLOCK)
WHERE hotel IN (" , queryParameters.HotelIdList , @")
AND startdate <= @checkoutdate
AND enddate >= @checkindate
Order by hotel, startdate" );
不能这样
db.AddInParameter( dbCommand , "@hotelidlist" , DbType.String , queryParameters.HotelIdList );
这样在IDataReader.Reader()时
会报错(Convert Varchar not to Int).
日期:2008年10月20日
28.Sql2005: newid()产生随机数
A B
1 1
1 1
2 2
2 2
3 3
3 3
取1,2,3中的某一条:
Select top 1 from
(select top 1 newid() as new_ID,* from table where A=1
union
select top 1 newid() as new_ID,* from table where A=2
union
select top 1 newid() as new_ID,* from table where A=3 )
order by newid()
日期:2008年7月25日
27.原来还可以这样写!
<asp:TemplateField>
<ItemTemplate>
<asp:HiddenField ID="hdPriceDetail" Value='<%#Eval("Description") %>' runat="server" />
<asp:Label ID="lblDetails" Text='<%#"Details"+Eval("Description") %>' runat="server" />
</ItemTemplate>
</asp:TemplateField>
日期:2008年7月18日
26.如何替换string中的特定字符串或者字符,为另一个字符串或者字符?
Ex: String SearchCropHotelSql = "Select ****************** r.WD_FGprice ,sdafasferaetefadsfasfsdfsdfdsfas#$#$#$@#fas";
ReplaceEx( SearchCropHotelSql , "r.WD_FGprice" , "r.WD_FGprice3" );
效果等同于:SearchCropHotelSql .Replace("r.WD_FGprice" , "r.WD_FGprice3" );
private static String ReplaceEx ( String original , String pattern , String replacement ) {
Int32 count , position0 , position1;
String upperString = original.ToUpper();
String upperPattern = pattern.ToUpper();
Int32 inc = ( original.Length / pattern.Length ) * ( replacement.Length - pattern.Length );
Char[] chars = new Char[original.Length + Math.Max( 0 , inc )];
count = position0 = position1 = 0;
while ( ( position1 = upperString.IndexOf( upperPattern , position0 ) ) != -1 ) {
for ( Int32 i = position0 ; i < position1 ; ++i ) {
chars[count++] = original[i];
}
for ( Int32 i = 0 ; i < replacement.Length ; ++i ) {
chars[count++] = replacement[i];
}
position0 = position1 + pattern.Length;
}
if ( position0 == 0 ) {
return original;
}
for ( Int32 i = position0 ; i < original.Length ; ++i ) {
chars[count++] = original[i];
}
return new String( chars , 0 , count );
}
日期:2008年7月15日
25.在C#中如何遍历Entity或Class中所有属性或者方法
public class test

{
public test()

{
Entity entity = new Entity();
entity.ID = "ID";
entity.Name = "Name";
Type t = entity.GetType();
foreach (PropertyInfo pi in t.GetProperties())

{
Console.WriteLine(pi.GetValue(entity, null));
}
}
}
public class Entity

{
string id;
string name;
public string ID

{

get
{ return id; }

set
{ id = value; }
}
public string Name

{

get
{ return name; }

set
{ name = value; }
}
}

-------------------------------------以前的积累----------------------------------------------------------------------------------
用于在兼容的引用类型之间执行转换。例如:
string s = someObject as string;
as 运算符类似于强制转换,所不同的是,当转换失败时,运算符将产生空,而不是引发异常。更严格地说,这种形式的表达式
expression as type
等效于
expression is type ? (type)expression : (type)null
只是 expression 只被计算一次。
注意,as 运算符只执行引用转换和装箱转换。as 运算符无法执行其他转换,如用户定义的转换,这类转换应使用 cast 表达式来执行。
using System;
class Class1


{
}
class Class2


{
}
class MainClass


{
static void Main()

