1 #include<cstdio> 2 #define MAXN 100010 3 struct node 4 { 5 int left0,right0,v0,left1,right1,v1,lazy; 6 }; 7 node tree[MAXN<<2]; 8 inline int MAX(int x,int y) 9 { 10 return x>y?x:y; 11 } 12 inline int MIN(int x,int y) 13 { 14 return x>y?y:x; 15 } 16 inline void swap(int &x,int &y) 17 { 18 int temp=x; 19 x=y; 20 y=temp; 21 } 22 inline void PushUp(int mid,int L,int R,int rt) 23 { 24 tree[rt].left0=tree[rt<<1].left0; 25 tree[rt].left1=tree[rt<<1].left1; 26 tree[rt].right0=tree[rt<<1|1].right0; 27 tree[rt].right1=tree[rt<<1|1].right1; 28 if(tree[rt].left0==mid-L+1) 29 tree[rt].left0+=tree[rt<<1|1].left0; 30 if(tree[rt].left1==mid-L+1) 31 tree[rt].left1+=tree[rt<<1|1].left1; 32 if(tree[rt].right0==R-mid) 33 tree[rt].right0+=tree[rt<<1].right0; 34 if(tree[rt].right1==R-mid) 35 tree[rt].right1+=tree[rt<<1].right1; 36 tree[rt].v0=MAX(tree[rt<<1].v0,tree[rt<<1|1].v0); 37 tree[rt].v0=MAX(tree[rt].v0,tree[rt<<1].right0+tree[rt<<1|1].left0); 38 tree[rt].v1=MAX(tree[rt<<1].v1,tree[rt<<1|1].v1); 39 tree[rt].v1=MAX(tree[rt].v1,tree[rt<<1].right1+tree[rt<<1|1].left1); 40 } 41 void Build(int L,int R,int rt) 42 { 43 tree[rt].lazy=0; 44 if(L==R) 45 { 46 scanf("%d",&tree[rt].v1); 47 tree[rt].left1=tree[rt].right1=tree[rt].v1; 48 tree[rt].left0=tree[rt].right0=tree[rt].v0=tree[rt].v1^1; 49 } 50 else 51 { 52 int mid=(L+R)>>1; 53 Build(L,mid,rt<<1); 54 Build(mid+1,R,rt<<1|1); 55 PushUp(mid,L,R,rt); 56 } 57 } 58 void Init(int rt) 59 { 60 swap(tree[rt].left0,tree[rt].left1); 61 swap(tree[rt].right0,tree[rt].right1); 62 swap(tree[rt].v0,tree[rt].v1); 63 } 64 inline void PushDown(int mid,int L,int R,int rt) 65 { 66 if(tree[rt].lazy) 67 { 68 tree[rt<<1].lazy^=1; 69 tree[rt<<1|1].lazy^=1; 70 Init(rt<<1); 71 Init(rt<<1|1); 72 tree[rt].lazy=0; 73 } 74 } 75 void Change(int x,int y,int L,int R,int rt) 76 { 77 if(x<=L&&R<=y) 78 { 79 tree[rt].lazy^=1; 80 Init(rt); 81 } 82 else 83 { 84 int mid=(L+R)>>1; 85 PushDown(mid,L,R,rt); 86 if(mid>=x) 87 Change(x,y,L,mid,rt<<1); 88 if(y>mid) 89 Change(x,y,mid+1,R,rt<<1|1); 90 PushUp(mid,L,R,rt); 91 } 92 } 93 int Query(int x,int y,int L,int R,int rt) 94 { 95 if(x<=L&&R<=y) 96 return tree[rt].v1; 97 int mid=(L+R)>>1,ans=0; 98 PushDown(mid,L,R,rt); 99 if(mid>=x) 100 ans=MAX(ans,Query(x,y,L,mid,rt<<1)); 101 if(y>mid) 102 ans=MAX(ans,Query(x,y,mid+1,R,rt<<1|1)); 103 return MAX(ans,MIN(mid-x+1,tree[rt<<1].right1)+MIN(y-mid,tree[rt<<1|1].left1)); 104 } 105 int main() 106 { 107 int n,m,type,x,y; 108 while(~scanf("%d",&n)) 109 { 110 Build(1,n,1); 111 scanf("%d",&m); 112 while(m--) 113 { 114 scanf("%d%d%d",&type,&x,&y); 115 if(type) 116 Change(x,y,1,n,1); 117 else 118 printf("%d\n",Query(x,y,1,n,1)); 119 } 120 } 121 return 0; 122 }
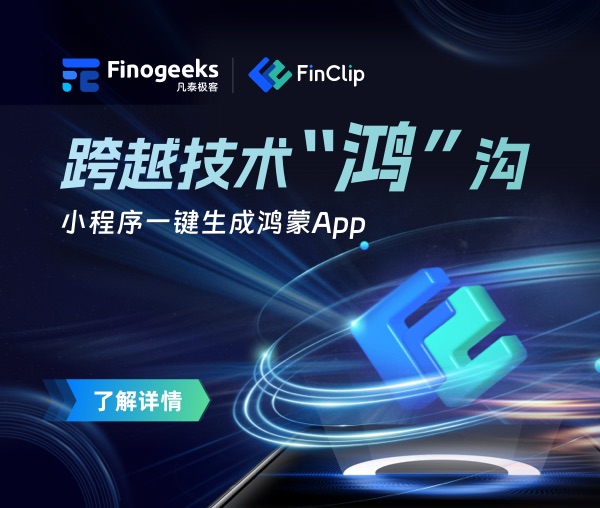