1 #include<cstdio> 2 #include<cstring> 3 #define MAXN 100010 4 int cnt; 5 struct node 6 { 7 int bit,val; 8 }; 9 node tree[MAXN<<2]; 10 inline void swap(int &x,int &y) 11 { 12 int temp=x; 13 x=y; 14 y=temp; 15 } 16 inline void PushUp(int rt) 17 { 18 tree[rt].bit=tree[rt<<1].bit|tree[rt<<1|1].bit; 19 } 20 inline void PushDown(int rt) 21 { 22 if(tree[rt].val) 23 { 24 tree[rt<<1]=tree[rt<<1|1]=tree[rt]; 25 tree[rt].val=0; 26 } 27 } 28 void Update(int x,int y,int val,int L,int R,int rt) 29 { 30 if(x<=L&&R<=y) 31 { 32 tree[rt].val=val; 33 tree[rt].bit=1<<(val-1); 34 } 35 else 36 { 37 int mid=(L+R)>>1; 38 PushDown(rt); 39 if(mid>=x) 40 Update(x,y,val,L,mid,rt<<1); 41 if(y>mid) 42 Update(x,y,val,mid+1,R,rt<<1|1); 43 PushUp(rt); 44 } 45 } 46 void Query(int x,int y,int L,int R,int rt) 47 { 48 if(x<=L&&R<=y) 49 cnt|=tree[rt].bit; 50 else 51 { 52 int mid=(L+R)>>1; 53 PushDown(rt); 54 if(mid>=x) 55 Query(x,y,L,mid,rt<<1); 56 if(y>mid) 57 Query(x,y,mid+1,R,rt<<1|1); 58 } 59 } 60 int main() 61 { 62 char ch; 63 int n,t,q,a,b,c,ans,i; 64 while(~scanf("%d%d%d",&n,&t,&q)) 65 { 66 memset(tree,0,sizeof(tree)); 67 Update(1,n,1,1,n,1); 68 while(q--) 69 { 70 scanf(" %c%d%d",&ch,&a,&b); 71 if(a>b) 72 swap(a,b); 73 if(ch=='C') 74 { 75 scanf("%d",&c); 76 Update(a,b,c,1,n,1); 77 } 78 else 79 { 80 cnt=0; 81 Query(a,b,1,n,1); 82 for(i=ans=0;i<t;i++) 83 { 84 if(cnt&(1<<i)) 85 ans++; 86 } 87 printf("%d\n",ans); 88 } 89 } 90 } 91 return 0; 92 }
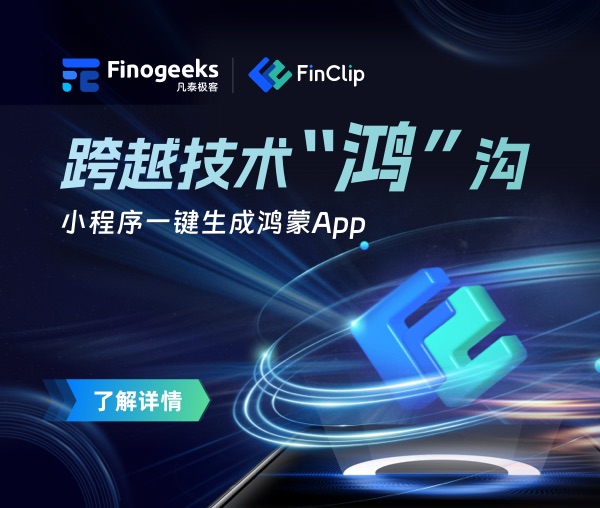