1,工厂方法模式 和简单工厂模式
A :简单工厂模式 :
简单工厂模式属于类的创建型模式,又叫做静态工厂方法模式。通过专门定义一个类来负责创建其他类的实例,被创建的实例通常都具有共同的父类。
主要用于创建对象。新添加类时,不会影响以前的系统代码。核心思想是用一个工厂来根据输入的条件产生不同的类,然后根据不同类的virtual函数得到不同的结果。
GOOD:适用于不同情况创建不同的类时 BUG:客户端必须要知道基类和工厂类,耦合性差
1 #include <iostream> 2 using namespace std; 3 //虚父类 4 class Fruit 5 { 6 public: 7 virtual void getFruit() = 0; 8 9 protected: 10 private: 11 }; 12 //子类A 13 class Banana : public Fruit 14 { 15 public: 16 virtual void getFruit() 17 { 18 cout << "我是香蕉...." << endl; 19 } 20 protected: 21 private: 22 }; 23 //子类B 24 class Apple : public Fruit 25 { 26 public: 27 virtual void getFruit() 28 { 29 cout << "我是苹果...." << endl; 30 } 31 protected: 32 private: 33 }; 34 35 //工厂类 36 class Factory 37 { 38 public: 39 Fruit *create(char *p) 40 { 41 42 if (strcmp(p, "banana") == 0) //若str1==str2,则返回零 43 { 44 return new Banana; 45 } 46 else if (strcmp(p, "apple") == 0) 47 { 48 return new Apple; 49 } 50 else 51 { 52 printf("不支持\n" ) ; 53 return NULL; 54 } 55 } 56 }; 57 58 59 void main() 60 { 61 Factory *f = new Factory; 62 63 Fruit *fruit = NULL; 64 65 66 //工厂生产 香蕉 67 fruit = f->create("banana"); 68 fruit->getFruit(); 69 delete fruit; 70 71 72 fruit = f->create("apple"); 73 fruit->getFruit(); 74 delete fruit; 75 76 delete f; 77 cout<<"hello..."<<endl; 78 system("pause"); 79 return ; 80 }
B :工厂方法模式 把工厂进一步抽象
工厂方法模式同样属于类的创建型模式又被称为多态工厂模式 。工厂方法模式的意义是定义一个创建产品对象的工厂接口,将实际创建工作推迟到子类当中。
核心工厂类不再负责产品的创建,这样核心类成为一个抽象工厂角色,仅负责具体工厂子类必须实现的接口,这样进一步抽象化的好处是使得工厂方法模式可以使系统在不修改具体工厂 角色的情况下引进新的产品。
*工厂方法模式和简单工厂模式比较
工厂方法模式与简单工厂模式在结构上的不同不是很明显。工厂方法类的核心是一个抽象工厂类,而简单工厂模式把核心放在一个具体类上。
工厂方法模式之所以有一个别名叫多态性工厂模式是因为具体工厂类都有共同的接口,或者有共同的抽象父类。
当系统扩展需要添加新的产品对象时,仅仅需要添加一个具体对象以及一个具体工厂对象,原有工厂对象不需要进行任何修改,也不需要修改客户端,很好的符合了“开放-封闭”原则。 而简单工厂模式在添加新产品对象后不得不修改工厂方法,扩展性不好。工厂方法模式退化后可以演变成简单工厂模式。
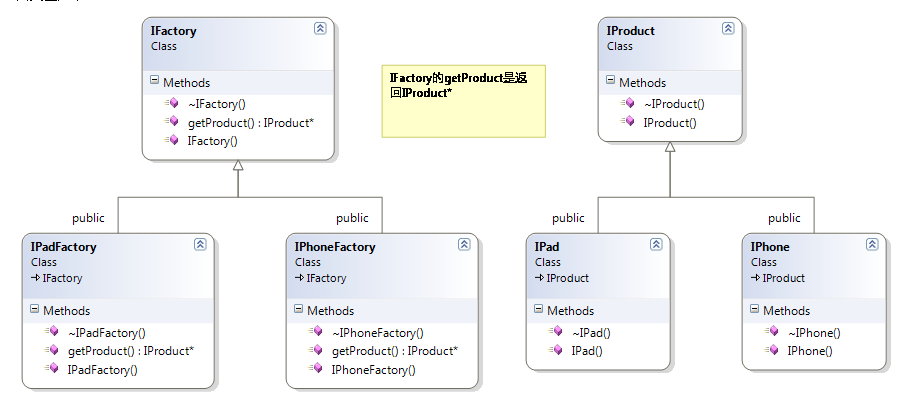
1 #include <iostream> 2 using namespace std; 3 4 //水果虚父类 5 class Fruit 6 { 7 public: 8 virtual void sayname() = 0; 9 }; 10 //水果子类A 11 class Banana : public Fruit 12 { 13 public: 14 void sayname() 15 { 16 cout << "我是香蕉" << endl; 17 } 18 }; 19 //水果子类B 20 class Apple : public Fruit 21 { 22 public: 23 void sayname() 24 { 25 cout << "我是 Apple" << endl; 26 } 27 }; 28 //工厂虚父类 29 class AbFactory 30 { 31 public: 32 virtual Fruit *CreateProduct() = 0; 33 }; 34 //工厂子类A 35 class BananaFactory :public AbFactory 36 { 37 public: 38 virtual Fruit *CreateProduct() 39 { 40 return new Banana; 41 } 42 }; 43 //工厂子类B 44 class AppleFactory :public AbFactory 45 { 46 public: 47 virtual Fruit *CreateProduct() 48 { 49 return new Apple; 50 } 51 }; 52 53 ////////////////////////////////////////////////////////////////////////// 54 //添加新的产品 只需添加 水果子类X 和工厂子类X 55 //水果子类X 56 class Pear : public Fruit 57 { 58 public: 59 virtual void sayname() 60 { 61 cout << "我是 pear" << endl; 62 } 63 protected: 64 private: 65 }; 66 //工厂子类X 67 class PearFactory : public AbFactory 68 { 69 public: 70 virtual Fruit *CreateProduct() 71 { 72 return new Pear; 73 } 74 }; 75 76 77 78 void main() 79 { 80 AbFactory *factory = NULL; 81 Fruit *fruit = NULL; 82 83 //吃 香蕉 84 factory = new BananaFactory; 85 fruit = factory->CreateProduct(); 86 fruit->sayname(); 87 88 delete fruit; 89 delete factory; 90 91 92 //Pear 93 factory = new PearFactory; 94 fruit = factory->CreateProduct(); 95 fruit->sayname(); 96 97 delete fruit; 98 delete factory; 99 100 101 cout<<"hello..."<<endl; 102 system("pause"); 103 return ; 104 }