{
object[] objArray = new object[6];
objArray[0] = new Class1();
objArray[1] = new Class2();
objArray[2] = "hello";
objArray[3] = 123;
objArray[4] = 123.4;
objArray[5] = null;
for (int i = 0; i < objArray.Length; ++i)

{
string s = objArray[i] as string;
Console.Write("{0}:", i);
if (s != null)

{
Console.WriteLine("'" + s + "'");
}
else

{
Console.WriteLine("not a string");
}
}
}
}
输出
0:not a string
1:not a string
2:'hello'
3:not a string
4:not a string
5:not a string

1
1.关于DATASET中rowfilter的使用问题
2
在DATASET中,想要使用rowfilter
3
4
select .OrderID,o.OrderTime,o.OrderAmount,o.OrderNumber,cr.UserName,cr.ContactPhone,cr.DetailAddress from C_CustomerRoute as cr,O_Order as o
5
where cr.CustomerRouteID=o.CustomerRouteID
6
上面的SQL语句用来填充DATASET,但想要在ROWFILTER中实现下面的功能
7
8
and datepart(day,OrderTime)=datepart(day,getdate())
9
10
这句已经在查询分析器里运行过,没有错误。要把这句放到ROWFILTER里怎么写呢?
11
12
比如: GetDataTable() {
13
DataTable dt1 = dt.copy();
14
foreach(DataRow dr in dt.rows)
15
{
16
//取出OrderDate的Day并与当前时间的天做比较。相同就把dr放到dt1中
17
}
18
return dt1;
19
}
20
rowfilter中不能使用别名 rowfilter = "c.useid = 'ww' "[错误的]
21
22
23
24
2.有关于动态加载隐藏域和动态取得隐藏域的值与页面的下拉框的中的值来进行比较。
25
第1种:.在页面中事先写好隐藏域。
26
<form>
27
<asp:HiddenField ID="year" runat="server" />
28
<asp:HiddenField ID="month" runat="server" />
29
</form>
30
然后在CodeFile文件中的page_load中对其进行动态赋值
31
this.year.Value = DateTime.Now.Year.ToString();
32
this.month.Value = DateTime.Now.Month.ToString();
33
最后在到客户端动态取得year,month
34
document.form.year.value
35
document.form.month.value
36
37
第二种:在codefile文件中page_load中进行动态加载隐藏域
38
使用literal控件装载隐藏域
39
this.libAddHidden.Text =" <asp:HiddenField ID=\"year\" value=\"2006\" runat=\"server\" />"+
40
"<asp:HiddenField ID=\"month\" value=\"6\" runat=\"server\" />";
41
这时可以发现在客户端在源码中在page_load中加载的asp:HiddenField 没有转变成 input type="hidden"的形式,
42
这时如果想取得没有转变的隐藏域的值,可以使用
43
document.getElementById("year").value
44
document.getElementById("month").value
45
如果使用以下的取值方式就不对了
46
//document.form.year.value
47
//document.form.month.value
48
反则:如果动态加载到literal控件中的是客户端的input type="hidden"的话,就可以使用任意一种取值方式,即可
49
50
3.JS控制输入
51
<input onkeyup="value=value.replace(/[^\u4E00-\u9FA5]/g,'')" onbeforepaste="clipboardData.setData('text',clipboardData.getData('text').replace(/[^\u4E00-\u9FA5]/g,''))">只能输入汉字
52
<input onkeyup="value=value.replace(/[^\uFF00-\uFFFF]/g,'')" onbeforepaste="clipboardData.setData('text',clipboardData.getData('text').replace(/[^\uFF00-\uFFFF]/g,''))">只能输入全角
53
<input onkeyup="value=value.replace(/[^\d]/g,'') "onbeforepaste="clipboardData.setData('text',clipboardData.getData('text').replace(/[^\d]/g,''))">只能输入数字
54
<input onkeyup="value=value.replace(/[\W]/g,'') "onbeforepaste="clipboardData.setData('text',clipboardData.getData('text').replace(/[^\d]/g,''))">只能输入英文和数字
55
<input onkeyup="value=value.replace(/[^\cd-\cd]/g,'')" onbeforepaste="clipboardData.setData('text',clipboardData.getData('text').replace(/[^\cd-\cd]/g,''))">什么都不能输入
56
<input onkeyup="value=value.replace(/[^\gb-\gb]/g,'')" onbeforepaste="clipboardData.setData('text',clipboardData.getData('text').replace(/[^\gb-\gb]/g,''))">只能输入这几个字母egfdcb
57
58
4.server.Transfer与response.Redirect的作用都是重定向,我认为它与response.Redirect相比有两个优点、一个缺点。
59
60
61
优点:
62
一、它在服务器端直接重定向,不用像response.Redirect一样先与浏览器通信再重定向,效率高。
63
二、它可以传递上一个页面的提交值。比如:A页面提交值到B页面,B页面Transfer到C页面,C页面同样可以收到A页面提交的值。
64
65
66
67
68
缺点:(2006-1-10修改)
69
不能刷新页面,比如:A页面提交登录信息到B页面,B页面处理后Transfer再到A,A页面得不到刷新,指定了过期也不行。如果A、B页面不在同一个目录下,我们还会发现更多意想不到的结果,使用相对链接的图片、超链接的指向都改变了,造成这种原因是B页面读取A页面内容却以B页面的身份输出,所以路径改变了。
70
71
说到server.transfer,就不得不说server.execute,这两个东西有点类似。主要的区别在于,server.execute在第二个页面结束执行后,还会返回第一个页面继续处理.
72
73
同时,使用Server.Transfer时应注意一点:目标页面将使用原始页面创建的应答流(就是跳转本身的页面),这导致ASP.NET的机器验证检查(Machine Authentication Check,MAC)认为新页面的ViewState已被篡改。因此,如果要保留原始页面的表单数据和查询字符串集合,必须把目标页面Page指令的EnableViewStateMac属性设置成False。
74
75
5.C#页面中判断绑定上来的值是否为空
76
<asp:TemplateField>
77
<ItemTemplate>
78
<%# DataBinder.Eval(Container.DataItem, "IDCardTypeName").ToString().Equals("") ?"其他":DataBinder.Eval(Container.DataItem, "IDCardTypeName")%>
79
</ItemTemplate>
80
</asp:TemplateField>
81
<asp:BoundField HeaderText="Type" DataField="IDCardTypeName">
82
<HeaderStyle Font-Bold="False" />
83
</asp:BoundField>
84
85
VB.net
86
<input id="txtPW" onBlur="javascript:formatNumberClient(this);" onFocus="javascript:unformatNumberClient(this,'on');" size=12 value='<%# IIF(isdbnull(ctype(DataBinder.Eval(Container.DataItem,"PostWage"),object)),"0.00",format(cdbl(IIF(isdbnull(DataBinder.Eval(Container.DataItem,"PostWage")),0,DataBinder.Eval(Container.DataItem,"PostWage"))),"##,##0.00")) %>' type="text" MaxLength="9" runat="server" NAME="txtPW" style="text-align:right" >
87
88
89
90
6.引用另一个工程的文件夹里的文件可以用<%=Application["项目工程名Path"]%> /JS/**.js
91
7.判断JS传过来的值,并返回不带空格的完整日期
92
传过来的值有三种形式:
93
1.1900-1-1 00:00:00
94
2.1900-11-1 20:00:00
95
3.1900-12-18 10:00:00以下是判断的代码
96
if(sIdB.substr(8,1) != '' && (sIdB.substr(9,1) == '0' || sIdB.substr(9,1) == '1' || sIdB.substr(9,1) == '2'))
97
{
98
//返回到前一个窗口的值
99
eval("window.opener.document.aspnetForm.birth"+j+".value='"+sIdB.substr(0,8)+"'");
100
}
101
else if(sIdB.substr(9,1)!='' && (sIdB.substr(10,1) == '0' || sIdB.substr(10,1) == '1' || sIdB.substr(10,1) == '2'))
102
{
103
eval("window.opener.document.aspnetForm.birth"+j+".value='"+sIdB.substr(0,9)+"'");
104
}
105
else if(sIdB.substr(10,1)!='' && (sIdB.substr(11,1) == '0' || sIdB.substr(11,1) == '1' || sIdB.substr(11,1) == '2'))
106
{
107
eval("window.opener.document.aspnetForm.birth"+j+".value='"+sIdB.substr(0,10)+"'");
108
}
109
8.RowFilter中不能使用between and 只能用>= and <=代替
110
9.获得任意窗体的实例化句柄
111
_Default wf1 = (_Default)Context.Handler;
112
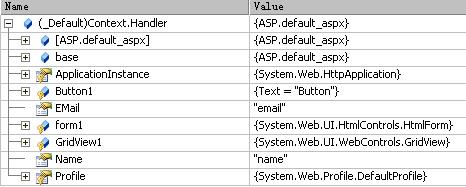
1
10.DataGridView隔行换色的属性
2
获取或设置应用于 DataGridView 的奇数行的默认单元格样式。
3
dataGridView1.AlternatingRowsDefaultCellStyle.BackColor = Color.DarkGray;
4
5
6
11.System.Configuration.ConfigurationErrorsException: The requested database IT-014-211952_FltOrderDB_SELECT is not defined in configuration.
7
8
是由于webconfig 没有正确config <connectionStrings configSource="Config\\DataBaseTest.config" />
9
10
12. IE与FireFox兼容性的问题
11
StringBuilder sb = new StringBuilder();
12
IE
13
14
1
StringBuilder sb = new StringBuilder();
2
sb.Append("<Form id=\"frmCreditCardTemp\" action=\"Default3.aspx\" method=\"post\"><input type=\"hidden\" name=\"orderid\" value=\"" + Request.Form["orderid"].ToString() + "\"></form><script language=\"javascript\">document.getElementById(\"frmCreditCardTemp\").submit();</script>");
3
Response.Write(sb);
FireFox中必须加上<html><body>。。。</body></html>
1
StringBuilder sb = new StringBuilder();
2
sb.Append("<html><body><Form id=\"frmCreditCardTemp\" action=\"Default3.aspx\" method=\"post\"><input type=\"hidden\" name=\"orderid\" value=\"" + Request.Form["orderid"].ToString() + "\"></form><script language=\"javascript\">document.getElementById(\"frmCreditCardTemp\").submit();</script></body></html>");
3
Response.Write(sb);
1
在FireFox中javascript最好包在<body>。。</body>中,不然会有一些问题。
2
3
13.c#里string 与char数组怎么转换
4
string yb = "abc";
5
char[] yy = yb.ToCharArray()
6
7
14.判断是页面上已经动态加载过javascript了
8
if (!Page.IsStartupScriptRegistered("javascriptKey"))
9
{
10
System.Text.StringBuilder vScripts = new System.Text.StringBuilder(2000);
11
vScripts.Append("<script language=\"javascript\">
alert('test')</script>");
12
Page.RegisterStartupScript("javascriptKey", vScripts.ToString());
13
}
14
15
15. 请问如何清除HTML页面文件的缓冲呢?
16
现象是:我在IE地址框中输入一个新的URL地址后,返回的还是当前页面,请问该如何清除客户端的缓存呢??
17
禁用客户端缓存
18
HTM网页
19
<META HTTP-EQUIV="pragma" CONTENT="no-cache">
20
<META HTTP-EQUIV="Cache-Control" CONTENT="no-cache, must-revalidate">
21
<META HTTP-EQUIV="expires" CONTENT="Wed, 26 Feb 1997 08:21:57 GMT">
22
C#中禁止cache的方法!
23
Response.Buffer=true;
24
Response.ExpiresAbsolute=System.DateTime.Now.AddSeconds(-1);
25
Response.Expires=0;
26
Response.CacheControl="no-cache";
27
28
1
16.通过程序把存储过程中的自增长的值输出来
2
C#:
3
Database db = DatabaseFactory.CreateDatabase(connectString);
4
DbCommand dbCommand = db.GetStoredProcCommand("sp1_SaveParamToTempParamForm");
5
6
#region Param
7
8
db.AddOutParameter(dbCommand, "@paramNum", DbType.Int32, 4);
9
//当前用户ID
10
db.AddInParameter(dbCommand, "uid", DbType.String, uid.Trim());
11
12
#endregion
13
14
db.ExecuteNonQuery(dbCommand);
15
16
return (int)db.GetParameterValue(dbCommand, "@paramNum");
17
**********************************************
18
存储过程:
19
CREATE PROCEDURE dbo.sp1_SaveParamToTempParamForm
20
@paramNum int output,
21
@Uid varchar(30),
22
@paramStack varchar(400),
23
@recordTime datetime
24
AS
25
DECLARE @retcode int, @rowcount int
26
Set Lock_Timeout 1000
27
28
Exec @RetCode = sp3_TempParamForm_i
29
@paramNum = @paramNum,
30
@Uid = @Uid,
31
@paramStack = @paramStack,
32
@recordTime = @recordTime
33
34
SELECT @retcode = @@ERROR, @rowcount = @@ROWCOUNT,@paramNum=@@IDENTITY
35
IF @retcode = 0 AND @rowcount = 0
36
RETURN 100
37
ELSE
38
RETURN @retcode
39
GO
40
41
--------------------------------------------
42
CREATE PROCEDURE [dbo].[sp3_TempParamForm_i]
43
@paramNum int output,
44
@Uid varchar(30),
45
@paramStack varchar(400),
46
@recordTime datetime
47
AS
48
49
DECLARE @retcode int, @rowcount int
50
51
SET LOCK_TIMEOUT 1000
52
53
INSERT INTO TempParamForm(Uid,paramStack,recordTime)
54
VALUES(@Uid,@paramStack,@recordTime)
55
56
SELECT @retcode = @@ERROR, @rowcount = @@ROWCOUNT,@paramNum=@@IDENTITY
57
IF @retcode = 0 AND @rowcount = 0
58
RETURN 100
59
ELSE
60
RETURN @retcode
61
GO
62
63
1
通过document.all.tags对HTML元素进行选择,这样就可以通过循环遍历到所有自己想找的元素
2
//檢測選擇多选框的是否指选择一项,并排除全选按钮
3
function chkCheckBoxState()
{
4
var inputs = document.all.tags("input");
5
var j=0;
6
for (var i=0; i < inputs.length; i++)
7
{
8
if (inputs[i].type == "checkbox" && inputs[i].id != "chb_all")
9
{
10
if(inputs[i].checked)
11
{
12
j++;
13
}
14
}
15
}
16
}
17.计算硬盘和文件夹的容量
using System;
using System.IO;
public class ShowDirSize


{
public static long DirSize(DirectoryInfo d)

{
long Size = 0;
// Add file sizes.
FileInfo[] fis = d.GetFiles();
foreach (FileInfo fi in fis)

{
Size += fi.Length;
}
// Add subdirectory sizes.
DirectoryInfo[] dis = d.GetDirectories();
foreach (DirectoryInfo di in dis)

{
Size += DirSize(di);
}
return(Size);
}
public static void Main(string[] args)

{
if (args.Length != 1)

{
Console.WriteLine("You must provide a directory argument at the command line.");
}
else

{
DirectoryInfo d = new DirectoryInfo(args[0]);
Console.WriteLine("The size of {0} and its subdirectories is {1} bytes.", d, DirSize(d));
}
}
}

引用当前工程中另一个项目名:
Application["WebResourcePath"].ToString()
调用网页当前访问的域名:
Request.ServerVariables["server_name"].ToLower()
Http:
Request.ServerVariables["HTTP_REFERER"]
18.在Web.config中创建自定义的Sections,以及如何读取?
网上有许多文章是以这种形式定义Section的,其实是错的
<section name="myDictionary" type="System.Configuration.NameValueSectionHandler,system"/>
正确的为以下
<section name="myDictionary" type="System.Configuration.NameValueSectionHandler"/>
Web.Config中

..
<configSections>
<section name="myDictionary" type="System.Configuration.NameValueSectionHandler"/>
</configSections>
<myDictionary>
<add key="first" value="1"/>
<add key="second" value="2"/>
<add key="third" value="3"/>
</myDictionary>

..
读取用的GetConfig即可:
System.Configuration.ConfigurationManager.GetSection(@"myDictionary");
19.有关于FindControl
FindControl 方法只搜索页的直接或顶级容器;它不在页所包含的命名容器中递归搜索控件。
Page 中FindControl,一般就是this.FindControl,就OK
MasterPage中FindControl
就是这样
ContentPlaceHolder cph;
Label lblLabel;
cph= (ContentPlaceHolder)Master.FindControl("ContentUp");
lblLabel = (Label)cph.FindControl("lblInfo");

20.INamingContainer
看到有下划线分开的ClientID,那就是INamingContainer造成的。
例如用一个Master吧,里面两个Content,它们内部都有一个LinkButton1,那么怎么区分呢?因为Content是INamingContainer,所以就会让两个LinkButton1分别命名为Content1_LinkButton1和Content2_LinkButton2。说得不严谨,不过INamingContainer大概就是这样运作的。
接着,在Master里面找LinkButton1,会找不到。递归查找,没办法保证返回的那个来自于Content1还是Content2(实际上能保证,但理论上不能依赖于这种现实)。
21.Func<T1,T2,Tresult> 2008年4月19日14:45:59
protected List<QueryConditionEntity> FindListByValue(List<QueryConditionEntity> iList, ListItemCollection rbl)

{
return Find(iList, rbl,

() =>
{ return FindListByValue(iList, rbl); });
}
public List<QueryConditionEntity> Find(List<QueryConditionEntity> iList, ListItemCollection rbl, Func<List<QueryConditionEntity>> sourceGetter)

{
Boolean emptyText = false;
for (int i = 0; i < iList.Count; i++)

{
emptyText = rbl.FindByValue(iList[i].OrderID.ToString()) != null;
iList[i].EmployeeID = emptyText ? rbl.FindByValue(iList[i].OrderID.ToString()).Text : String.Empty;
}
return iList;
}
22.给CheckBoxList和RadioButtonList添加滚动条 2008年4月20日10:26:14
23.解决LinkButton不能指定target的问题
lbtn.Attributes.Add("href", "~/hotelSearch.aspx");
lbtn.Attributes.Add("target", "_blank");
24. GridView中的ToolTip 2008年5月13日 23:05:41
看看以下範例是否有用:
protected void GridView1_RowDataBound(object sender, GridViewRowEventArgs e)

{
GridViewRow item = e.Row;
if (e.Row.RowType == DataControlRowType.DataRow)

{
item.Cells[0].Attributes.Add("title",
GridView1.DataKeys[item.RowIndex][0].ToString());
}
}
當滑鼠移到第一個欄位時,顯示的 tooltip 會是第一個欄位的內容。
這跟您想要的有些差距,因為這種方法只能針對特定欄位設定 tooltip,而不是整個 GridView 的 tooltip。若要以此方法達成您的需求,可以在 RowDataBound 事件中把每個欄位的 "title" 屬性設定成特定欄位的內容。這樣應該就很接近您想要的了。

我來提供另一種方法:

Protected Sub GridView1_RowDataBound()Sub GridView1_RowDataBound(ByVal sender As Object, ByVal e As System.Web.UI.WebControls.GridViewRowEventArgs) Handles GridView1.RowDataBound
For index As Integer = 0 To GridView1.Rows.Count - 1
GridView1.Rows(index).Cells(1).ToolTip = "這個是:" & GridView1.Rows(index).Cells(1).Text
Next
End Sub
針對每一個CELL去設定ToolTip。
